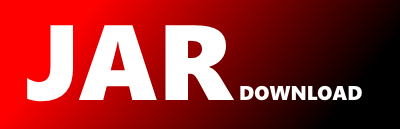
javax.xml.xquery.XQQueryException Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Saxon-HE Show documentation
Show all versions of Saxon-HE Show documentation
The XSLT and XQuery Processor
/*
* Copyright 2003, 2004, 2005, 2006, 2007, 2008 Oracle. All rights reserved.
*/
package javax.xml.xquery;
import javax.xml.namespace.QName;
import java.lang.String;
/**
* An exception that provides information on errors occurring during the
* evaluation of an xquery.
*
*
* Each XQQueryException
provides several kinds of optional
* information, in addition to the properties inherited from
* XQException
:
*
* - an error code. This
QName
identifies the error
* according to the standard as described in
* Appendix F, XQuery 1.0:
* An XML Query language,
* Appendix C, XQuery 1.0 and XPath 2.0 Functions and Operators, and
* and its associated specifications; implementation-defined errors may be raised.
* - a position. This identifies the character position of the failing expression in
* the query text. This is a
0
based position. -1
if unknown.
* - the line number in the query string where the error occured. Line numbering
* starts at
1
. -1
if unknown
* - the column number in the query string where the error occured. Column numbering
* starts at
1
. -1
if unknown
* - the module uri. This identifies the module in which the error
* occurred,
null
when the error is located in the main module.
* - the XQuery error object of this exception. This is the
*
$error-object
argument specified through the fn:error()
* function. May be null
if not specified.
* - the XQuery stack trace. This provides additional dynamic
* information where the exception occurred in the XQuery expression.
*/
public class XQQueryException extends XQException {
private QName errorCode;
private XQSequence errorObject;
private XQStackTraceElement[] stackTrace;
private int line = -1;
private int column = -1;
private int position = -1;
private String moduleURI;
/**
* Constructs an XQQueryException
object with a given message.
*
* @param message the description of the error. null
indicates
* that the message string is non existant
*/
public XQQueryException(String message) {
super(message);
}
/**
* Constructs an XQQueryException
object with a given message,
* and error code.
*
* @param message the description of the error. null
indicates
* that the message string is non existant
* @param errorCode QName
which identifies the error
* according to the standard as described in
*
* Appendix F, XQuery 1.0: An XML Query language,
*
* Appendix C, XQuery 1.0 and XPath 2.0 Functions and Operators,
* and its associated specifications; implementation-defined
* errors may be raised.
*/
public XQQueryException(String message, QName errorCode) {
super(message);
this.errorCode = errorCode;
}
/**
* Constructs an XQQueryException
object with a given message,
* error code, line number, column number, and position.
*
* @param message the description of the error. null
indicates
* that the message string is non existant
* @param errorCode QName
which identifies the error
* according to the standard as described in
*
* Appendix F, XQuery 1.0: An XML Query language,
*
* Appendix C, XQuery 1.0 and XPath 2.0 Functions and Operators,
* and its associated specifications; implementation-defined
* errors may be raised
* @param line the line number in the query string where the error occured.
* Line numbering starts at 1
. -1
if unknown
* @param column the column number in the query string where the error occured.
* Column numbering starts at 1
. -1
if unknown
* @param position the position in the query string where the error occured. This
* is a 0
based position. -1
if unknown
*/
public XQQueryException(String message, QName errorCode,
int line, int column, int position) {
super(message);
this.errorCode = errorCode;
this.line = line;
this.column = column;
this.position = position;
}
/**
* Constructs an XQQueryException
object with a given message,
* vendor code, error code, line number, column number, and position.
*
* @param message the description of the error. null
indicates
* that the message string is non existant
* @param vendorCode a vendor-specific string identifying the error.
* null
indicates there is no vendor
* code or it is unknown
* @param errorCode QName
which identifies the error
* according to the standard as described in
*
* Appendix F, XQuery 1.0: An XML Query language,
*
* Appendix C, XQuery 1.0 and XPath 2.0 Functions and Operators,
* and its associated specifications; implementation-defined
* errors may be raised
* @param line the line number in the query string where the error occured.
* Line numbering starts at 1
. -1
if unknown
* @param column the column number in the query string where the error occured.
* Column numbering starts at 1
. -1
if unknown
* @param position the position in the query string where the error occured. This
* is a 0
based position. -1
if unknown
*/
public XQQueryException(String message, String vendorCode, QName errorCode,
int line, int column, int position) {
super(message, vendorCode);
this.errorCode = errorCode;
this.line = line;
this.column = column;
this.position = position;
}
/**
* Constructs an XQQueryException
object with a given message,
* vendor code, error code, line number, column number, position, module URI,
* error object, and stack trace.
*
* @param message the description of the error. null
indicates
* that the message string is non existant
* @param vendorCode a vendor-specific string identifying the error.
* null
indicates there is no vendor
* code or it is unknown
* @param errorCode QName
which identifies the error
* according to the standard as described in
*
* Appendix F, XQuery 1.0: An XML Query language,
*
* Appendix C, XQuery 1.0 and XPath 2.0 Functions and Operators,
* and its associated specifications; implementation-defined
* errors may be raised
* @param line the line number in the query string where the error occured.
* Line numbering starts at 1
. -1
if unknown
* @param column the column number in the query string where the error occured.
* Column numbering starts at 1
. -1
if unknown
* @param position the position in the query string where the error occured. This
* is a 0
based position. -1
if unknown
* @param moduleURI the module URI of the module in which the error occurred.
* null
when it is the main module or when the module is
* unknown
* @param errorObject an XQSequence
representing the error object passed to
* fn:error()
. null
if this error was not
* triggered by fn:error()
or when the error object is
* not available.
* @param stackTrace the XQuery stack trace where the error occurred. null
* if not available
*/
public XQQueryException(String message, String vendorCode, QName errorCode,
int line, int column, int position,
String moduleURI, XQSequence errorObject, XQStackTraceElement[] stackTrace) {
super(message, vendorCode);
this.errorCode = errorCode;
this.line = line;
this.column = column;
this.position = position;
this.moduleURI = moduleURI;
this.errorObject = errorObject;
this.stackTrace = stackTrace;
}
/**
* Gets the code identifying the error according to the standard as
* described in Appendix F, XQuery 1.0:
* An XML Query language,
* Appendix C, XQuery 1.0 and XPath 2.0 Functions and Operators, and
* its associated specifications; imlementation-defined errors may also be raised;
* finally the error code may also be specified in the query using fn:error()
.
*
* @return the code identifying the error, or null
* if not available
*/
public QName getErrorCode() {
return errorCode;
}
/**
* Gets an XQSequence
representing the error object passed to
* fn:error()
. Returns null
if this error was not triggered by
* fn:error()
or when the error object is not available.
*
* @return the sequence passed to fn:error()
,
* null
if not available
*/
public XQSequence getErrorObject() {
return errorObject;
}
/**
* Gets the character position in the query string where this exception
* occurred.
*
* This is a 0
based position. -1
if unknown.
*
* @return the character position in the query string where the
* exception occurred
*/
public int getPosition() {
return position;
}
/**
* Returns the query stack stackTrace when the exception occurred, or null if
* none. On some implementations only the top frame may be visible.
*
* @return the stackTrace where the exception occurred
*/
public XQStackTraceElement[] getQueryStackTrace() {
return stackTrace;
}
/**
* Gets the module URI of the module in which the error occurred.
* null
when it is the main module or when the module is
* unknown.
*
* @return the module URI or null
*/
public String getModuleURI() {
return moduleURI;
}
/**
* Gets the line number in the query string where the error occurred.
*
* Line numbering starts at 1
. -1
is returned
* if the line number is unknown. If the implementation does not support this method,
* it must return -1
*
* @return the line number in the query string where
* the error occurred
*/
public int getLineNumber() {
return line;
}
/**
* Gets the column number in the query string where the error occurred.
*
* Column numbering starts at 1
. -1
is returned
* if the column number is unknown. If the implementation does not support this method,
* it must return -1
*
* @return the column number in the query string where
* the error occurred
*/
public int getColumnNumber() {
return column;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy