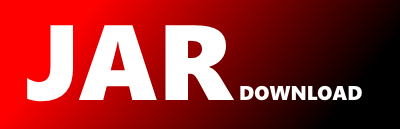
aSaxon-B-9-0-0-8sources.api.Saxon.Api.Destination.cs Maven / Gradle / Ivy
The newest version!
using System;
using System.IO;
using System.Xml;
using System.Collections;
using JConfiguration = net.sf.saxon.Configuration;
using JReceiver = [email protected];
using JProperties = java.util.Properties;
using JOutputStream = java.io.OutputStream;
using JWriter = java.io.Writer;
using JFileOutputStream = java.io.FileOutputStream;
using JXPathException = net.sf.saxon.trans.XPathException;
using JResult = javax.xml.transform.Result;
using JStreamResult = javax.xml.transform.stream.StreamResult;
using JTinyBuilder = net.sf.saxon.tinytree.TinyBuilder;
using net.sf.saxon.@event;
using net.sf.saxon.om;
using net.sf.saxon.value;
using net.sf.saxon.query;
using net.sf.saxon.dotnet;
namespace Saxon.Api {
///
/// An abstract destination for the results of a query or transformation
///
///
/// Note to implementors: To implement a new kind of destination, you need
/// to supply a method getResult which returns an implementation of
/// the JAXP Result interface. Optionally, if the destination
/// performs serialization, you can also implement getOutputProperties ,
/// which returns the properties used for serialization.
///
///
public abstract class XmlDestination {
///
/// Get a Result to which the XML document can be sent as a series
/// of events.
///
///
/// This must be an implementation of the JAXP Result interface that is
/// recognized by Saxon.
///
public abstract JResult GetResult();
///
/// Get a set of Properties representing the parameters to the serializer.
/// The default implementation returns an empty set of properties.
///
public virtual JProperties GetOutputProperties() {
return new JProperties();
}
///
/// Close the Destination, releasing any resources that need to be released.
///
///
/// This method is called by the system on completion of a query or transformation.
/// Some kinds of Destination may need to close an output stream, others might
/// not need to do anything. The default implementation does nothing.
///
public virtual void Close() {
}
}
///
/// A Serializer takes a tree representation of XML and turns
/// it into lexical XML markup.
///
///
/// Note that this is serialization in the sense of the W3C XSLT and XQuery specifications.
/// Unlike the class System.Xml.Serialization.XmlSerializer , this object does not
/// serialize arbitrary CLI objects.
///
public class Serializer : XmlDestination {
private JProperties props = new JProperties();
private JOutputStream outputStream = null;
private JWriter writer = null;
private bool mustClose = true;
/// QName identifying the serialization parameter "method". If the method
/// is a user-defined method, then it is given as a QName in Clark notation, that is
/// "{uri}local".
public static readonly QName METHOD =
new QName("", "method");
/// QName identifying the serialization parameter "byte-order-mark"
public static readonly QName BYTE_ORDER_MARK =
new QName("", "byte-order-mark");
/// QName identifying the serialization parameter "cdata-section-elements".
/// The value of this parameter is given as a space-separated list of expanded QNames in
/// Clark notation, that is "{uri}local".
public static readonly QName CDATA_SECTION_ELEMENTS =
new QName("", "cdata-section-elements");
/// QName identifying the serialization parameter "doctype-public"
public static readonly QName DOCTYPE_PUBLIC =
new QName("", "doctype-public");
/// QName identifying the serialization parameter "doctype-system"
public static readonly QName DOCTYPE_SYSTEM =
new QName("", "doctype-system");
/// QName identifying the serialization parameter "encoding"
public static readonly QName ENCODING =
new QName("", "encoding");
/// QName identifying the serialization parameter "escape-uri-attributes".
/// The value is the string "yes" or "no".
public static readonly QName ESCAPE_URI_ATTRIBUTES =
new QName("", "escape-uri-attributes");
/// QName identifying the serialization parameter "include-content-type".
/// The value is the string "yes" or "no".
public static readonly QName INCLUDE_CONTENT_TYPE =
new QName("", "include-content-type");
/// QName identifying the serialization parameter "indent".
/// The value is the string "yes" or "no".
public static readonly QName INDENT =
new QName("", "indent");
/// QName identifying the serialization parameter "media-type".
public static readonly QName MEDIA_TYPE =
new QName("", "media-type");
/// QName identifying the serialization parameter "normalization-form"
public static readonly QName NORMALIZATION_FORM =
new QName("", "normalization-form");
/// QName identifying the serialization parameter "omit-xml-declaration".
/// The value is the string "yes" or "no".
public static readonly QName OMIT_XML_DECLARATION =
new QName("", "omit-xml-declaration");
/// QName identifying the serialization parameter "standalone".
/// The value is the string "yes" or "no" or "omit".
public static readonly QName STANDALONE =
new QName("", "standalone");
/// QName identifying the serialization parameter "undeclare-prefixes".
/// The value is the string "yes" or "no".
public static readonly QName UNDECLARE_PREFIXES =
new QName("", "undeclare-prefixes");
/// QName identifying the serialization parameter "use-character-maps".
/// This is available only with XSLT. The value of the parameter is a list of expanded QNames
/// in Clark notation giving the names of character maps defined in the XSLT stylesheet.
public static readonly QName USE_CHARACTER_MAPS =
new QName("", "use-character-maps");
/// QName identifying the serialization parameter "version"
public static readonly QName VERSION =
new QName("", "version");
private const String SAXON = NamespaceConstant.SAXON;
/// QName identifying the serialization parameter "saxon:character-representation"
public static readonly QName SAXON_CHARACTER_REPRESENTATION =
new QName(SAXON, "saxon:character-representation");
/// QName identifying the serialization parameter "saxon:indent-spaces". The value
/// is an integer (represented as a string) indicating the amount of indentation required.
/// If specified, this parameter overrides indent="no".
public static readonly QName SAXON_INDENT_SPACES =
new QName(SAXON, "saxon:indent-spaces");
/// QName identifying the serialization parameter "saxon:next-in-chain". This
/// is available only with XSLT, and identifies the URI of a stylesheet that is to be used to
/// process the results before passing them to their final destination.
public static readonly QName NEXT_IN_CHAIN =
new QName(SAXON, "saxon:next-in-chain");
/// QName identifying the serialization parameter "saxon:require-well-formed". The
/// value is the string "yes" or "no". If set to "yes", the output must be a well-formed
/// document, or an error will be reported. ("Well-formed" here means that the document node
/// must have exactly one element child, and no text node children other than whitespace-only
/// text nodes).
public static readonly QName SAXON_REQUIRE_WELL_FORMED =
new QName(SAXON, "saxon:require-well-formed");
/// Create a Serializer
public Serializer() {
}
/// Set a serialization property
/// In the case of XSLT, properties set within the serializer override
/// any properties set in xsl:output declarations in the stylesheet.
/// Similarly, with XQuery, they override any properties set in the Query
/// prolog using declare option saxon:output .
///
///
/// Serializer qout = new Serializer();
/// qout.SetOutputProperty(Serializer.METHOD, "xml");
/// qout.SetOutputProperty(Serializer.INDENT, "yes");
/// qout.SetOutputProperty(Serializer.SAXON_INDENT_SPACES, "1");
///
///
/// The name of the serialization property to be set
/// The value to be set for the serialization property. May be null
/// to unset the property (that is, to set it back to the default value).
public void SetOutputProperty(QName name, String value) {
props.setProperty(name.ClarkName, value);
}
/// Specify the destination of the serialized output, in the
/// form of a file name
/// The name of the file to receive the serialized output
/// Throws a DynamicError if it is not possible to create an output
/// stream to write to this file, for example, if the filename is in a directory
/// that does not exist.
public void SetOutputFile(String filename) {
try {
outputStream = new JFileOutputStream(filename);
mustClose = true;
} catch (java.io.IOException err) {
JXPathException e = new JXPathException(err);
throw new DynamicError(e);
}
}
/// Specify the destination of the serialized output, in the
/// form of a Stream
/// Saxon will not close the stream on completion; this is the
/// caller's responsibility.
/// The stream to which the output will be written.
/// This must be a stream that allows writing.
public void SetOutputStream(Stream stream) {
outputStream = new DotNetOutputStream(stream);
mustClose = false;
}
/// Specify the destination of the serialized output, in the
/// form of a TextWriter
/// Note that when writing to a TextWriter , character encoding is
/// the responsibility of the TextWriter , not the Serializer . This
/// means that the encoding requested in the output properties is ignored; it also
/// means that characters that cannot be represented in the target encoding will
/// use whatever fallback representation the TextWriter defines, rather than
/// being represented as XML character references.
/// The stream to which the output will be written.
/// This must be a stream that allows writing. Saxon will not close the
/// textWriter on completion; this is the caller's responsibility.
public void SetOutputWriter(TextWriter textWriter) {
writer = new DotNetWriter(textWriter);
mustClose = false;
}
internal JReceiver GetReceiver(JConfiguration config) {
return config.getSerializerFactory().getReceiver(
GetResult(),
config.makePipelineConfiguration(),
GetOutputProperties());
}
///
public override JResult GetResult() {
if (outputStream != null) {
return new JStreamResult(outputStream);
} else if (writer != null) {
return new JStreamResult(writer);
} else {
return new JStreamResult(new DotNetWriter(Console.Out));
}
}
///
public override JProperties GetOutputProperties() {
return props;
}
///
public override void Close() {
if (mustClose) {
if (outputStream != null) {
outputStream.close();
}
if (writer != null) {
writer.close();
}
}
}
}
///
/// A DomDestination represents an XmlDocument that is constructed to hold the
/// output of a query or transformation.
///
///
/// No data needs to be supplied to the DomDestination object. The query or transformation
/// populates an XmlDocument , which may then be retrieved as the value of the XmlDocument
/// property
///
public class DomDestination : XmlDestination {
internal DotNetDomBuilder builder;
/// Construct a DomDestination
public DomDestination() {
builder = new DotNetDomBuilder();
}
/// After construction, retrieve the constructed document node
public XmlDocument XmlDocument {
get { return builder.getDocumentNode(); }
}
///
public override JResult GetResult() {
return builder;
}
}
///
/// A TextWriterDestination is an implementation of XmlDestination that wraps
/// an instance of XmlWriter .
///
///
/// The name TextWriterDestination is a misnomer; originally this class would
/// only wrap an XmlTextWriter . It will now wrap any XmlWriter .
/// Note that when a TextWriterDestination is used to process the output of a stylesheet
/// or query, the output format depends only on the way the underlying XmlTextWriter
/// is configured; serialization parameters present in the stylesheet or query are ignored.
///
public class TextWriterDestination : XmlDestination {
internal XmlWriter writer;
/// Construct a TextWriterDestination
/// The XmlTextWriter that is to be notified of the events
/// representing the XML document.
public TextWriterDestination(XmlWriter writer) {
this.writer = writer;
}
///
public override JResult GetResult() {
DotNetReceiver dnr = new DotNetReceiver(writer);
return dnr;
//[email protected] filter = new [email protected]();
//filter.setUnderlyingReceiver(dnr);
//return filter;
}
}
///
/// An XdmDestination is an XmlDestination in which an XdmNode
/// is constructed to hold the output of a query or transformation:
/// that is, a tree using Saxon's implementation of the XDM data model
///
///
/// No data needs to be supplied to the XdmDestination object. The query or transformation
/// populates an XmlNode , which may then be retrieved as the value of the XmlNode
/// property.
/// An XdmDestination can be reused to hold the results of a second transformation only
/// if the reset method is first called to reset its state.
///
public class XdmDestination : XmlDestination {
internal JTinyBuilder builder;
/// Construct an XdmDestination
public XdmDestination() {
builder = new JTinyBuilder();
}
/// Reset the state of the XdmDestination so that it can be used to hold
/// the result of another transformation.
public void Reset() {
builder = new JTinyBuilder();
}
/// After construction, retrieve the constructed document node
public XdmNode XdmNode {
get {
return (XdmNode)XdmValue.Wrap(builder.getCurrentRoot());
}
}
///
public override JResult GetResult() {
return builder;
}
}
}
//
// The contents of this file are subject to the Mozilla Public License Version 1.0 (the "License");
// you may not use this file except in compliance with the License. You may obtain a copy of the
// License at http://www.mozilla.org/MPL/
//
// Software distributed under the License is distributed on an "AS IS" basis,
// WITHOUT WARRANTY OF ANY KIND, either express or implied.
// See the License for the specific language governing rights and limitations under the License.
//
// The Original Code is: all this file.
//
// The Initial Developer of the Original Code is Michael H. Kay.
//
// Portions created by (your name) are Copyright (C) (your legal entity). All Rights Reserved.
//
// Contributor(s): none.
//
© 2015 - 2025 Weber Informatics LLC | Privacy Policy