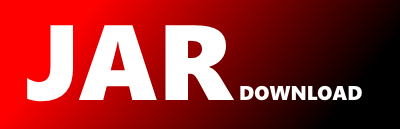
net.sf.sido.spring.DataFactoryLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sido-spring Show documentation
Show all versions of sido-spring Show documentation
SiDO Spring-related classes
package net.sf.sido.spring;
import net.sf.sido.DataFactory;
import net.sf.sido.factory.DataFactoryReader;
import net.sf.sido.factory.UniversalDataFactoryReader;
import net.sf.sido.support.DataFactories;
import org.apache.commons.lang.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Required;
import org.springframework.context.ApplicationContext;
import org.springframework.core.io.Resource;
import java.io.IOException;
import java.net.URL;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
public class DataFactoryLoader {
private final Logger logger = LoggerFactory
.getLogger(DataFactoryLoader.class);
private DataFactoryReader reader;
private String paths;
private DataFactory dataFactory;
private final ApplicationContext context;
@Autowired
public DataFactoryLoader(ApplicationContext context) {
this.context = context;
}
public DataFactory getDataFactory() {
return dataFactory;
}
public DataFactoryReader getReader() {
return reader;
}
public String getPaths() {
return paths;
}
public DataFactory load() throws IOException {
// Creates the factory if needed
DataFactory factory;
if (dataFactory != null) {
factory = dataFactory;
} else {
factory = DataFactories.typedFactory();
}
// Creates the reader if needed
DataFactoryReader factoryReader;
if (reader != null) {
factoryReader = reader;
} else {
factoryReader = new UniversalDataFactoryReader();
}
// Gets the list of all resources
List resources = new ArrayList();
// Gets the list of paths
logger.debug("Loading from paths {}", paths);
String[] pathArray = StringUtils.split(paths, ",; \t\r\n");
for (String path : pathArray) {
logger.debug("Getting resources from path {}", path);
// Loads the resources
Resource[] resourcesArray = context.getResources(path);
resources.addAll(Arrays.asList(resourcesArray));
}
// Logs the full list of paths
if (logger.isDebugEnabled()) {
logger.debug("All resources to load...");
int index = 1;
for (Resource resource : resources) {
logger.debug("\t{} - {}", index++, resource);
}
}
// Collects the URLs
Collection urls = new ArrayList();
// For each resource
for (Resource resource : resources) {
// Gets the URL
URL url = resource.getURL();
// Adss the URL
urls.add(url);
}
// Loads the URLs
factoryReader.read(urls, factory);
// OK
return factory;
}
public void setDataFactory(DataFactory dataFactory) {
this.dataFactory = dataFactory;
}
public void setReader(DataFactoryReader reader) {
this.reader = reader;
}
@Required
public void setPaths(String paths) {
this.paths = paths;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy