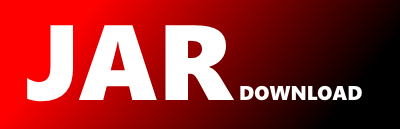
net.sf.sido.pojo.mojo.SidoPojoMojo Maven / Gradle / Ivy
The newest version!
package net.sf.sido.pojo.mojo;
import net.sf.sido.pojo.tool.Tool;
import net.sf.sido.pojo.tool.ToolListener;
import org.apache.maven.artifact.Artifact;
import org.apache.maven.model.Resource;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.project.MavenProject;
import org.codehaus.plexus.compiler.util.scan.InclusionScanException;
import org.codehaus.plexus.compiler.util.scan.SimpleSourceInclusionScanner;
import org.codehaus.plexus.compiler.util.scan.SourceInclusionScanner;
import org.codehaus.plexus.compiler.util.scan.mapping.SuffixMapping;
import java.io.File;
import java.io.IOException;
import java.net.URL;
import java.net.URLClassLoader;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Set;
/**
* Generation of POJO that bridge with SiDO instances.
*
* @goal generate
* @phase generate-sources
* @requiresDependencyResolution runtime
* @requiresProject true
*/
public class SidoPojoMojo
extends AbstractMojo {
/**
* Project the plug-in executes into.
*
* @parameter expression="${project}"
* @required
* @readonly
*/
private MavenProject project;
/**
* Specifies the directory containing SiDOL files.
*
* @parameter expression="${sido.source.dir}" default-value="${basedir}/src/main/sido"
* @required
*/
private File sourceDirectory;
/**
* Specifies the output package
*
* @parameter expression="${sido.output.package}"
* @required
*/
private String outputPackage;
/**
* Location for generated Java files.
*
* @parameter expression="${sido.output.dir}" default-value="${project.build.directory}/generated-sources/sido"
* @required
*/
private File outputDirectory;
/**
* Location for generated services files.
*
* @parameter expression="${sido.services.dir}" default-value="${project.build.directory}/generated-sources/sido-resources"
* @required
*/
private File servicesDirectory;
/**
* Flag used to indicate to generate the sources and resources in the test scope, instead
* of the normal compilation scope
*
* @parameter expression="${sido.test}" default-value="false"
*/
private boolean test = false;
public void execute()
throws MojoExecutionException {
// Summary
log("Source directory: %s", sourceDirectory);
log("Output directory: %s", outputDirectory);
log("Output package: %s", outputPackage);
log("Test: %s", test);
// Gets all SiDOL files in the source directory
Set sidolFiles;
try {
SourceInclusionScanner scan = new SimpleSourceInclusionScanner(
Collections.singleton("**/*.sidol"),
Collections.emptySet());
scan.addSourceMapping(new SuffixMapping(".sidol", Collections.emptySet()));
sidolFiles = scan.getIncludedSources(sourceDirectory, null);
} catch (InclusionScanException ex) {
throw new MojoExecutionException("Cannot get the list of SiDOL files", ex);
}
if (sidolFiles.isEmpty()) {
log("No SiDOL file has been found in %s", sourceDirectory);
return;
}
if (getLog().isInfoEnabled()) {
log("List of files to include:");
for (File sidolFile : sidolFiles) {
log(" * %s", sidolFile.getPath());
}
}
// New class loading space
ClassLoader formerClassLoader = Thread.currentThread().getContextClassLoader();
List urls = new ArrayList();
try {
// Add all the artifacts
Set artifacts = project.getArtifacts();
log("Adding all artifacts to the plug-in class path:");
for (Artifact artifact : artifacts) {
URL artifactURL = artifact.getFile().toURI().toURL();
log(" * %s", artifactURL);
urls.add(artifactURL);
}
} catch (Exception ex) {
throw new MojoExecutionException("Cannot set the classpath for the dependencies", ex);
}
URLClassLoader depLoader = new URLClassLoader(urls.toArray(new URL[urls.size()]), formerClassLoader) {
@Override
protected Class> findClass(String name) throws ClassNotFoundException {
log("[CL] findClass: %s", name);
return super.findClass(name);
}
@Override
public URL findResource(String name) {
log("[CL] findResource: %s", name);
return super.findResource(name);
}
};
// Sets the class path
log("Former class loader is: %s", formerClassLoader);
try {
// Make the child realm the ContextClassLoader
log("Using class loader: %s", depLoader);
Thread.currentThread().setContextClassLoader(depLoader);
log("Actual class loader set: %s", Thread.currentThread().getContextClassLoader());
// Instantiates the tool
Tool tool = new Tool();
// Configure the tool
tool.setSourceFiles(sidolFiles);
tool.setOutputPackage(outputPackage);
tool.setOutputDirectory(outputDirectory);
tool.setServicesDirectory(servicesDirectory);
// Listener
ToolListener toolListener = new ToolListener() {
@Override
public void log(String pattern, Object... params) {
SidoPojoMojo.this.log(pattern, params);
}
};
// Generation
try {
tool.generate(toolListener);
} catch (IOException e) {
throw new MojoExecutionException("Cannot generate the SiDO POJO files", e);
}
// Adds the output directory as sources
if (test) {
project.addTestCompileSourceRoot(outputDirectory.getPath());
} else {
project.addCompileSourceRoot(outputDirectory.getPath());
}
// Services directory as resources
Resource resource = new Resource();
resource.setDirectory(servicesDirectory.getPath());
resource.setFiltering(false);
if (test) {
project.addTestResource(resource);
} else {
project.addResource(resource);
}
} finally {
// Restores the class path
Thread.currentThread().setContextClassLoader(formerClassLoader);
}
}
protected void log(String pattern, Object... params) {
getLog().info(String.format(pattern, params));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy