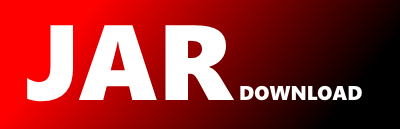
net.sf.opendse.optimization.SATCreatorDecoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of opendse-optimization Show documentation
Show all versions of opendse-optimization Show documentation
The optimization module of OpenDSE
/*******************************************************************************
* Copyright (c) 2015 OpenDSE
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*******************************************************************************/
package net.sf.opendse.optimization;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import net.sf.opendse.model.Link;
import net.sf.opendse.model.Mapping;
import net.sf.opendse.model.Resource;
import net.sf.opendse.model.Specification;
import net.sf.opendse.optimization.encoding.Interpreter;
import net.sf.opendse.optimization.encoding.variables.CR;
import net.sf.opendse.optimization.encoding.variables.EAVI;
import org.opt4j.core.Genotype;
import org.opt4j.core.common.random.Rand;
import org.opt4j.core.optimizer.Control;
import org.opt4j.core.start.Constant;
import org.opt4j.satdecoding.AbstractSATDecoder;
import org.opt4j.satdecoding.Constraint;
import org.opt4j.satdecoding.Model;
import org.opt4j.satdecoding.SATManager;
import com.google.inject.Inject;
import com.google.inject.Singleton;
@Singleton
public class SATCreatorDecoder extends AbstractSATDecoder {
List> order = new ArrayList>();
Map, Double> lb = new HashMap, Double>();
Map, Double> ub = new HashMap, Double>();
int indexOf(Object object) {
for (int i = 0; i < order.size(); i++) {
if (order.get(i).isAssignableFrom(object.getClass())) {
return i;
}
}
return -1;
}
protected final SATConstraints constraints;
protected final SpecificationWrapper specificationWrapper;
protected final Interpreter interpreter;
protected final Control control;
protected final boolean useVariableOrder;
@Inject
public SATCreatorDecoder(SATManager manager, Rand random, SATConstraints constraints, SpecificationWrapper specificationWrapper,
Interpreter interpreter, Control control,
@Constant(value = "variableorder", namespace = SATCreatorDecoder.class) boolean useVariableOrder) {
super(manager, random);
this.constraints = constraints;
this.specificationWrapper = specificationWrapper;
this.interpreter = interpreter;
this.control = control;
this.useVariableOrder = useVariableOrder;
if (useVariableOrder) {
order.add(Resource.class);
order.add(Link.class);
order.add(EAVI.class);
order.add(Mapping.class);
order.add(CR.class);
} else {
order.add(Object.class);
}
}
@Override
public ImplementationWrapper convertModel(Model model) {
if (model == null) {
control.doTerminate();
System.err.println("No feasible implementation exists.");
return new ImplementationWrapper(null);
}
model = constraints.decorate(model);
Specification specification = specificationWrapper.getSpecification();
Specification implementation = interpreter.toImplementation(specification, model);
ImplementationWrapper wrapper = new ImplementationWrapper(implementation);
return wrapper;
}
@Override
public Set createConstraints() {
Set constraints = new HashSet(this.constraints.getConstraints());
return constraints;
}
@Override
public void randomize(Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy