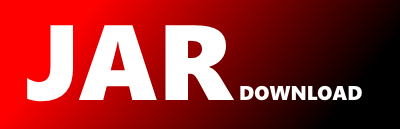
org.openas2.processor.storage.BaseStorageModule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openas2-server Show documentation
Show all versions of openas2-server Show documentation
Open source implementation of the AS2 standard for signed encrypted and compressed document transfer
package org.openas2.processor.storage;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.Map;
import org.apache.commons.io.IOUtils;
import org.openas2.OpenAS2Exception;
import org.openas2.Session;
import org.openas2.message.Message;
import org.openas2.params.InvalidParameterException;
import org.openas2.processor.BaseProcessorModule;
import org.openas2.util.IOUtil;
public abstract class BaseStorageModule extends BaseProcessorModule implements StorageModule {
public static final String PARAM_FILENAME = "filename";
public static final String PARAM_PROTOCOL = "protocol";
public static final String PARAM_TEMPDIR = "tempdir";
public boolean canHandle(String action, Message msg, Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy