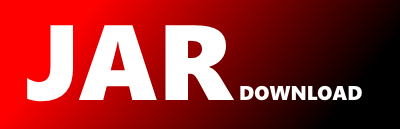
net.sf.sfac.gui.asynch.WaitManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sfac-core Show documentation
Show all versions of sfac-core Show documentation
This project is the core part of the Swing Framework and Components (SFaC).
It contains the Swing framework classes and the graphical component classes.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2009 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.sfac.gui.asynch;
import java.awt.AWTEvent;
import java.awt.Component;
import java.awt.Cursor;
import java.awt.EventQueue;
import java.awt.Toolkit;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.InputEvent;
import javax.swing.Timer;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* This utility class allows to set a hourglass and block the input event dispatching during a long action (without blocking the
* other events like repaint). It defines a trigger time (+- 400 milliseconds) under which no hourglass is displayed (to avoid a
* flickering hourglass) and a timeout time (+- 200 000 milliseconds = 3.3 minutes) after which the event blocking is stopped (to
* avoid deadlock).
*
* @author Olivier Berlanger
*/
public class WaitManager {
private static final Log log = LogFactory.getLog(WaitManager.class);
/** Default application cursor (typically white arrow). */
private static final Cursor DEFAULT_CURSOR = Cursor.getDefaultCursor();
/** Wait application cursor (typically hourglass). */
private static final Cursor WAIT_CURSOR = Cursor.getPredefinedCursor(Cursor.WAIT_CURSOR);
/** The main component (typically frame) where the cursor is set. */
private Component controlledComp;
/** The busy action count (event are blocked when busyCount>0). */
private int busyCount;
/** The timer user to implement triggering and timeout times. */
private Timer tim;
/** The number of timer ticks (every 200 millis) received since event blocking. */
private int nbrTick;
/** The eventQueue instance consuming all input events. */
private BlockinEventQueue blocker;
/** The currently displayed cursor (on the controlledComp). */
private Cursor currentCursor;
/**
* Create a WaitManager associated to the given component.
*
* @param controlledCmp
* The main component (typically a frame) where the wait cursor is set.
*/
public WaitManager(Component controlledCmp) {
controlledComp = controlledCmp;
tim = new Timer(200, new ActionListener() {
public void actionPerformed(ActionEvent e) {
timerTick();
}
});
}
/**
* Set the WaitManager busy (= blocking the event and showing wait cursor) or not busy. The WaitManager will be not busy again
* when a setBusy(false)
is called for each setBusy(true)
.
*
* @param busy
* true to increment the busyCount
, false to decrement it.
*/
public void setBusy(boolean busy) {
// if (!SwingUtilities.isEventDispatchThread()) throw new IllegalStateException("You must use EventDispatchThread");
if (busy) {
if (busyCount == 0) {
nbrTick = 0;
blocker = new BlockinEventQueue();
blocker.start();
tim.start();
}
busyCount++;
} else {
if (busyCount <= 0) throw new IllegalStateException("Cannot set busy false when not busy");
busyCount--;
if (busyCount == 0) {
nbrTick = 0;
blocker.stop();
tim.stop();
if (currentCursor != DEFAULT_CURSOR) {
controlledComp.setCursor(DEFAULT_CURSOR);
currentCursor = DEFAULT_CURSOR;
}
}
}
}
/**
* Check if this WaitManager
is currently busy (= blocking the event and showing wait cursor).
*
* @return true iff this WaitManager
is currently busy
*/
public boolean isBusy() {
return busyCount > 0;
}
/**
* Notification that 200 more millis have elapsed since we were busy.
*/
void timerTick() {
nbrTick++;
if ((nbrTick >= 2) && (currentCursor != WAIT_CURSOR)) {
controlledComp.setCursor(WAIT_CURSOR);
currentCursor = WAIT_CURSOR;
}
if (nbrTick > 1000) {
log.info("TIME OUT for hourglass");
while (isBusy()) {
setBusy(false);
}
}
}
/**
* EventQueue
object consuming all the input events.
*
* @author Olivier Berlanger
*/
static class BlockinEventQueue extends EventQueue {
BlockinEventQueue() {
}
void start() {
Toolkit.getDefaultToolkit().getSystemEventQueue().push(this);
}
void stop() {
pop();
}
@Override
protected void dispatchEvent(AWTEvent event) {
// Block all input event
if (event instanceof InputEvent) {
((InputEvent) event).consume();
} else if (event.getClass().getName().endsWith("ComponentWorkRequest")) {
// hack to avoid nullPoinetrException in repaint
} else {
super.dispatchEvent(event);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy