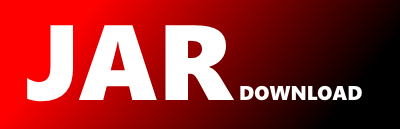
net.sf.sfac.gui.layout.SparseMatrix Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sfac-core Show documentation
Show all versions of sfac-core Show documentation
This project is the core part of the Swing Framework and Components (SFaC).
It contains the Swing framework classes and the graphical component classes.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2009 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.sfac.gui.layout;
/**
* Util class used by MultiBorderLayout to keep it's info. It's almost like an two dimensional ArrayList but you can get and set at
* any (positive) index without getting a arrayIndexOutOfBoundException.
*
* @author Olivier Berlanger
*/
class SparseMatrix {
/** Two dimensional array containing the objects of this matrix. */
private Object[][] matrix;
/** Number of rows in this matrix (some can be null). It always point just after the last non-null object. */
private int nbrRows;
/** Number of column in each row. It point just after the last non-null object for each row. */
private int[] nbrCols;
/** Maximum number of column in the matrix (maximum of nbrCols values). */
private int nbrMaxCol;
/**
* Create an empty SpareMatrix with an initial buffer size of 5x0 objects.
*/
protected SparseMatrix() {
matrix = new Object[5][0];
nbrCols = new int[5];
nbrRows = 0;
nbrMaxCol = 0;
}
/**
* Get the object at specified grid x & y position. If the index is out of the bounds of the buffer array, null is returned.
*
* @param gridY
* the row index of the object returned
* @param gridX
* the column index of the object returned
* @return the object at specified gridY/column or null if one index is out of bounds.
*/
protected Object get(int gridX, int gridY) {
if ((gridY < nbrRows) && (gridX < nbrCols[gridY])) return matrix[gridY][gridX];
return null;
}
/**
* Set the object at specified grid x & y position in the matrix. Setting a null value is like removing the existing object.
*
* @param gridY
* the row index of the new value
* @param gridX
* the column index of the new value
* @param element
* the new element value for the matrix
*/
protected void set(int gridX, int gridY, Object element) {
if (element == null) removeEl(gridX, gridY);
else addEl(gridX, gridY, element);
}
private void addEl(int gridX, int gridY, Object element) {
int len = matrix.length;
// check the row
if (gridY >= len) {
// enlarge the number of rows
int newLen = len * 2;
if (gridX >= newLen) newLen = gridX + 5;
Object[][] newMatrix = new Object[newLen][0];
System.arraycopy(matrix, 0, newMatrix, 0, len);
matrix = newMatrix;
// enlarge also the array keeping number of columns per row
int[] newNbrCols = new int[newLen];
System.arraycopy(nbrCols, 0, newNbrCols, 0, len);
nbrCols = newNbrCols;
}
Object[] rowArray = matrix[gridY];
int rowLen = rowArray.length;
if (gridX >= rowLen) {
// enlarge the selected row
int newLen = rowLen * 2;
if (gridX >= newLen) newLen = gridX + 5;
Object[] newRowArray = new Object[newLen];
System.arraycopy(rowArray, 0, newRowArray, 0, rowLen);
rowArray = newRowArray;
matrix[gridY] = newRowArray;
}
rowArray[gridX] = element;
if (gridY >= nbrRows) nbrRows = gridY + 1;
if (gridX >= nbrCols[gridY]) nbrCols[gridY] = gridX + 1;
if (gridX >= nbrMaxCol) nbrMaxCol = gridX + 1;
}
private void removeEl(int gridX, int gridY) {
if ((gridY < nbrRows) && (gridX < nbrCols[gridY])) {
boolean theMaxRow = (nbrMaxCol == nbrCols[gridY]);
Object[] rowArray = matrix[gridY];
rowArray[gridX] = null;
// trim the row size to last non-null object
while ((nbrCols[gridY] > 0) && (rowArray[nbrCols[gridY] - 1] == null))
nbrCols[gridY]--;
if (nbrCols[gridY] == 0) {
// trim the number of rows
while ((nbrRows > 0) && (nbrCols[nbrRows - 1] == 0))
nbrRows--;
}
// recalculate the number max of columns
if (theMaxRow && (nbrMaxCol != nbrCols[gridY])) {
nbrMaxCol = 0;
for (int i = 0; i < nbrRows; i++) {
if (nbrMaxCol < nbrCols[i]) nbrMaxCol = nbrCols[i];
}
}
}
}
protected int getNbrRows() {
return nbrRows;
}
protected int getNbrColumns(int gridY) {
return (gridY < nbrRows) ? nbrCols[gridY] : 0;
}
protected int getMaxNbrColumns() {
return nbrMaxCol;
}
/**
* Get the minimum index of a non-null object or -1 if list is empty.
*
* @return the minimum index of a non-null object or -1 if list is empty.
*/
protected int getMinRow() {
for (int i = 0; i < nbrRows; i++)
if (nbrCols[i] > 0) return i;
return -1;
}
// public void dump() {
// int cellDisplayLen=16 ;
// System.out.println(getClass().getName()+" "+nbrRows+" x "+nbrMaxCol) ;
// for(int i=0 ; i
© 2015 - 2024 Weber Informatics LLC | Privacy Policy