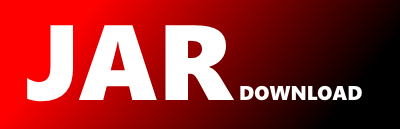
net.sf.saxon.tree.iter.MappingJavaIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of Saxon-HE Show documentation
Show all versions of Saxon-HE Show documentation
The XSLT and XQuery Processor
package net.sf.saxon.tree.iter;
import java.util.Iterator;
/**
* A Java Iterator which applies a mapping function to each item in an input sequence
* @param the type of the input items
* @param the type of the delivered item
*/
public class MappingJavaIterator implements Iterator {
private Iterator input;
private Mapper mapper;
/**
* Create a mapping iterator
* @param in the input sequence
* @param mapper the mapping function to be applied to each item in the ipnut sequence to
* generate the corresponding item in the result sequence
*/
public MappingJavaIterator(Iterator in, Mapper mapper) {
this.input = in;
this.mapper = mapper;
}
public boolean hasNext() {
return input.hasNext();
}
public T next() {
while (true) {
T next = mapper.map(input.next());
if (next != null) {
return next;
}
}
}
public void remove() {
input.remove();
}
/**
* Interface defining the mapping function
* @param the type of the input items
* @param the type of the output items
*/
public interface Mapper {
/**
* Map one input item to one output item
* @param in the input item
* @return an output item, or null. Any nulls are omitted from the result sequence delivered by the mapping
* iterator.
*/
T map(S in);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy