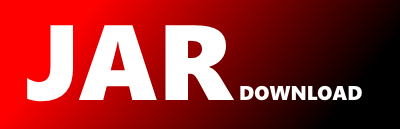
javax.xml.xquery.XQStackTraceElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Saxon-HE Show documentation
Show all versions of Saxon-HE Show documentation
The XSLT and XQuery Processor
/*
* Copyright 2003, 2004, 2005, 2006, 2007, 2008 Oracle. All rights reserved.
*/
package javax.xml.xquery;
import javax.xml.namespace.QName;
import java.io.Serializable;
/**
* This class represents a frame in a stack trace, akin to the
* java.lang.StackTraceElement
but for XQuery callstacks
* instead of Java.
*
* @see XQQueryException#getQueryStackTrace XQQueryException.getQueryStackTrace
*/
public class XQStackTraceElement implements Serializable {
private String module;
private int line = -1;
private int column = -1;
private int position = -1;
private QName function;
private XQStackTraceVariable[] variables;
/**
* Construct an XQStackTraceElement
object representing
* a frame in a stack trace.
*
* @param moduleURI the module URI containing the execution point representing
* the stack trace element. null
when it is the main
* module or when the module is unknown
* @param line the line number in the query string where the error occured.
* Line numbering starts at 1
. -1
if unknown
* @param column the column number in the query string where the error occured.
* Column numbering starts at 1
. -1
if unknown
* @param position the position in the query string where the error occured. This
* is a 0
based position. -1 if unknown
* @param function the QName
of the function in which the exception occurred,
* or null
if it occurred outside an enclosing function
* @param variables the variables in scope at this execution point,
* or null
if no variable value retrieval is possible
*/
public XQStackTraceElement(String moduleURI, int line, int column, int position,
QName function, XQStackTraceVariable[] variables) {
this.module = moduleURI;
this.line = line;
this.column = column;
this.position = position;
this.function = function;
this.variables = variables;
}
/**
* Gets the module URI containing the execution point represented by this
* stack trace element.
* null
when it is the main module or when the module is
* unknown.
*
* @return the module URI containing the excution point
* represented by the stack trace element or null
*/
public String getModuleURI() {
return module;
}
/**
* Gets the character position in the query string containing the execution
* point represented by this stack trace element.
*
* This is a 0
based position. -1
if unknown.
*
* @return the character position in the query string containing the
* execution point represented by the stack trace element
*/
public int getPosition() {
return position;
}
/**
* Gets the line number in the query string containing the execution
* point represented by this stack trace element.
*
* Line numbering starts at 1
. -1
is returned
* if the line number is unknown. If the implementation does not support this method,
* it must return -1
*
* @return the line number in the query string containing the
* execution point represented by the stack trace element
*/
public int getLineNumber() {
return line;
}
/**
* Gets the column number in the query string containing the execution
* point represented by this stack trace element.
*
* Column numbering starts at 1
. -1
is returned
* if the column number is unknown. If the implementation does not support this method,
* it must return -1
*
* @return the column number in the query string containing the
* execution point represented by the stack trace element
*/
public int getColumnNumber() {
return column;
}
/**
* Gets the QName
of the function in which the error occurred,
* or null
if it occurred outside an enclosing function (in a main module).
*
* @return the QName
of the function in which the error
* occurred for this stack element or null
if it
* occurred outside an enclosing function
*/
public QName getFunctionQName() {
return function;
}
/**
* Gets the variables in scope at this execution point, or null
if no
* variable value retrieval is possible.
*
* @return the variables in scope at this execution point, or
* null
if no variable value retrieval is
* possible.
*/
public XQStackTraceVariable[] getVariables() {
return variables;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy