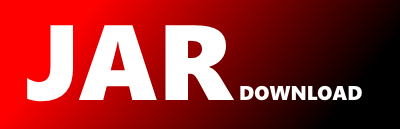
net.sf.sfac.gui.cmp.RollOverSplitPane Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sfac-core Show documentation
Show all versions of sfac-core Show documentation
This project is the core part of the Swing Framework and Components (SFaC).
It contains the Swing framework classes and the graphical component classes.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2009 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.sfac.gui.cmp;
import java.awt.Color;
import java.awt.Component;
import java.awt.Graphics;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import javax.swing.JSplitPane;
import javax.swing.border.Border;
import javax.swing.plaf.basic.BasicSplitPaneDivider;
import javax.swing.plaf.basic.BasicSplitPaneUI;
import net.sf.sfac.setting.Settings;
/**
* Split pane with invisible separator bar. The separator bar become visible only when you roll-over the mouse on it. In addition
* this component can automatically store/retrive the divider location in a Settings
object.
*
* @author Olivier Berlanger
* @see net.sf.sfac.setting.Settings
*/
@SuppressWarnings("serial")
public class RollOverSplitPane extends JSplitPane {
/** Settings where the divider location is stored (if set). */
private Settings sett;
/** Key of the divider location value in the Settings
. */
private String dividerSettingKey;
/**
* Creates a new RollOverSplitPane
configured to arrange the child components side-by-side horizontally with no
* continuous layout, using two buttons for the components.
*/
public RollOverSplitPane() {
this(JSplitPane.HORIZONTAL_SPLIT, false, null, null);
}
/**
* Creates a new RollOverSplitPane
configured with the specified orientation and no continuous layout.
*
* @param splitOrientation
* JSplitPane.HORIZONTAL_SPLIT
or JSplitPane.VERTICAL_SPLIT
* @exception IllegalArgumentException
* if orientation
is not one of HORIZONTAL_SPLIT or VERTICAL_SPLIT.
*/
public RollOverSplitPane(int splitOrientation) {
this(splitOrientation, false, null, null);
}
/**
* Creates a new RollOverSplitPane
with the specified orientation and redrawing style.
*
* @param splitOrientation
* JSplitPane.HORIZONTAL_SPLIT
or JSplitPane.VERTICAL_SPLIT
* @param splitContinuousLayout
* a boolean, true for the components to redraw continuously as the divider changes position, false to wait until the
* divider position stops changing to redraw
* @exception IllegalArgumentException
* if orientation
is not one of HORIZONTAL_SPLIT or VERTICAL_SPLIT
*/
public RollOverSplitPane(int splitOrientation, boolean splitContinuousLayout) {
this(splitOrientation, splitContinuousLayout, null, null);
}
/**
* Creates a new RollOverSplitPane
with the specified orientation and with the specified components that do not do
* continuous redrawing.
*
* @param splitOrientation
* JSplitPane.HORIZONTAL_SPLIT
or JSplitPane.VERTICAL_SPLIT
* @param leftCmp
* the Component
that will appear on the left of a horizontally-split pane, or at the top of a
* vertically-split pane
* @param rightCmp
* the Component
that will appear on the right of a horizontally-split pane, or at the bottom of a
* vertically-split pane
* @exception IllegalArgumentException
* if orientation
is not one of: HORIZONTAL_SPLIT or VERTICAL_SPLIT
*/
public RollOverSplitPane(int splitOrientation, Component leftCmp, Component rightCmp) {
this(splitOrientation, false, leftCmp, rightCmp);
}
/**
* Creates a new RollOverSplitPane
with the specified orientation and redrawing style, and with the specified
* components.
*
* @param splitOrientation
* JSplitPane.HORIZONTAL_SPLIT
or JSplitPane.VERTICAL_SPLIT
* @param splitContinuousLayout
* a boolean, true for the components to redraw continuously as the divider changes position, false to wait until the
* divider position stops changing to redraw
* @param leftCmp
* the Component
that will appear on the left of a horizontally-split pane, or at the top of a
* vertically-split pane
* @param rightCmp
* the Component
that will appear on the right of a horizontally-split pane, or at the bottom of a
* vertically-split pane
* @exception IllegalArgumentException
* if orientation
is not one of HORIZONTAL_SPLIT or VERTICAL_SPLIT
*/
public RollOverSplitPane(int splitOrientation, boolean splitContinuousLayout, Component leftCmp, Component rightCmp) {
super(splitOrientation, splitContinuousLayout, leftCmp, rightCmp);
setBorder(null);
}
/**
* Use the give Settings
object to store/retrive the divider location.
*
* @param s
* the settings storage object
* @param baseKey
* a base key from wich the divider location setting key will be derived by adding ".dividerLocation".
* @param defaultDividerLocation
* initial value of divider location if not stored previously in settings.
*/
public void useSettings(Settings s, String baseKey, int defaultDividerLocation) {
sett = s;
dividerSettingKey = baseKey + ".dividerLocation";
if (sett != null) {
int dividerLocation = sett.getIntProperty(dividerSettingKey, defaultDividerLocation);
setDividerLocation(dividerLocation);
addPropertyChangeListener(JSplitPane.DIVIDER_LOCATION_PROPERTY, new PropertyChangeListener() {
public void propertyChange(PropertyChangeEvent evt) {
getSettings().setIntProperty(getDividerSettingKey(), getDividerLocation());
}
});
} else {
setDividerLocation(defaultDividerLocation);
}
}
public Settings getSettings() {
return sett;
}
String getDividerSettingKey() {
return dividerSettingKey;
}
/**
* Plug the custom UI delegate.
*/
@Override
public void updateUI() {
setUI(new RollOverSplitPaneUI());
setBorder(null);
revalidate();
}
static class RollOverSplitPaneUI extends BasicSplitPaneUI {
/**
* Creates the divider visible only when mouse is over.
*/
@Override
public BasicSplitPaneDivider createDefaultDivider() {
return new RollOverSplitPaneDivider(this);
}
}
static class RollOverSplitPaneDivider extends BasicSplitPaneDivider {
private boolean isRollOver;
RollOverSplitPaneDivider(BasicSplitPaneUI ui) {
super(ui);
addMouseListener(new MouseAdapter() {
@Override
public void mouseEntered(MouseEvent e) {
setRollover(true);
repaint();
}
@Override
public void mouseExited(MouseEvent e) {
setRollover(false);
repaint();
}
});
}
protected void setRollover(boolean rollover) {
isRollOver = rollover;
}
@Override
public void setBorder(Border border) {
// Never use border
}
@Override
public void paint(Graphics g) {
if (isRollOver) {
int x = getWidth() / 2;
int height = getHeight();
g.setColor(Color.gray);
g.drawLine(x - 1, 0, x - 1, height);
g.drawLine(x + 1, 0, x + 1, height);
g.setColor(Color.white);
g.drawLine(x, 0, x, height);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy