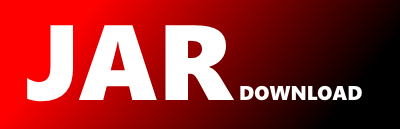
net.sf.sfac.gui.table.DragOutTableHeader Maven / Gradle / Ivy
Show all versions of sfac-core Show documentation
/*-------------------------------------------------------------------------
Copyright 2009 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.sfac.gui.table;
import javax.swing.table.JTableHeader;
import javax.swing.table.TableColumn;
import javax.swing.table.TableColumnModel;
/**
* Table header allowing to remove a column simply by dragging it out.
*
* @author Olivier Berlanger
*/
@SuppressWarnings("serial")
public class DragOutTableHeader extends JTableHeader {
private TableColumn draggedOut;
/**
* Sets the header's draggedColumn
to aColumn
.
*
* This method is overriden hide columns dragged out of the table. If the drag stops (setDraggedColumn(null) called) when the
* column is out of the table (draggedOut!=null) then it should be hidden by the HidingTableColumnModel. This behavior is
* available only if the associated TableColumnModel
is an instance of HidingTableColumnModel
*
* @param aColumn
* the new value for draggedColumn
* @see #setDraggedDistance
* @see HidingTableColumnModel
*/
@Override
public void setDraggedColumn(TableColumn aColumn) {
super.setDraggedColumn(aColumn);
if ((draggedOut != null) && (aColumn == null)) {
TableColumnModel cm = getColumnModel();
if (cm.getColumnCount() > 1) {
TableColumnModel tcm = getColumnModel();
if (tcm instanceof HidingTableColumnModel) {
HidingTableColumnModel htcm = (HidingTableColumnModel) tcm;
htcm.hideColumn(draggedOut);
}
}
draggedOut = null;
}
}
/**
* Sets the header's draggedDistance
to distance
.
*
* This method is overriden to check if the column is dragged out of the table. The column is dragged out of the table when the
* first column (index==0) or the last column (index==getColumnCount()-1) is dragged on a (negative or positive) distance
* greater than it's width. In this case this method remind the reference of the column dragged out of the table. If the drag
* stops (setDraggedColumn(null) called) when the column is still out of the table then it should be hidden by the
* HidingTableColumnModel.
*
* @param distance
* the distance dragged
* @see #setDraggedColumn
* @see HidingTableColumnModel
*/
@Override
public void setDraggedDistance(int distance) {
super.setDraggedDistance(distance);
TableColumn dragged = getDraggedColumn();
TableColumnModel cm = getColumnModel();
if (dragged != null) {
int draggedIndex = cm.getColumnIndex(dragged.getIdentifier());
if ((draggedIndex == 0) && ((-distance) > dragged.getWidth())) {
draggedOut = dragged;
} else if ((draggedIndex == (cm.getColumnCount() - 1)) && (distance > dragged.getWidth())) {
draggedOut = dragged;
} else {
if (distance > 0) {
draggedOut = null;
}
}
} else {
draggedOut = null;
}
}
}