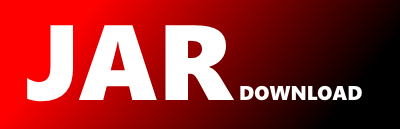
net.sf.sfac.gui.UiUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sfac-core Show documentation
Show all versions of sfac-core Show documentation
This project is the core part of the Swing Framework and Components (SFaC).
It contains the Swing framework classes and the graphical component classes.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2009 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.sfac.gui;
import java.awt.Color;
import java.awt.Component;
import java.awt.Container;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.GridLayout;
import java.awt.Image;
import java.awt.image.ImageObserver;
import java.util.StringTokenizer;
import javax.swing.JComponent;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
/**
* Various unrelated utility methods (see each method javadoc).
*
* @author Olivier Berlanger
*/
public class UiUtils {
/**
* Return a component displaying the text in multiple lines. If the text contains more lines than nbrMax, it is put in a
* scrollPane sized to display nbrMax lines.
*
* @param text
* the multi-line text to display (new lines are specified by '\n')
* @param nbrMax
* maximum number of line displayed in the component.
*/
public static JComponent getMultiLineComponent(String text, int nbrMax) {
StringTokenizer st = new StringTokenizer(text, "\n");
int len = st.countTokens();
JPanel linesPanel = new JPanel(new GridLayout(len, 1));
for (int i = 0; i < len; i++) {
String line = st.nextToken();
linesPanel.add(new JLabel(line));
}
if (len <= nbrMax) return linesPanel;
// Put the linesPanel on a scroll pane
Dimension dim = linesPanel.getPreferredSize();
JScrollPane scroll = new JScrollPane(linesPanel, JScrollPane.VERTICAL_SCROLLBAR_AS_NEEDED,
JScrollPane.HORIZONTAL_SCROLLBAR_NEVER);
scroll.setPreferredSize(new Dimension(dim.width + 20, (dim.height * nbrMax) / len));
return scroll;
}
/**
* Paint a 'best fit' image in the proposed rectangle.
*
* @return true if the image is fully painted.
*/
@SuppressWarnings("cast")
public static boolean paintImage(Graphics g, Image image, int x, int y, int width, int height, Color bg, ImageObserver obs) {
boolean succeed = false;
int imWidth = image.getWidth(obs);
int imHeight = image.getHeight(obs);
if ((imWidth > 0) && (imHeight > 0)) {
int posX;
int posY;
int paintedWidth;
int paintedHeight;
// resize the image
float ratio = ((float) imWidth) / ((float) imHeight);
if (imWidth < width) {
if (imHeight < height) {
paintedWidth = imWidth;
paintedHeight = imHeight;
posX = (width - paintedWidth) / 2;
posY = (height - paintedHeight) / 2;
} else {
paintedWidth = (int) (((float) height) * ratio);
paintedHeight = height;
posX = (width - paintedWidth) / 2;
posY = 0;
}
} else {
if (imHeight <= height) {
paintedWidth = width;
paintedHeight = (int) (((float) width) / ratio);
posX = 0;
posY = (height - paintedHeight) / 2;
} else {
int tempWidth = (int) (((float) height) * ratio);
int tempHeight = (int) (((float) width) / ratio);
if (tempHeight <= height) {
paintedWidth = width;
paintedHeight = tempHeight;
posX = 0;
posY = (height - paintedHeight) / 2;
} else {
paintedWidth = tempWidth;
paintedHeight = height;
posX = (width - paintedWidth) / 2;
posY = 0;
}
}
}
// paint image (in the the background)
if (bg == null) succeed = g.drawImage(image, x + posX, y + posY, paintedWidth, paintedHeight, obs);
else succeed = g.drawImage(image, x + posX, y + posY, paintedWidth, paintedHeight, bg, obs);
}
return succeed;
}
/**
* Resize a label font and optionally center it.
* This method is typically used to make title labels.
*
* @param lbl
* The label to reformat
* @param factor
* multiplying factor for the new font size.
* @param centerText
* if true the label text is aligned to the center of the label.
*/
public static void resizeLabelFont(JComponent lbl, float factor, boolean centerText) {
Font labelFont = lbl.getFont();
lbl.setFont(labelFont.deriveFont(factor * labelFont.getSize2D()));
if (centerText && (lbl instanceof JLabel)) {
((JLabel) lbl).setHorizontalAlignment(JLabel.CENTER);
}
}
/**
* Recursively set background on a component tree.
*/
public static void setBackground(Component cmp, Color col) {
cmp.setBackground(col);
if (cmp instanceof Container) {
Container cont = (Container) cmp;
Component[] subCmp = cont.getComponents();
if (subCmp != null) {
for (Component sub : subCmp) {
setBackground(sub, col);
}
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy