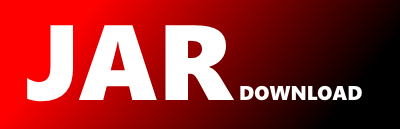
net.sf.sfac.gui.cmp.ConsoleDialog Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sfac-core Show documentation
Show all versions of sfac-core Show documentation
This project is the core part of the Swing Framework and Components (SFaC).
It contains the Swing framework classes and the graphical component classes.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2009 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.sfac.gui.cmp;
import java.awt.BorderLayout;
import java.awt.Dialog;
import java.awt.Dimension;
import java.awt.Frame;
import java.awt.Rectangle;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.IOException;
import java.io.OutputStream;
import javax.swing.BoxLayout;
import javax.swing.JButton;
import javax.swing.JCheckBox;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.SwingUtilities;
import net.sf.sfac.lang.LanguageSupport;
import net.sf.sfac.setting.Settings;
/**
* This class is a JDialog displaying a big text area that can be used as a console to display text output.
* To add text in the console, use the append(..)
methods of this class or an OutputStream provided by the
* getConsoleStream()
method.
*
* @author Olivier Berlanger
*/
@SuppressWarnings("serial")
public class ConsoleDialog extends SizedDialog {
private JTextArea textArea;
private JButton clearButton;
private JCheckBox scrollLockCB;
private JScrollPane scrollPane;
public ConsoleDialog(Frame owner, String title, Settings sett) {
super(owner, sett, LanguageSupport.getOptionalLocalizedString("CONSOLE", "Console"));
setTitle(title);
buildGui();
}
public ConsoleDialog(Dialog owner, String title, Settings sett) {
super(owner, sett, LanguageSupport.getOptionalLocalizedString("CONSOLE", "Console"));
setTitle(title);
buildGui();
}
private void buildGui() {
// main area displaying console text
textArea = new JTextArea();
scrollPane = new JScrollPane(textArea);
// console control pane
JPanel bottomPane = new JPanel();
bottomPane.setLayout(new BoxLayout(bottomPane, BoxLayout.X_AXIS));
scrollLockCB = new JCheckBox(LanguageSupport.getOptionalLocalizedString("SCROLL_LOCK", "Scroll Lock"));
clearButton = new JButton(LanguageSupport.getOptionalLocalizedString("CLEAR", "Clear"));
clearButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent newE) {
clear();
}
});
bottomPane.add(scrollLockCB);
bottomPane.add(clearButton);
// put all together
JPanel contentPane = (JPanel) getContentPane();
contentPane.add(scrollPane, BorderLayout.CENTER);
contentPane.add(bottomPane, BorderLayout.SOUTH);
}
public boolean isScrollLock() {
return scrollLockCB.isSelected();
}
public void setScrollLock(boolean scrollLock) {
scrollLockCB.setSelected(scrollLock);
}
public void clear() {
textArea.setText("");
}
public void write(String txt) {
append(txt);
}
public void writeln(String txt) {
append(txt);
append("\n");
}
public void append(final String txt) {
if (SwingUtilities.isEventDispatchThread()) {
textArea.append(txt);
if (!isScrollLock()) {
Dimension textAreaSize = textArea.getSize();
int textAreaHeight = textAreaSize.height;
if (textAreaHeight > 16) {
Rectangle bottomRect = new Rectangle(0, textAreaHeight - 16, 16, 16);
textArea.scrollRectToVisible(bottomRect);
}
}
} else {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
append(txt);
}
});
}
}
public void append(int i) {
append(String.valueOf(i));
}
public void append(char ch) {
append(String.valueOf(ch));
}
public void append(byte[] b) {
append(new String(b));
}
public void append(byte[] b, int start, int len) {
append(new String(b, start, len));
}
public OutputStream getConsoleStream() {
return new ConsoleStream();
}
class ConsoleStream extends OutputStream {
@Override
public void write(int b) throws IOException {
append((char) b);
}
@Override
public void write(byte[] b) throws IOException {
append(b);
}
@Override
public void write(byte[] b, int start, int len) throws IOException {
append(b, start, len);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy