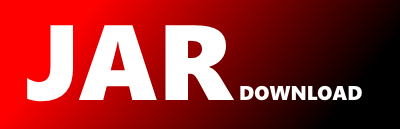
net.sf.sfac.editor.EditorConfig Maven / Gradle / Ivy
Show all versions of sfac-utils Show documentation
/*-------------------------------------------------------------------------
Copyright 2009 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.sfac.editor;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
/**
* Annotation used by the GenericObjectEditor to build the Editor GUI.
* Annotate a class like this:
*
*
* @EditorConfig(name="My Object", editor="org.mycompany.MyEditor")
* public class ObjectToEdit() {
* ...
* }
*
*
* to configure the way the object will appear in a generated form GUI.
* Annotate a getter (method without parameter or a method with an integer index for indexed getters) like this:
*
*
* @EditorConfig(name="My field", index=0, editor="org.mycompany.MyRenderer")
* public String getSomething() {
* ...
* }
*
*
* and the return value of the method will be edited in a generated form GUI.
* The annotated method is taken as the getter for the field to edit. The editor will try to find corresponding setter. If no setter
* is found, the field will be read-only.
* See methods javadoc for meaning of annotation attributes.
*
* @author Olivier Berlanger
*/
@Retention(RetentionPolicy.RUNTIME)
public @interface EditorConfig {
public static final String UNDEFINED = "<[undefined]>";
/**
* Gives a displayable name for the edited field/type. If omitted or empty string, no label is displayed.
* The way this label is used in the resulting GUI depends on the ObjectEditor used, but in usually:
*
* - When used in a type annotation (of a main edited object) it is used to display the form title.
*
- When used on a getter method, it is used as label in front of the editor component
*
*
* The given string can reference an attribute (with "{@...}") or a method (with "{$...}") of the edited object. In this
* case, the specified method is called on the edited object to get the label.
* See net.sf.sfac.gui.editor.access.ModelAccessFactory class javadoc for more info.
*
* Note: If the LanguageSupport is configured, the given label is used as bundle key.
*/
public String label() default UNDEFINED;
/**
* Gives a displayable description for the edited field/type. If omitted or empty string, no description is displayed.
* The way this description is used in the resulting GUI depends on the ObjectEditor used, but in usually:
*
* - When used in a type annotation (of a main edited object) it is used to display a sub-title just below the form title.
*
- When used on a getter method, it is used as tooltip on the field label and widget.
*
*
* The given string can reference an attribute (with "{@...}") or a method (with "{$...}") of the edited object. In this
* case, the specified method is called on the edited object to get the description.
* See net.sf.sfac.gui.editor.access.ModelAccessFactory class javadoc for more info.
*
* Note: If the LanguageSupport is configured, the given description is used as bundle key.
*/
public String description() default UNDEFINED;
/**
* Gives the preferred field editor position. The field editors are sorted on this index (if present) and added on the generated
* GUI in this order. The editor of fields not providing indexes are processed after, more or less in the order of definition in
* the owning code.
*/
public int index() default -1;
/**
* Full class name of the editor ObjectEditor to use.
*
* - When used in a class annotation, it means that EditorFactory will use the provided ObjectEditor as default editor for
* this class.
*
- When used on a getter method, it is used to display the field in the editor generated (by GenericObjectEditor) for the
* owner type.
*
*/
public String editor() default UNDEFINED;
/**
* Set the editor the editable or not (the isEditable() in swing components).
* By default, an editor is editable if its parent is editable, if a model is set and if there is a setter defined allowing it
* to update the model.
* A static visibility ("true" or "false") is not really useful, so for this annotation value, you should preferably use the
* method/attribute reference explained below.
*
* The given string can reference an attribute (with "{@...}") or a method (with "{$...}") of the edited object. In this
* case, the specified method is called on the edited object to get the editable attribute.
* See net.sf.sfac.gui.editor.access.ModelAccessFactory class javadoc for more info.
*
*/
public String editable() default UNDEFINED;
/**
* Set the editor the enabled or not (the isEnabled() in swing components).
* By default, an editor is editable if its parent is editable, if a model is set and if there is a setter defined allowing it
* to update the model.
* A static visibility ("true" or "false") is not really useful, so for this annotation value, you should preferably use the
* method/attribute reference explained below.
*
* The given string can reference an attribute (with "{@...}") or a method (with "{$...}") of the edited object. In this
* case, the specified method is called on the edited object to get the enabled attribute.
* See net.sf.sfac.gui.editor.access.ModelAccessFactory class javadoc for more info.
*
*/
public String enabled() default UNDEFINED;
/**
* Definition editor the visibility.
* By default, an editor is visible if its parent is visible (and root are visible by default).
* A static visibility ("true" or "false") is not really useful, so for this annotation value, you should preferably use the
* method/attribute reference explained below.
*
* The given string can reference an attribute (with "{@...}") or a method (with "{$...}") of the edited object. In this
* case, the specified method is called on the edited object to get the visibility.
* See net.sf.sfac.gui.editor.access.ModelAccessFactory class javadoc for more info.
*
*/
public String visibility() default UNDEFINED;
/**
* Type of editor: this parameter can be interpreted differently (or ignored) by each editor.
*
* For example, with the StringEditor, you can use type="line" (the default) or type="text" to have a single-line (i.e. *
* JTextField) or a multi-line (i.e. JTextArea) string editor.
*
*/
public String type() default UNDEFINED;
/**
* Key of a list to use: this parameter can be interpreted differently (or ignored) by each editor.
* The actual object containing the list is provided by the editor context (method getContextObject(key)
)
*/
public String list() default UNDEFINED;
/**
* Id of the Tab where to display the field: this parameter can be interpreted differently (or ignored) by each editor.
*/
public String tab() default UNDEFINED;
/**
* List of properties: The returned array must contain an odd number of strings, each time the property id followed by the
* property value. This parameter can be interpreted differently (or ignored) by each editor.
*/
public String[] properties() default {};
}