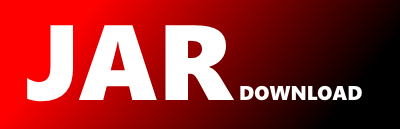
org.openas2.util.RawDataManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openas2-server Show documentation
Show all versions of openas2-server Show documentation
Open source implementation of the AS2 standard for signed encrypted and compressed document transfer
/**
*
*/
package org.openas2.util;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.CommandLineParser;
import org.apache.commons.cli.DefaultParser;
import org.apache.commons.cli.HelpFormatter;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.Options;
import org.apache.commons.cli.ParseException;
/**
* @author chris
*
*/
public class RawDataManager {
public static final String FILE_OPT = "f";
public static final String BASEDIR_OPT = "b";
public static final String MDNFILE_OPT = "m";
public static final String HELP_OPT = "h";
/*
* Options in this format: short-opt, long-opt, has-argument, required, description
*/
public String [][] opts = {
{ FILE_OPT, "file", "true", "true", "the raw file to load" }
,{ MDNFILE_OPT, "mdn-file", "true", "flase", "verify this raw MDN response file associated with the file" }
,{ BASEDIR_OPT, "basedir", "true", "false", "base directory for source files" }
,{ HELP_OPT, "help", "false", "false", "print this help" }
};
private void usage(Options options) {
String header = "Manages raw data files created by the audit mechanism in OpenAS2."
+ "\nCan be used to print the raw data in human readable format or verify message files against MDN response.\n\n"
+ "\nIf basedir is specified then all source files will be prefixed with this";
String footer = "\nPlease report issues or enhancement suggestions here: https://sourceforge.net/p/openas2/discussion/";
HelpFormatter formatter = new HelpFormatter();
formatter.printHelp(this.getClass().getName(), header, options, footer, true);
}
private CommandLine parseCommandLine(String[] args) {
// create the command line parser
CommandLineParser parser = new DefaultParser();
// create the Options
Options options = new Options();
for (String[] opt : opts) {
Option option = Option.builder(opt[0]).longOpt(opt[1]).hasArg("true".equalsIgnoreCase(opt[2])).desc(opt[4]).build();
option.setRequired("true".equalsIgnoreCase(opt[3]));
options.addOption(option);
}
// parse the command line arguments
CommandLine line = null;
try {
line = parser.parse(options, args);
} catch (ParseException e) {
System.out.println("Unexpected exception:" + e.getMessage());
usage(options);
}
return line;
}
private void process(String[] args) {
CommandLine line = parseCommandLine(args);
String filename = line.getOptionValue(FILE_OPT);
if (line.hasOption(BASEDIR_OPT)) {
filename = line.getOptionValue(BASEDIR_OPT) + File.separator + filename;
}
if (line.hasOption(MDNFILE_OPT)) {
// Verify against an MDN
}
else
printFile(filename);
}
private void printFile(String filename) {
FileInputStream fis = null;
try {
fis = new FileInputStream(filename);
} catch (FileNotFoundException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
finally {
if (fis != null)
try {
fis.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
/**
* @param args
*/
public static void main(String[] args) {
try {
RawDataManager mgr = new RawDataManager();
mgr.process(args);
} catch (Exception e) {
System.out.println("Unexpected exception:" + e.getMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy