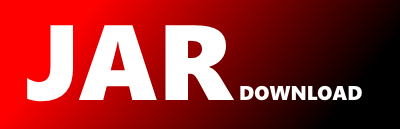
net.sf.sfac.setting.Settings Maven / Gradle / Ivy
Show all versions of sfac-utils Show documentation
/*-------------------------------------------------------------------------
Copyright 2009 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.sfac.setting;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Rectangle;
import java.beans.PropertyChangeListener;
import java.io.File;
import java.io.IOException;
import java.util.Iterator;
import net.sf.sfac.file.FilePathUtils;
import net.sf.sfac.file.InvalidPathException;
/**
* Interface used to store the settings of an application.
* It provides a kind of service able to store some object values and retrieve them using a string key. It also provide
* PropertyChangeListener
support to listen to settings changes (if needed).
*
* This settings store allows to convert the file properties (strings representing file paths) from absolute to relative (and
* vice-versa). The idea is that the file settings values are always stored relatively to the base path (and always get as
* absolute). So if you move or copy your application to another directory (and if the base path is correctly updated by the
* application) every file property will be resolved to the correct location.
*
*
* @author Olivier Berlanger
*/
public interface Settings {
/**
* Get the FilePathUtils used by this settings object.
* This FilePathUtils is used to convert between absolute and relative file path settings. (So it holds the default base path).
*
* @return The FilePathUtils used by this settings object.
*/
public FilePathUtils getFilePathUtils();
/**
* Get the path to the property file used to store the settings.
* The returned path is absolute.
*
* @return The path to the property file used to store the settings.
*/
public String getPropertyFilePath();
/**
* Ask the settings implementation to load the settings values. (the real effect of it will depend of the concrete
* Settings
implementation.)
*
* @exception IOException
* If something prevents the settings to be loaded.
*/
public void load() throws IOException;
/**
* Ask the settings implementation to save the settings values. (the real effect of it will depend of the concrete
* Settings
implementation.)
*
* @exception IOException
* If something prevents the settings to be saved.
*/
public void save() throws IOException;
/**
* Set a new property file to store the settings. The given property file will be set as new reference for paths.
*
* @param fil
* The new properties file.
*/
public void setPropertyFile(File fil);
/**
* Save all the settings to a new property file. If the file don't exists, it is created, if the file exists it is overriden
* without warning. The given property file will be set as new reference for paths.
*
* @param fil
* The new properties file.
* @exception IOException
* If something prevents the settings to be saved.
*/
public void saveAs(File fil) throws IOException;
/**
* Check if there is a property value defined for the given key.
*
* @param key
* Key for the value.
* @return True iff there is a property value defined for the given key.
*/
public boolean containsProperty(String key);
/**
* Remove the property.
* If the property was not defined, it does nothing.
*
* @param key
* Key of the property to remove.
*/
public void removeProperty(String key);
/**
* Return an iterator for all the defined keys.
*
* @return an iterator for all the defined keys.
*/
public Iterator getKeys();
/**
* Get a String property value.
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key.
* @return The value associated to the given key (or default value if no value was found).
*/
public String getStringProperty(String key, String defaultValue);
/**
* Set a new value for a String property.
* This method will fire an propertyChangeEvent to all registered listeners if the value set is different than the current
* value.
*
* @param key
* Key for the value.
* @param value
* New value to be associated with the key.
*/
public void setStringProperty(String key, String value);
/**
* Get a String property value to be used as a password.
* The password will be obfuscated (obfuscation is much lighter that encryption, so don't store very sensitive password using
* this mechanism).
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key.
* @return The value associated to the given key (or default value if no value was found).
*/
public String getPasswordProperty(String key, String defaultValue);
/**
* Set a new value for a String property.
* This method will fire an propertyChangeEvent to all registered listeners if the value set is different than the current
* value. The password will be obfuscated (obfuscation is much lighter that encryption, so don't store very sensitive password
* using this mechanism).
*
* @param key
* Key for the value.
* @param value
* New value to be associated with the key.
*/
public void setPasswordProperty(String key, String value);
/**
* Get a String array property value.
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key.
* @return The value associated to the given key (or default value if no value was found).
*/
public String[] getStringArrayProperty(String key, String[] defaultValue);
/**
* Set a new value for a String array property.
* This method will fire an propertyChangeEvent to all registered listeners if the value set is different than the current
* value.
*
* @param key
* Key for the value.
* @param value
* New value to be associated with the key.
*/
public void setStringArrayProperty(String key, String[] value);
/**
* Get a int property value.
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key.
* @return The value associated to the given key (or default value if no value was found).
*/
public int getIntProperty(String key, int defaultValue);
/**
* Set a new value for a int property.
* This method will fire an propertyChangeEvent to all registered listeners if the value set is different than the current
* value.
*
* @param key
* Key for the value.
* @param value
* New value to be associated with the key.
*/
public void setIntProperty(String key, int value);
/**
* Get a Integer property value.
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key.
* @return The value associated to the given key (or default value if no value was found).
*/
public Integer getIntegerProperty(String key, Integer defaultValue);
/**
* Set a new value for a Integer property.
* This method will fire an propertyChangeEvent to all registered listeners if the value set is different than the current
* value.
*
* @param key
* Key for the value.
* @param value
* New value to be associated with the key.
*/
public void setIntegerProperty(String key, Integer value);
/**
* Get a double property value.
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key.
* @return The value associated to the given key (or default value if no value was found).
*/
public double getDoubleProperty(String key, double defaultValue);
/**
* Set a new value for a double property.
* This method will fire an propertyChangeEvent to all registered listeners if the value set is different than the current
* value.
*
* @param key
* Key for the value.
* @param value
* New value to be associated with the key.
*/
public void setDoubleProperty(String key, double value);
/**
* Get a boolean property value.
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key.
* @return The value associated to the given key (or default value if no value was found).
*/
public boolean getBooleanProperty(String key, boolean defaultValue);
/**
* Set a new value for a boolean property.
* This method will fire an propertyChangeEvent to all registered listeners if the value set is different than the current
* value.
*
* @param key
* Key for the value.
* @param value
* New value to be associated with the key.
*/
public void setBooleanProperty(String key, boolean value);
/**
* Get a Boolean property value.
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key.
* @return The value associated to the given key (or default value if no value was found).
*/
public Boolean getBooleanProperty(String key, Boolean defaultValue);
/**
* Set a new value for a Boolean property.
* This method will fire an propertyChangeEvent to all registered listeners if the value set is different than the current
* value.
*
* @param key
* Key for the value.
* @param value
* New value to be associated with the key.
*/
public void setBooleanProperty(String key, Boolean value);
/**
* Get a int array property value.
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key.
* @return The value associated to the given key (or default value if no value was found).
*/
public int[] getIntArrayProperty(String key, int[] defaultValue);
/**
* Set a new value for a int array property.
* This method will fire an propertyChangeEvent to all registered listeners if the value set is different than the current
* value.
*
* @param key
* Key for the value.
* @param value
* New value to be associated with the key.
*/
public void setIntArrayProperty(String key, int[] value);
/**
* Get a Rectangle property value.
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key.
* @return The value associated to the given key (or default value if no value was found).
*/
public Rectangle getRectangleProperty(String key, Rectangle defaultValue);
/**
* Set a new value for a Rectangle property.
* This method will fire an propertyChangeEvent to all registered listeners if the value set is different than the current
* value.
*
* @param key
* Key for the value.
* @param value
* New value to be associated with the key.
*/
public void setRectangleProperty(String key, Rectangle value);
/**
* Get a Dimension property value.
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key.
* @return The value associated to the given key (or default value if no value was found).
*/
public Dimension getDimensionProperty(String key, Dimension defaultValue);
/**
* Set a new value for a Dimension property.
* This method will fire an propertyChangeEvent to all registered listeners if the value set is different than the current
* value.
*
* @param key
* Key for the value.
* @param value
* New value to be associated with the key.
*/
public void setDimensionProperty(String key, Dimension value);
/**
* Get a Color property value.
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key.
* @return The value associated to the given key (or default value if no value was found).
*/
public Color getColorProperty(String key, Color defaultValue);
/**
* Set a new value for a Color property.
* This method will fire an propertyChangeEvent to all registered listeners if the value set is different than the current
* value.
*
* @param key
* Key for the value.
* @param value
* New value to be associated with the key.
*/
public void setColorProperty(String key, Color value);
/**
* Get a enumerated property value.
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key.
* Note: this default value cannot be null, it must be a value of the enumeration.
* @return The value associated to the given key (or default value if no value was found).
*/
public > T getEnumProperty(String key, T defaultValue);
/**
* Set a new value for a enumerated property.
* This method will fire an propertyChangeEvent to all registered listeners if the value set is different than the current
* value.
*
* @param key
* Key for the value.
* @param value
* New value to be associated with the key.
*/
public > void setEnumProperty(String key, T value);
/**
* Get a absolute File or Directory property value.
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key. (if used, the default value will be processed to
* ensure it's absolute)
* @return The value associated to the given key (or default value if no value was found).
*/
public String getFileProperty(String key, String defaultValue);
/**
* Get a File or Directory property value.
*
* @param key
* Key for the value.
* @param defaultValue
* Default value to be returned if no value is found for the key. (if used, the default value will be processed to
* ensure it's relative or absolute as requested)
* @param absolute
* tells if the returned file should be absolute or relative to the settings base directory.
* @return The absolute or relative file path associated to the given key (or default value if no value was found).
*/
public String getFileProperty(String key, String defaultValue, boolean absolute);
/**
* Set a new value for a File or Directory property.
* The specified file can be absolute or relative. If a relative file is specifed, it's taken as relative to the base path of
* this settings.
* This method will fire an propertyChangeEvent to all registered listeners if the value set is different than the current
* value.
*
* @param key
* Key for the value.
* @param value
* New value to be associated with the key.
* @exception InvalidPathException
* if the file set is invalid.
*/
public void setFileProperty(String key, String value) throws InvalidPathException;
// -------------------- Internal management property getter/setter ----------------------------------------
/**
* Get a property as stored internally by the map (as string).
* This is used to act on the key/values independently actual type of the value (that can be not known yet). The actual format
* of the string object depends on the implementation.
*
* @param key
* Key for the property value.
* @return The property value associated to the given key using the internal string representation. returns null if the property
* is not defined.
*/
public String getPropertyAsInternalString(String key);
/**
* Set a property value using its internal string representation. Setting a null value will remove the property.
*
* @param key
* Key for the property value.
* @param value
* The new value of the property to associate to the given key.
*/
public void setPropertyAsInternalString(String key, String value);
/**
* Get a property of type defined by the helper. This method is useful when you want to extend this class to new types (by
* creating new TypeHelper
classes). For properties using a pre-defined type you should use the type-specific
* method.
*
* Note that if you create a new data type the same type helper INSTANCE should be used in all set and get for this type.
*
*
* @param key
* Key for the property value.
* @param helper
* Helper identifying the value type and knowing how to convert between string and the requested type. If the helper
* is null, a string value is returned.
* @return The property value associated to the given key and of given type.
*/
public T getProperty(String key, TypeHelper helper, T defaultValue);
/**
* Set a property of type defined by the helper. This method is usefull when you want to extend this class to new types (by
* creating new TypeHelper
classes). For properties using a pre-defined type you should use the type-specific
* method.
* Giving a null value removes the property (values and keys are removed).
* If the new value is different than the previous one, a property change event is fired.
*
* Note that if you create a new data type the same type helper INSTANCE should be used in all set and get for this type.
*
*
* @param key
* Key for the property value.
* @param helper
* Helper identifying the value type and knowing how to convert between string and the requested type. If the given
* helper is null, the property will be stored as string (with basic toString
conversion in the backing
* properties map). When the given helper is null, no propertyChange event is generated.
* @param value
* The new value of the property to associate to the given key.
*/
public void setProperty(String key, TypeHelper helper, T value);
// -------------------- Property change listener management ----------------------------------------
/**
* Add a PropertyChangeListener for a specific setting.
*
* @param settingKey
* The key of the setting to listen on.
* @param listener
* The PropertyChangeListener to be added
*/
public void addPropertyChangeListener(String settingKey, PropertyChangeListener listener);
/**
* Remove a PropertyChangeListener for a specific setting.
*
* @param settingKey
* The key of the setting that was listened on.
* @param listener
* The PropertyChangeListener to be removed
*/
public void removePropertyChangeListener(String settingKey, PropertyChangeListener listener);
}