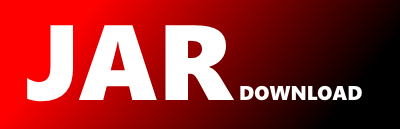
net.sf.sfac.setting.SubSettingsProxy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sfac-utils Show documentation
Show all versions of sfac-utils Show documentation
This project is the model side of the Swing Framework and Components (SFaC).
If your doing a clean separation between model (or business) and view (or GUI or rendering) parts of your application,
(like in the MVC pattern), then the only classes of SFaC your model can access are in this project.
On the other hand, the classes in sfac-core project are GUI-specific and should not be known by your model.
The newest version!
/*-------------------------------------------------------------------------
Copyright 2009 Olivier Berlanger
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
-------------------------------------------------------------------------*/
package net.sf.sfac.setting;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Rectangle;
import java.beans.PropertyChangeListener;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import net.sf.sfac.file.FilePathUtils;
import net.sf.sfac.file.InvalidPathException;
/**
* Implementation of the Settings
interface, allowing to present a subset of the settings as another settings object.
* A prefix is added by this proxy to all the keys before to put them in the parent settings. It allows to easily make profiles (=
* different set of identical settings saved in the same global settings file).
*
* @author Olivier Berlanger
*/
public class SubSettingsProxy implements Settings, Comparable {
public static final String SUB_SETTING_NAME = "subSettingName";
private Settings parent;
private String baseKey;
private int index;
/**
* Construct a new settings implementation bound to a given property file.
*
* @param propertyFile
* File where the properties are stored.
* @param descr
* Description of properties written as header in properties file.
*/
public SubSettingsProxy(Settings parentSettings, String prefix) {
this(parentSettings, prefix, -1);
}
/**
* Construct a new indexed settings implementation bound to a given property file.
* Note the index is just added in the key.
*
* @param propertyFile
* File where the properties are stored.
* @param descr
* Description of properties written as header in properties file.
* @param i
* the index of this SubSettingsProxy (if they are indexed). -1 for non-indexed.
*/
public SubSettingsProxy(Settings parentSettings, String prefix, int i) {
parent = parentSettings;
index = i;
if (index >= 0) baseKey = prefix + "_" + index + ".";
else baseKey = prefix + ".";
}
public int getIndex() {
return index;
}
/**
* Remove all the settings corresponding to this proxy from the parent settings.
*
*/
public void clear() {
List keysToRemove = new ArrayList();
for (Iterator iter = parent.getKeys(); iter.hasNext();) {
String key = iter.next();
if (key.startsWith(baseKey)) {
keysToRemove.add(key);
}
}
for (String key : keysToRemove) {
parent.removeProperty(key);
}
}
/**
* Copy all the values of the other settings into this one.
* This method doesn't care about the current content of this settings, existing values are simply overwritten. If you don't
* want to keep the current content, don't forget to do a clear()
before copying.
*
* @param other
* the other settings containing the values to copy.
*/
public void copyValues(Settings other) {
if (other == parent) throw new IllegalArgumentException("Cannot copy values from parent");
// put the keys in a temporary list to avoid concurrent modifications because two SubSettingProxy can share the same
// map.
List keys = new ArrayList();
for (Iterator iter = other.getKeys(); iter.hasNext();) {
keys.add(iter.next());
}
// do the actual copy
for (String key : keys) {
if (!SubSettingsProxy.SUB_SETTING_NAME.equals(key)) {
String value = other.getPropertyAsInternalString(key);
setPropertyAsInternalString(key, value);
}
}
}
public void dump() {
System.out.println("----- Sub settings: " + baseKey + ", index=" + index);
for (Iterator iter = getKeys(); iter.hasNext();) {
String key = iter.next();
System.out.println(" * " + key + " = " + getPropertyAsInternalString(key));
}
}
private String getFullKey(String key) {
return baseKey + key;
}
String getBaseKey() {
return baseKey;
}
@Override
public String toString() {
return getName();
}
public String getName() {
return getStringProperty(SUB_SETTING_NAME, "?");
}
public void setName(String newName) {
setStringProperty(SUB_SETTING_NAME, newName);
}
public int compareTo(SubSettingsProxy other) {
return getName().compareTo(other.getName());
}
@Override
public boolean equals(Object other) {
if (other == null) return false;
if (getClass() != other.getClass()) return false;
return getName().equals(((SubSettingsProxy) other).getName());
}
@Override
public int hashCode() {
return getName().hashCode();
}
// -------------------------------------- Delegation to settings ----------------------------------------------------
public String getPropertyAsInternalString(String key) {
return parent.getPropertyAsInternalString(getFullKey(key));
}
public void setPropertyAsInternalString(String key, String value) {
parent.setPropertyAsInternalString(getFullKey(key), value);
}
public T getProperty(String key, TypeHelper helper, T defaultValue) {
return parent.getProperty(getFullKey(key), helper, defaultValue);
}
public void setProperty(String key, TypeHelper helper, T value) {
parent.setProperty(getFullKey(key), helper, value);
}
public String getPropertyFilePath() {
return parent.getPropertyFilePath();
}
public FilePathUtils getFilePathUtils() {
return parent.getFilePathUtils();
}
public void load() throws IOException {
parent.load();
}
public void save() throws IOException {
parent.save();
}
public void setPropertyFile(File fil) {
parent.setPropertyFile(fil);
}
public void saveAs(File fil) throws IOException {
parent.saveAs(fil);
}
public boolean containsProperty(String key) {
return parent.containsProperty(getFullKey(key));
}
public String getStringProperty(String key, String defaultValue) {
return parent.getStringProperty(getFullKey(key), defaultValue);
}
public void setStringProperty(String key, String value) {
parent.setStringProperty(getFullKey(key), value);
}
public String getPasswordProperty(String key, String defaultValue) {
return parent.getPasswordProperty(getFullKey(key), defaultValue);
}
public void setPasswordProperty(String key, String value) {
parent.setPasswordProperty(getFullKey(key), value);
}
public String[] getStringArrayProperty(String key, String[] defaultValue) {
return parent.getStringArrayProperty(getFullKey(key), defaultValue);
}
public void setStringArrayProperty(String key, String[] value) {
parent.setStringArrayProperty(getFullKey(key), value);
}
public int getIntProperty(String key, int defaultValue) {
return parent.getIntProperty(getFullKey(key), defaultValue);
}
public void setIntProperty(String key, int value) {
parent.setIntProperty(getFullKey(key), value);
}
public Integer getIntegerProperty(String key, Integer defaultValue) {
return parent.getIntegerProperty(getFullKey(key), defaultValue);
}
public void setIntegerProperty(String key, Integer value) {
parent.setIntegerProperty(getFullKey(key), value);
}
public double getDoubleProperty(String key, double defaultValue) {
return parent.getDoubleProperty(getFullKey(key), defaultValue);
}
public void setDoubleProperty(String key, double value) {
parent.setDoubleProperty(getFullKey(key), value);
}
public boolean getBooleanProperty(String key, boolean defaultValue) {
return parent.getBooleanProperty(getFullKey(key), defaultValue);
}
public void setBooleanProperty(String key, boolean value) {
parent.setBooleanProperty(getFullKey(key), value);
}
public Boolean getBooleanProperty(String key, Boolean defaultValue) {
return parent.getBooleanProperty(getFullKey(key), defaultValue);
}
public void setBooleanProperty(String key, Boolean value) {
parent.setBooleanProperty(getFullKey(key), value);
}
public int[] getIntArrayProperty(String key, int[] defaultValue) {
return parent.getIntArrayProperty(getFullKey(key), defaultValue);
}
public void setIntArrayProperty(String key, int[] value) {
parent.setIntArrayProperty(getFullKey(key), value);
}
public Rectangle getRectangleProperty(String key, Rectangle defaultValue) {
return parent.getRectangleProperty(getFullKey(key), defaultValue);
}
public void setRectangleProperty(String key, Rectangle value) {
parent.setRectangleProperty(getFullKey(key), value);
}
public Dimension getDimensionProperty(String key, Dimension defaultValue) {
return parent.getDimensionProperty(getFullKey(key), defaultValue);
}
public void setDimensionProperty(String key, Dimension value) {
parent.setDimensionProperty(getFullKey(key), value);
}
public Color getColorProperty(String key, Color defaultValue) {
return parent.getColorProperty(getFullKey(key), defaultValue);
}
public void setColorProperty(String key, Color value) {
parent.setColorProperty(getFullKey(key), value);
}
public > T getEnumProperty(String key, T defaultValue) {
return parent.getEnumProperty(getFullKey(key), defaultValue);
}
public > void setEnumProperty(String key, T value) {
parent.setEnumProperty(getFullKey(key), value);
}
public String getFileProperty(String key, String defaultValue) {
return parent.getFileProperty(getFullKey(key), defaultValue);
}
public String getFileProperty(String key, String defaultValue, boolean absolute) {
return parent.getFileProperty(getFullKey(key), defaultValue, absolute);
}
public void setFileProperty(String key, String value) throws InvalidPathException {
parent.setFileProperty(getFullKey(key), value);
}
public void addPropertyChangeListener(String settingKey, PropertyChangeListener listener) {
throw new UnsupportedOperationException("Not implemented");
}
public void removePropertyChangeListener(String settingKey, PropertyChangeListener listener) {
throw new UnsupportedOperationException("Not implemented");
}
public Iterator getKeys() {
return new SubIterator(parent.getKeys());
}
public void removeProperty(String key) {
parent.removeProperty(getFullKey(key));
}
/**
* Iterator showing only the keys of settings managed by the SubSettingsProxy.
*
* @author Olivier Berlanger
*/
private class SubIterator implements Iterator {
private Iterator parentIter;
private String next;
SubIterator(Iterator p) {
parentIter = p;
getNext();
}
private void getNext() {
next = null;
String base = getBaseKey();
while (parentIter.hasNext() && (next == null)) {
String key = parentIter.next();
if (key.startsWith(base)) {
next = key.substring(base.length());
}
}
}
public boolean hasNext() {
return next != null;
}
public String next() {
String n = next;
getNext();
return n;
}
public void remove() {
throw new UnsupportedOperationException("Remove not supported from sub-context iterator");
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy