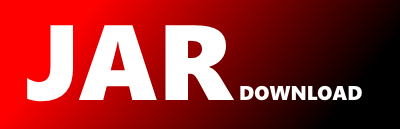
net.sf.sfac.gui.cmp.ToolbarToggleButton Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sfac-core Show documentation
Show all versions of sfac-core Show documentation
This project is the core part of the Swing Framework and Components (SFaC).
It contains the Swing framework classes and the graphical component classes.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2009 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.sfac.gui.cmp;
import java.awt.Insets;
import javax.swing.Action;
import javax.swing.ButtonModel;
import javax.swing.Icon;
import javax.swing.JToggleButton;
/**
* Toggle Button using only an icon and with roll-over effect on the border (as windows toolbar buttons). This button is
* designed to be used in a toolbar so you cannot set text on it and it cannot take focus.
*
* @author Olivier Berlanger
*/
@SuppressWarnings("serial")
public class ToolbarToggleButton extends JToggleButton {
/**
* Create a new ToolbarToggleButton
with the given icon.
*
* @param ico
* the button icon.
*/
public ToolbarToggleButton(Icon ico) {
super(ico);
setMargin(new Insets(2, 2, 2, 2));
super.setRolloverEnabled(true);
setRequestFocusEnabled(false);
}
/**
* Create a new ToolbarToggleButton
from the given action.
*
* @param act
* Action bound to this button.
* @param butModel
* the button model to be used by this button.
*/
public ToolbarToggleButton(Action act, ButtonModel butModel) {
super(act);
setModel(butModel);
// as we set a new model after the action we have to re-synchronize the 'setEnabled' flag
butModel.setEnabled(act.isEnabled());
setMargin(new Insets(2, 2, 2, 2));
super.setRolloverEnabled(true);
setRequestFocusEnabled(false);
}
/**
* Override the ancestor method so roll-over cannot be disabled by the look-and-feel.
*
* @param ignored
* ignored param.
*/
public void setRolloverEnabled(boolean ignored) {
super.setRolloverEnabled(true);
}
/**
* Override the ancestor method so a text cannot be set on this button.
*
* @param text
* ignored param.
*/
public void setText(String text) {
}
/**
* Override the ancestor method because a text cannot be set on this button.
*
* @return null
*/
public String getText() {
return null;
}
/**
* Override the ancestor method to return true only if roll-over is currently true.
*
* @return true iff the border should be painted.
*/
public boolean isBorderPainted() {
return (isEnabled() && getModel().isRollover()) || getModel().isSelected();
}
/**
* Return always false as a toolbar button cannot take the focus.
*
* @return false.
*/
public boolean isFocusable() {
return false;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy