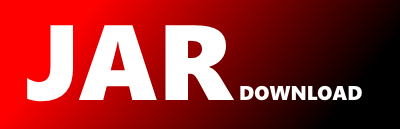
net.sf.sfac.file.LineReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sfac-utils Show documentation
Show all versions of sfac-utils Show documentation
This project is the model side of the Swing Framework and Components (SFaC).
If your doing a clean separation between model (or business) and view (or GUI or rendering) parts of your application,
(like in the MVC pattern), then the only classes of SFaC your model can access are in this project.
On the other hand, the classes in sfac-core project are GUI-specific and should not be known by your model.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2009 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.sfac.file;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.URL;
/**
* Object wrapping a BufferedReader adding line peeking and counting.
* It can:
*
* - Peek line (=getting content of next line without getting it out).
*
- Count lines.
*
- Remind the source name (file name, resource name, url)
*
*/
public class LineReader {
private static String readerEncoding = "ISO8859_1";
private BufferedReader br;
private String sourceName;
private int lineIndex;
private String line;
public static String getReaderEncoding() {
return readerEncoding;
}
public static void setReaderEncoding(String newReaderEncoding) {
readerEncoding = newReaderEncoding;
}
public LineReader(File src) throws IOException {
sourceName = src.getAbsolutePath();
setup(new BufferedReader(new InputStreamReader(new FileInputStream(src), readerEncoding)));
}
public LineReader(URL src) throws IOException {
sourceName = src.toExternalForm();
setup(new BufferedReader(new InputStreamReader(src.openStream(), readerEncoding)));
}
public LineReader(Class> loader, String resourceName) throws IOException {
URL resourceURL = loader.getResource(resourceName);
sourceName = resourceURL.toExternalForm();
setup(new BufferedReader(new InputStreamReader(resourceURL.openStream(), readerEncoding)));
}
public LineReader(BufferedReader reader) throws IOException {
sourceName = "input stream";
setup(reader);
}
private void setup(BufferedReader reader) {
br = reader;
lineIndex = 0;
}
public String peekLine() throws IOException {
if (lineIndex <= 0) line = readLineImpl();
return line;
}
/**
* Equivalent to calling readLine()
and then peekLine()
.
*/
public String peekNextLine() throws IOException {
readLine();
return line;
}
public String readLine() throws IOException {
String returnedLine = line;
line = readLineImpl();
return returnedLine;
}
protected String readLineImpl() throws IOException {
lineIndex++;
return br.readLine();
}
public String getSourceName() {
return sourceName;
}
/**
* Returns the index of the line returned if you currently call the peekLine() method.
*/
public int getLineIndex() {
return lineIndex;
}
public void close() throws IOException {
br.close();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy