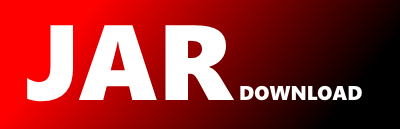
javax.xml.xquery.XQStaticContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Saxon-HE Show documentation
Show all versions of Saxon-HE Show documentation
The XSLT and XQuery Processor
/*
* Copyright 2003, 2004, 2005, 2006, 2007, 2008 Oracle. All rights reserved.
*/
package javax.xml.xquery;
/**
* An XQStaticContext
represents default values for various
*
* XQuery Static Context Components. Further it includes the
* static XQJ properties for an XQExpression
or
* XQPreparedExpression
object.
*
* The following XQuery Static Context Components are supported through the
* XQStaticContext
interface:
*
* - Statically known namespaces
*
- Default element/type namespace
*
- Default function namespace
*
- Context item static type
*
- Default collation
*
- Construction mode
*
- Ordering mode
*
- Default order for empty sequences
*
- Boundary-space policy
*
- Copy-namespaces mode
*
- Base URI
*
* As described in the XQuery specification, each of these default values can
* be overridden or augmented in the query prolog.
*
* In addition XQStaticContext
includes the static XQJ properties
* for an XQExpression
or XQPreparedExpression
object:
*
* - Binding mode
*
- Holdability of the result sequences
*
- Scrollability of the result sequences
*
- Query language
*
- Query timeout
*
*
* Note that XQStaticContext
is a value object, changing attributes in
* such object doesn't affect any existing XQExpression
or
* XQPreparedExpression
object.
* In order to take effect, the application needs to explicitly change the XQConnection
* default values, or specify an XQStaticContext
object when creating an
* XQExpression
or XQPreparedExpression
.
*
* XQConnection conn = XQDatasource.getConnection();
* // get the default values from the implementation
* XQStaticContext cntxt = conn.getStaticContext();
* // change the base uri
* cntxt.setBaseURI("http://www.foo.com/xml/");
* // change the implementation defaults
* conn.setStaticContext(cntxt);
*
* // create an XQExpression using the new defaults
* XQExpression expr1 = conn.createExpression();
*
* // creat an XQExpression, using BaseURI "file:///root/user/john/"
* cntxt.setBaseURI("file:///root/user/john/");
* XQExpression expr2 = conn.createExpression(cntxt);
* ...
*
*/
public interface XQStaticContext {
/**
* Returns the prefixes of all the statically known namespaces.
* Use the getNamespaceURI
method to look up the namespace URI
* corresponding to a specific prefix.
*
* @return String
array containing the namespace prefixes.
* Cannot be null
*/
public String[] getNamespacePrefixes();
/**
* Retrieves the namespace URI associated with a prefix. An XQException
* is thrown if an unknown prefix is specified, i.e. a prefix not returned by the
* getInScopeNamespacePrefixes
method.
*
* @param prefix the prefix for which the namespace URI is sought. Cannot
* be null
* @return the namespace URI. Cannot be null
* @throws XQException if a null
prefix is specified or if the prefix
* is unknown
*/
public String getNamespaceURI(String prefix) throws XQException;
/**
* Declares a namespace prefix and associates it with a namespace URI. If the namespace URI is
* the empty string, the prefix is removed from the in-scope namespace definitions.
*
* @param prefix the prefix for the namespace URI
* @param uri the namespace URI. An empty string
* undeclares the specific prefix. Cannot be null
* @throws XQException if (1) a null
prefix, or (2) a null
namespace
* URI is specified
*/
public void declareNamespace(String prefix, String uri) throws XQException;
/**
* Gets the URI of the default element/type namespace, the empty string
* if not set.
*
* @return the URI of the default element/type namespace,
* if set, else the empty string. Cannot be null
*/
public String getDefaultElementTypeNamespace();
/**
* Sets the URI of the default element/type namespace, the empty string
* to make it unspecified.
*
* @param uri the namespace URI of the default element/type namespace,
* the empty string to make it unspecified.
* Cannot be null
.
* @throws XQException if a null
uri is specified
*/
public void setDefaultElementTypeNamespace(String uri) throws XQException;
/**
* Gets the URI of the default function namespace, the empty string
* if not set.
*
* @return the URI of the default function namespace,
* if set, else the empty string. Cannot be null
*/
public String getDefaultFunctionNamespace();
/**
* Sets the URI of the default function namespace, the empty string
* to make it unspecified.
*
* @param uri the namespace URI of the default function namespace,
* the empty string to make it unspecified.
* Cannot be null
.
* @throws XQException if a null
URI is specified
*/
public void setDefaultFunctionNamespace(String uri) throws XQException;
/**
* Gets the static type of the context item. null
if unspecified.
*
* @return the static type of the context item,
* if set, else null
*/
public XQItemType getContextItemStaticType();
/**
* Sets the static type of the context item, specify null
* to make it unspecified.
*
* @param contextItemType the static type of the context item;
* null
if unspecified.
* @throws XQException if the contextItemType
is not
* a valid XQItemType
*/
public void setContextItemStaticType(XQItemType contextItemType)
throws XQException;
/**
* Gets the URI of the default collation.
*
* @return the URI of the default collation.
* Cannot be null
.
*/
public String getDefaultCollation();
/**
* Sets the URI of the default collation.
*
* @param uri the namespace URI of the default collation.
* Cannot be null
.
* @throws XQException if a null
URI is specified
*/
public void setDefaultCollation(String uri) throws XQException;
/**
* Gets the construction mode defined in the static context.
*
* @return construction mode value. One of:
* XQConstants.CONSTRUCTION_MODE_PRESERVE
,
* XQConstants.CONSTRUCTION_MODE_STRIP
*/
public int getConstructionMode();
/**
* Sets the construction mode in the static context.
*
* @param mode construction mode value. One of:
* XQConstants.CONSTRUCTION_MODE_PRESERVE
,
* XQConstants.CONSTRUCTION_MODE_STRIP
.
* @throws XQException the specified mode is different from
* XQConstants.CONSTRUCTION_MODE_PRESERVE
,
* XQConstants.CONSTRUCTION_MODE_STRIP
*/
public void setConstructionMode(int mode) throws XQException;
/**
* Gets the ordering mode defined in the static context.
*
* @return ordering mode value. One of:
* XQConstants.ORDERING_MODE_ORDERED
,
* XQConstants.ORDERING_MODE_UNORDERED
.
*/
public int getOrderingMode();
/**
* Sets the ordering mode in the static context.
*
* @param mode ordering mode value. One of:
* XQConstants.ORDERING_MODE_ORDERED
,
* XQConstants.ORDERING_MODE_UNORDERED
.
* @throws XQException the specified mode is different from
* XQConstants.ORDERING_MODE_ORDERED
,
* XQConstants.ORDERING_MODE_UNORDERED
*/
public void setOrderingMode(int mode) throws XQException;
/**
* Gets the default order for empty sequences defined in the static context.
*
* @return default order for empty sequences value. One of:
* XQConstants.DEFAULT_ORDER_FOR_EMPTY_SEQUENCES_GREATEST
,
* XQConstants.DEFAULT_ORDER_FOR_EMPTY_SEQUENCES_LEAST
.
*/
public int getDefaultOrderForEmptySequences();
/**
* Sets the default order for empty sequences in the static context.
*
* @param order the default order for empty sequences. One of:
* XQConstants.DEFAULT_ORDER_FOR_EMPTY_SEQUENCES_GREATEST
,
* XQConstants.DEFAULT_ORDER_FOR_EMPTY_SEQUENCES_LEAST
.
* @throws XQException the specified order for empty sequences is different from
* XQConstants.DEFAULT_ORDER_FOR_EMPTY_SEQUENCES_GREATEST
,
* XQConstants.DEFAULT_ORDER_FOR_EMPTY_SEQUENCES_LEAST
*/
public void setDefaultOrderForEmptySequences(int order) throws XQException;
/**
* Gets the boundary-space policy defined in the static context.
*
* @return the boundary-space policy value. One of:
* XQConstants.BOUNDARY_SPACE_PRESERVE
,
* XQConstants.BOUNDARY_SPACE_STRIP
.
*/
public int getBoundarySpacePolicy();
/**
* Sets the boundary-space policy in the static context.
*
* @param policy boundary space policy. One of:
* XQConstants.BOUNDARY_SPACE_PRESERVE
,
* XQConstants.BOUNDARY_SPACE_STRIP
.
* @throws XQException the specified mode is different from
* XQConstants.BOUNDARY_SPACE_PRESERVE
,
* XQConstants.BOUNDARY_SPACE_STRIP
*/
public void setBoundarySpacePolicy(int policy) throws XQException;
/**
* Gets the preserve part of the copy-namespaces mode
* defined in the static context.
*
* @return construction mode value. One of:
* XQConstants.COPY_NAMESPACES_MODE_PRESERVE
,
* XQConstants.COPY_NAMESPACES_MODE_NO_PRESERVE
.
*/
public int getCopyNamespacesModePreserve();
/**
* Sets the preserve part of the copy-namespaces mode in the static context.
*
* @param mode ordering mode value. One of:
* XQConstants.COPY_NAMESPACES_MODE_PRESERVE
,
* XQConstants.COPY_NAMESPACES_MODE_NO_PRESERVE
.
* @throws XQException the specified mode is different from
* XQConstants.COPY_NAMESPACES_MODE_PRESERVE
,
* XQConstants.COPY_NAMESPACES_MODE_NO_PRESERVE
*/
public void setCopyNamespacesModePreserve(int mode) throws XQException;
/**
* Gets the inherit part of the copy-namespaces mode
* defined in the static context.
*
* @return construction mode value. One of:
* XQConstants.COPY_NAMESPACES_MODE_INHERIT
,
* XQConstants.COPY_NAMESPACES_MODE_NO_INHERIT
.
*/
public int getCopyNamespacesModeInherit();
/**
* Sets the inherit part of the copy-namespaces mode in the static context.
*
* @param mode ordering mode value. One of:
* XQConstants.COPY_NAMESPACES_MODE_INHERIT
,
* XQConstants.COPY_NAMESPACES_MODE_NO_INHERIT
.
* @throws XQException the specified mode is different from
* XQConstants.COPY_NAMESPACES_MODE_INHERIT
,
* XQConstants.COPY_NAMESPACES_MODE_NO_INHERIT
*/
public void setCopyNamespacesModeInherit(int mode) throws XQException;
/**
* Gets the Base URI, if set in the static context, else the empty string.
*
* @return the base URI, if set, else the empty string.
* Cannot be null
..
*/
public String getBaseURI();
/**
* Sets the Base URI in the static context, specify the empty string to make it undefined.
*
* @param baseUri the new baseUri, or empty string to make it undefined.
* Cannot be null
.
* @throws XQException if a null
base uri is specified
*/
public void setBaseURI(String baseUri) throws XQException;
/**
* Gets the value of the binding mode property.
* By default an XQJ implementation operates in immediate binding mode.
*
* @return the binding mode. One of
* XQConstants.BINDING_MODE_IMMEDIATE
,
* orXQConstants.BINDING_MODE_DEFERRED
.
*/
public int getBindingMode();
/**
* Sets the binding mode property.
* By default an XQJ implementation operates in immediate binding mode.
*
* @param bindingMode the binding mode. One of:
* XQConstants.BINDING_MODE_IMMEDIATE
,
* orXQConstants.BINDING_MODE_DEFERRED
.
* @throws XQException the specified mode is different from
* XQConstants.BINDING_MODE_IMMEDIATE
,
* XQConstants.BINDING_MODE_DEFERRED
*/
public void setBindingMode(int bindingMode) throws XQException;
/**
* Gets the value of the holdability property.
*
* @return the type of a result's holdability. One of:
* XQConstants.HOLDTYPE_HOLD_CURSORS_OVER_COMMIT
,
* or XQConstants.HOLDTYPE_CLOSE_CURSORS_AT_COMMIT
.
*/
public int getHoldability();
/**
* Sets the holdability property.
*
* @param holdability the holdability of the result. One of:
* XQConstants.HOLDTYPE_HOLD_CURSORS_OVER_COMMIT
,
* or XQConstants.HOLDTYPE_CLOSE_CURSORS_AT_COMMIT
.
* @throws XQException the specified holdability is different from
* XQConstants.HOLDTYPE_HOLD_CURSORS_OVER_COMMIT
,
* XQConstants.HOLDTYPE_CLOSE_CURSORS_AT_COMMIT
*/
public void setHoldability(int holdability) throws XQException;
/**
* Gets the value of the language type and version property.
* By default an XQJ implementation's default is XQConstants.LANGTYPE_XQUERY
.
*
* @return the language type and version. One of:
* XQConstants.LANGTYPE_XQUERY
,
* or XQConstants.LANGTYPE_XQUERYX
* or a negative value indicating a vendor specific
* query language type and version.
*/
public int getQueryLanguageTypeAndVersion();
/**
* Sets the input query language type and version.
* When this is set to a particular language type and version, then the
* query is assumed to be in that language and version.
*
* @param langType the query language type and version of the
* inputs. One of: XQConstants.LANGTYPE_XQUERY
* (default), or XQConstants.LANGTYPE_XQUERYX
.
* A negative number indicates a vendor specific
* query language type and version.
* @throws XQException the specified langtype is different from
* XQConstants.LANGTYPE_XQUERY
,
* XQConstants.LANGTYPE_XQUERYX
and is not negative
*/
public void setQueryLanguageTypeAndVersion(int langType) throws XQException;
/**
* Gets the value of the scrollability property.
* By default query results are forward only.
*
* @return the type of a result's scrollability. One of:
* XQConstants.SCROLLTYPE_FORWARD_ONLY
, or
* XQConstants.SCROLLTYPE_SCROLLABLE
.
*/
public int getScrollability();
/**
* Sets the scrollability of the result sequence.
* By default query results are forward only.
*
* @param scrollability the scrollability of the result. One of:
* XQConstants.SCROLLTYPE_FORWARD_ONLY
, or
* XQConstants.SCROLLTYPE_SCROLLABLE
.
* @throws XQException the specified crollability type is different from
* XQConstants.SCROLLTYPE_FORWARD_ONLY
,
* XQConstants.SCROLLTYPE_SCROLLABLE
*/
public void setScrollability(int scrollability) throws XQException;
/**
* Retrieves the number of seconds an implementation will wait for a
* query to execute.
*
* @return the query execution timeout value in seconds.
* A value of 0 indicates no limit.
*/
public int getQueryTimeout();
/**
* Sets the number of seconds an implementation will wait for a
* query to execute. If the implementation does not support query timeout
* it can ignore the specified timeout value.
* It the limit is exceeded, the behavor of the query is the same as an
* execution of a cancel by another thread.
*
* @param seconds the query execution timeout value in seconds.
* A value of 0 indicates no limit
* @throws XQException if the passed in value is negative
*/
public void setQueryTimeout(int seconds) throws XQException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy