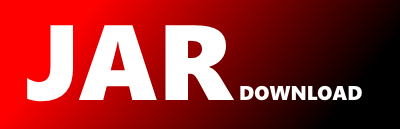
net.sf.saxon.ma.trie.Option Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Saxon-HE Show documentation
Show all versions of Saxon-HE Show documentation
The XSLT and XQuery Processor
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
// Copyright (c) 2012 Michael Froh.
// This Source Code Form is subject to the terms of the Mozilla Public License, v. 2.0.
// If a copy of the MPL was not distributed with this file, You can obtain one at http://mozilla.org/MPL/2.0/.
// This Source Code Form is "Incompatible With Secondary Licenses", as defined by the Mozilla Public License, v. 2.0.
////////////////////////////////////////////////////////////////////////////////////////////////////////////////////
package net.sf.saxon.ma.trie;
import java.util.Collections;
import java.util.Iterator;
import java.util.NoSuchElementException;
public abstract class Option implements Iterable {
public abstract T get();
public abstract boolean isDefined();
public final T getOrElse(T defaultVal) {
return isDefined() ? get() : defaultVal;
}
public Iterator iterator() {
return isDefined() ? Collections.singleton(get()).iterator() :
Collections.emptySet().iterator();
}
public static Option option(T value) {
if (value == null) {
return none();
}
return some(value);
}
// Factory method to return the singleton None instance
@SuppressWarnings({"unchecked"})
public static Option none() {
return NONE;
}
// Factory method to return a non-empty Some instance
public static Option some(final T value) {
return new Some(value);
}
private static None NONE = new None();
private static class None extends Option {
@Override
public Object get() {
throw new NoSuchElementException("get() called on None");
}
@Override
public boolean isDefined() {
return false;
}
@Override
public String toString() {
return "None";
}
}
private static class Some extends Option {
private final T value;
public Some(final T value) {
this.value = value;
}
@Override
public T get() {
return value;
}
@Override
public boolean isDefined() {
return true;
}
@Override
public String toString() {
return "Some(" + value + ")";
}
public boolean equals(Object other) {
return other instanceof Some && ((Some) other).value.equals(value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy