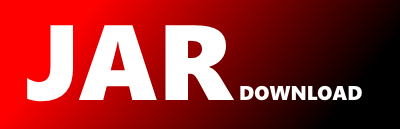
aSaxon-B-9-0-0-8sources.api.Saxon.Api.Errors.cs Maven / Gradle / Ivy
using System;
using System.Collections;
using javax.xml.transform;
using javax.xml.transform.stream;
using JStaticError = net.sf.saxon.trans.StaticError;
using JDynamicError = net.sf.saxon.trans.DynamicError;
using XPathException = net.sf.saxon.trans.XPathException;
namespace Saxon.Api {
///
/// The StaticError class contains information about a static error detected during
/// compilation of a stylesheet, query, or XPath expression.
///
[Serializable]
public class StaticError : Exception {
private XPathException exception;
internal bool isWarning;
///
/// Create a new StaticError, wrapping a Saxon XPathException
///
internal StaticError(TransformerException err) {
if (err is XPathException) {
this.exception = (XPathException)err;
} else {
this.exception = XPathException.makeXPathException(err);
}
}
///
/// The error code, as a QName. May be null if no error code has been assigned
///
public QName ErrorCode {
get {
return new QName("err",
exception.getErrorCodeNamespace(),
exception.getErrorCodeLocalPart());
}
}
///
/// Return the message associated with this error
///
public override String Message {
get {
return exception.getMessage();
}
}
///
/// The URI of the query or stylesheet module in which the error was detected
/// (as a string)
///
///
/// May be null if the location of the error is unknown, or if the error is not
/// localized to a specific module, or if the module in question has no known URI
/// (for example, if it was supplied as an anonymous Stream)
///
public String ModuleUri {
get {
if (exception.getLocator() == null) {
return null;
}
return exception.getLocator().getSystemId();
}
}
///
/// The line number locating the error within a query or stylesheet module
///
///
/// May be set to -1 if the location of the error is unknown
///
public int LineNumber {
get {
SourceLocator loc = exception.getLocator();
if (loc == null) {
if (exception.getException() is TransformerException) {
loc = ((TransformerException)exception.getException()).getLocator();
if (loc != null) {
return loc.getLineNumber();
}
}
return -1;
}
return loc.getLineNumber();
}
}
///
/// Indicate whether this error is being reported as a warning condition. If so, applications
/// may ignore the condition, though the results may not be as intended.
///
public bool IsWarning {
get {
return isWarning;
}
set {
isWarning = value;
}
}
///
/// Indicate whether this condition is a type error.
///
public bool IsTypeError {
get {
return exception.isTypeError();
}
}
///
/// Return the error message.
///
public override String ToString() {
return exception.getMessage();
}
}
///
/// The DynamicError class contains information about a dynamic error detected during
/// execution of a stylesheet, query, or XPath expression.
///
[Serializable]
public class DynamicError : Exception {
private XPathException exception;
internal bool isWarning;
///
/// Create a new DynamicError, specifying the error message
///
public DynamicError(String message) {
exception = new XPathException(message);
}
///
/// Create a new DynamicError, wrapping a Saxon XPathException
///
internal DynamicError(TransformerException err) {
if (err is XPathException) {
this.exception = (XPathException)err;
} else {
this.exception = XPathException.makeXPathException(err);
}
}
///
/// The error code, as a QName. May be null if no error code has been assigned
///
public QName ErrorCode {
get {
return new QName("err",
exception.getErrorCodeNamespace(),
exception.getErrorCodeLocalPart());
}
}
///
/// Return the message associated with this error
///
public override String Message {
get {
return exception.getMessage();
}
}
///
/// The URI of the query or stylesheet module in which the error was detected
/// (as a string)
///
///
/// May be null if the location of the error is unknown, or if the error is not
/// localized to a specific module, or if the module in question has no known URI
/// (for example, if it was supplied as an anonymous Stream)
///
public String ModuleUri {
get {
if (exception.getLocator() == null) {
return null;
}
return exception.getLocator().getSystemId();
}
}
///
/// The line number locating the error within a query or stylesheet module
///
///
/// May be set to -1 if the location of the error is unknown
///
public int LineNumber {
get {
SourceLocator loc = exception.getLocator();
if (loc == null) {
if (exception.getException() is TransformerException) {
loc = ((TransformerException)exception.getException()).getLocator();
if (loc != null) {
return loc.getLineNumber();
}
}
return -1;
}
return loc.getLineNumber();
}
}
///
/// Indicate whether this error is being reported as a warning condition. If so, applications
/// may ignore the condition, though the results may not be as intended.
///
public bool IsWarning {
get {
return isWarning;
}
set {
isWarning = value;
}
}
///
/// Indicate whether this condition is a type error.
///
public bool IsTypeError {
get {
return exception.isTypeError();
}
}
///
/// Return the error message.
///
public override String ToString() {
return exception.getMessage();
}
}
[Serializable]
internal class ErrorGatherer : javax.xml.transform.ErrorListener {
private IList errorList;
public ErrorGatherer(IList errorList) {
this.errorList = errorList;
}
public void warning(TransformerException exception) {
StaticError se = new StaticError(exception);
se.isWarning = true;
//Console.WriteLine("(Adding warning " + exception.getMessage() + ")");
errorList.Add(se);
}
public void error(TransformerException error) {
StaticError se = new StaticError(error);
se.isWarning = false;
//Console.WriteLine("(Adding error " + error.getMessage() + ")");
errorList.Add(se);
}
public void fatalError(TransformerException error) {
StaticError se = new StaticError(error);
se.isWarning = false;
errorList.Add(se);
//Console.WriteLine("(Adding fatal error " + error.getMessage() + ")");
throw error;
}
}
}
//
// The contents of this file are subject to the Mozilla Public License Version 1.0 (the "License");
// you may not use this file except in compliance with the License. You may obtain a copy of the
// License at http://www.mozilla.org/MPL/
//
// Software distributed under the License is distributed on an "AS IS" basis,
// WITHOUT WARRANTY OF ANY KIND, either express or implied.
// See the License for the specific language governing rights and limitations under the License.
//
// The Original Code is: all this file.
//
// The Initial Developer of the Original Code is Michael H. Kay.
//
// Portions created by (your name) are Copyright (C) (your legal entity). All Rights Reserved.
//
// Contributor(s): none.
//
© 2015 - 2025 Weber Informatics LLC | Privacy Policy