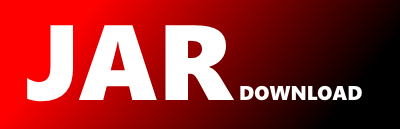
net.sf.sfac.gui.utils.TranslatingCellRenderer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sfac-core Show documentation
Show all versions of sfac-core Show documentation
This project is the core part of the Swing Framework and Components (SFaC).
It contains the Swing framework classes and the graphical component classes.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2009 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.sfac.gui.utils;
import java.awt.Color;
import java.awt.Component;
import javax.swing.DefaultListCellRenderer;
import javax.swing.JList;
import javax.swing.JTable;
import javax.swing.UIManager;
import javax.swing.border.Border;
import javax.swing.table.TableCellRenderer;
import net.sf.sfac.lang.LanguageSupport;
/**
* ListCellRenderer translating the displayed (String) values using the LanguageSupport
.
*/
@SuppressWarnings("serial")
public class TranslatingCellRenderer extends DefaultListCellRenderer implements TableCellRenderer {
public TranslatingCellRenderer() {
}
@Override
public Component getListCellRendererComponent(JList> list, Object value, int index, boolean isSelected, boolean cellHasFocus) {
super.getListCellRendererComponent(list, value, index, isSelected, cellHasFocus);
setValue(value);
return this;
}
protected void setValue(Object value) {
if (value != null) {
String defaultValue = value.toString();
String key = defaultValue;
if (value instanceof Enum) {
key = value.getClass().getSimpleName() + "." + ((Enum>) value).name();
}
setText(LanguageSupport.getOptionalLocalizedString(key, defaultValue));
} else {
setText("");
}
}
// ------------- Code copied from DefaultTableCellRenderer
private Color unselectedForeground;
private Color unselectedBackground;
@Override
public void setForeground(Color c) {
super.setForeground(c);
unselectedForeground = c;
}
@Override
public void setBackground(Color c) {
super.setBackground(c);
unselectedBackground = c;
}
@Override
public void updateUI() {
super.updateUI();
setForeground(null);
setBackground(null);
}
/**
*
* Returns the default table cell renderer.
*
* @param table
* the JTable
* @param value
* the value to assign to the cell at [row, column]
* @param isSelected
* true if cell is selected
* @param hasFocus
* true if cell has focus
* @param row
* the row of the cell to render
* @param column
* the column of the cell to render
* @return the default table cell renderer
*/
public Component getTableCellRendererComponent(JTable table, Object value, boolean isSelected, boolean hasFocus, int row, int column) {
if (isSelected) {
super.setForeground(table.getSelectionForeground());
super.setBackground(table.getSelectionBackground());
} else {
super.setForeground((unselectedForeground != null) ? unselectedForeground : table.getForeground());
super.setBackground((unselectedBackground != null) ? unselectedBackground : table.getBackground());
}
setFont(table.getFont());
if (hasFocus) {
Border border = null;
if (isSelected) {
border = UIManager.getBorder("Table.focusSelectedCellHighlightBorder");
}
if (border == null) {
border = UIManager.getBorder("Table.focusCellHighlightBorder");
}
setBorder(border);
if (!isSelected && table.isCellEditable(row, column)) {
Color col;
col = UIManager.getColor("Table.focusCellForeground");
if (col != null) {
super.setForeground(col);
}
col = UIManager.getColor("Table.focusCellBackground");
if (col != null) {
super.setBackground(col);
}
}
} else {
setBorder(noFocusBorder);
}
setValue(value);
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy