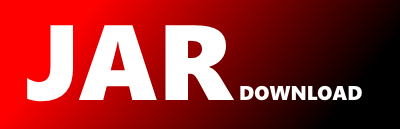
net.sf.sfac.file.FileUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sfac-utils Show documentation
Show all versions of sfac-utils Show documentation
This project is the model side of the Swing Framework and Components (SFaC).
If your doing a clean separation between model (or business) and view (or GUI or rendering) parts of your application,
(like in the MVC pattern), then the only classes of SFaC your model can access are in this project.
On the other hand, the classes in sfac-core project are GUI-specific and should not be known by your model.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2009 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.sfac.file;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.Reader;
import java.io.Writer;
/**
* Common file operations.
*/
public class FileUtils {
public static void copyFile(File source, File target) throws IOException {
if (!source.exists()) throw new IOException("Source must exists: " + source);
if (!source.isFile()) throw new IOException("Source must be a file: " + source);
File targetDir = target.getParentFile();
if (targetDir != null) targetDir.mkdirs();
FileInputStream is = new FileInputStream(source);
FileOutputStream os = new FileOutputStream(target);
copyFile(is, os);
is.close();
os.close();
}
public static void copyFile(InputStream in, OutputStream out) throws IOException {
byte[] buffer = new byte[1024];
int len;
while ((len = in.read(buffer)) > 0) {
out.write(buffer, 0, len);
}
out.flush();
}
public static void copyFile(Reader in, Writer out) throws IOException {
char[] buffer = new char[1024];
int len;
while ((len = in.read(buffer)) > 0) {
out.write(buffer, 0, len);
}
out.flush();
}
public static boolean areFilesEqual(File f1, File f2) throws IOException {
if (!f1.exists()) throw new IOException("Source must exists: " + f1);
if (!f2.isFile()) throw new IOException("Source must be a file: " + f2);
FileInputStream in1 = new FileInputStream(f1);
FileInputStream in2 = new FileInputStream(f2);
boolean equals = areFilesEqual(in1, in2);
in1.close();
in2.close();
return equals;
}
public static boolean areFilesEqual(InputStream in1, InputStream in2) throws IOException {
BufferedInputStream bi1 = (in1 instanceof BufferedInputStream) ? (BufferedInputStream) in1 : new BufferedInputStream(in1);
BufferedInputStream bi2 = (in2 instanceof BufferedInputStream) ? (BufferedInputStream) in2 : new BufferedInputStream(in2);
int ch1 = bi1.read();
int ch2 = bi2.read();
while ((ch1 >= 0) && (ch2 >= 0) && (ch1 == ch2)) {
ch1 = bi1.read();
ch2 = bi2.read();
}
return (ch1 < 0) && (ch2 < 0);
}
public static void moveFile(File source, File target) throws IOException {
if (!source.exists()) throw new IOException("Source must exists: " + source);
if (target.exists()) throw new IOException("Target already exists: " + target);
ensureParentDirectoryExists(target);
boolean success = source.renameTo(target);
if (!success) throw new IOException("Failed to move " + source + " to " + target);
}
public static void ensureParentDirectoryExists(File f) throws IOException {
File parentDir = f.getAbsoluteFile().getParentFile();
if ((parentDir != null) && !parentDir.exists()) {
boolean success = parentDir.mkdirs();
if (!success) throw new IOException("Failed to create parent directory " + parentDir);
}
}
public static void deleteFile(File fil) throws IOException {
if (!fil.exists()) throw new IOException("File must exists: " + fil);
boolean success = fil.delete();
if (!success) throw new IOException("Failed to delete " + fil);
}
public static void renameToBackup(File source) throws IOException {
if (!source.exists()) throw new IOException("Source must exists: " + source);
File dir = source.getParentFile();
String sourceName = source.getName();
String ext = FilePathUtils.getExtension(sourceName);
String nameWithoutExt = (ext == null) ? sourceName : sourceName.substring(0, sourceName.length() - (ext.length() + 1));
int i = 1;
while (true) {
StringBuffer sb = new StringBuffer().append(nameWithoutExt).append("_").append(i);
if (ext != null) sb.append(".").append(ext);
String newName = sb.toString();
File renamed = (dir == null) ? new File(newName) : new File(dir, newName);
if (!renamed.exists()) {
moveFile(source, renamed);
break;
}
i++;
}
}
public static String removeReservedFileCharacters(String src) {
if (src == null) return null;
int len = src.length();
if (len == 0) return "";
// check if file need changes
boolean needChange = isReservedFileBoundCharacters(src.charAt(0));
if (!needChange) needChange = isReservedFileBoundCharacters(src.charAt(len - 1));
for (int i = 1; (i < len) && !needChange; i++) {
needChange = isReservedFileCharacters(src.charAt(i));
}
if (needChange) {
// Do the transformation
int startIndex = 0;
while ((startIndex < len) && isReservedFileBoundCharacters(src.charAt(startIndex))) {
startIndex++;
}
StringBuilder sb = new StringBuilder();
for (int i = startIndex; i < len; i++) {
if (!isReservedFileCharacters(src.charAt(i))) {
sb.append(src.charAt(i));
}
}
while ((sb.length() > 0) && isReservedFileBoundCharacters(sb.charAt(sb.length() - 1))) {
sb.deleteCharAt(sb.length() - 1);
}
return sb.toString();
} else {
return src;
}
}
public static boolean isReservedFileCharacters(char ch) {
if (ch == '/') return true;
if (ch == '\\') return true;
if (ch == '*') return true;
if (ch == '?') return true;
if (ch == '"') return true;
if (ch == '\n') return true;
if (ch == '\t') return true;
if (ch == '>') return true;
if (ch == '<') return true;
if (ch == '|') return true;
if (ch == ':') return true;
return false;
}
public static boolean isReservedFileBoundCharacters(char ch) {
if (isReservedFileCharacters(ch)) return true;
if (ch == '.') return true;
if (ch == ' ') return true;
return false;
}
public static InputStream getUncloseableStream(InputStream is) {
return new UncloseableStream(is);
}
static class UncloseableStream extends InputStream {
private InputStream wrapped;
UncloseableStream(InputStream wrappedStream) {
wrapped = wrappedStream;
}
@Override
public void close() throws IOException {
// do nothing
}
@Override
public int read() throws IOException {
return wrapped.read();
}
@Override
public int read(byte[] b) throws IOException {
return wrapped.read(b);
}
@Override
public int read(byte[] b, int off, int len) throws IOException {
return wrapped.read(b, off, len);
}
@Override
public long skip(long n) throws IOException {
return wrapped.skip(n);
}
@Override
public int available() throws IOException {
return wrapped.available();
}
@Override
public void mark(int readlimit) {
wrapped.mark(readlimit);
}
@Override
public void reset() throws IOException {
wrapped.reset();
}
@Override
public boolean markSupported() {
return wrapped.markSupported();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy