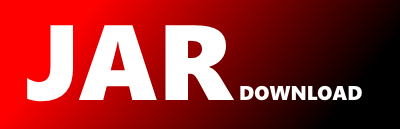
org.openas2.cmd.CommandResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openas2-server Show documentation
Show all versions of openas2-server Show documentation
Open source implementation of the AS2 standard for signed encrypted and compressed document transfer
package org.openas2.cmd;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
public class CommandResult {
public static final String TYPE_OK = "OK";
public static final String TYPE_ERROR = "ERROR";
public static final String TYPE_WARNING = "WARNING";
public static final String TYPE_INVALID_PARAM_COUNT = "INVALID PARAMETER COUNT";
public static final String TYPE_COMMAND_NOT_SUPPORTED = "COMMAND NOT SUPPORTED";
public static final String TYPE_EXCEPTION = "EXCEPTION";
private String type;
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy