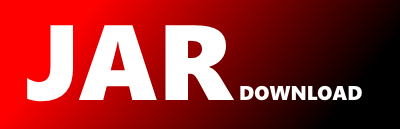
org.fife.ui.autocomplete.AbstractCompletionProvider Maven / Gradle / Ivy
/* * 12/21/2008 * * AbstractCompletionProvider.java - Base class for completion providers. * Copyright (C) 2008 Robert Futrell * robert_futrell at users.sourceforge.net * http://fifesoft.com/rsyntaxtextarea * * This library is free software; you can redistribute it and/or * modify it under the terms of the GNU Lesser General Public * License as published by the Free Software Foundation; either * version 2.1 of the License, or (at your option) any later version. * * This library is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU * Lesser General Public License for more details. * * You should have received a copy of the GNU Lesser General Public * License along with this library; if not, write to the Free Software * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA. */ package org.fife.ui.autocomplete; import java.io.Serializable; import java.util.ArrayList; import java.util.Collections; import java.util.Comparator; import java.util.List; import javax.swing.text.JTextComponent; /** * A base class for completion providers. {@link Completion}s are kept in * a sorted list. To get the list of completions that match a given input, * a binary search is done to find the first matching completion, then all * succeeding completions that also match are also returned. * * @author Robert Futrell * @version 1.0 */ public abstract class AbstractCompletionProvider extends CompletionProviderBase { /** * The completions this provider is aware of. Subclasses should ensure * that this list is sorted alphabetically (case-insensitively). */ protected List completions; /** * Compares a {@link Completion} against a String. */ protected Comparator comparator; /** * Constructor. */ public AbstractCompletionProvider() { comparator = new CaseInsensitiveComparator(); clearParameterizedCompletionParams(); } /** * Adds a single completion to this provider. If you are adding multiple * completions to this provider, for efficiency reasons please consider * using {@link #addCompletions(List)} instead. * * @param c The completion to add. * @throws IllegalArgumentException If the completion's provider isn't * this CompletionProvider. * @see #addCompletions(List) * @see #removeCompletion(Completion) * @see #clear() */ public void addCompletion(Completion c) { checkProviderAndAdd(c); Collections.sort(completions); } /** * Adds {@link Completion}s to this provider. * * @param completions The completions to add. This cannot be *
if this provider contained the specified * completion. * @see #clear() * @see #addCompletion(Completion) * @see #addCompletions(List) */ public boolean removeCompletion(Completion c) { // Don't just call completions.remove(c) as it'll be a linear search. int index = Collections.binarySearch(completions, c); if (index<0) { return false; } completions.remove(index); return true; } /** * Returns whethernull
. * @throws IllegalArgumentException If a completion's provider isn't * this CompletionProvider. * @see #addCompletion(Completion) * @see #removeCompletion(Completion) * @see #clear() */ public void addCompletions(List completions) { //this.completions.addAll(completions); for (int i=0; iCompletionProvider, if there is one. * * @see #addCompletion(Completion) * @see #addCompletions(List) * @see #removeCompletion(Completion) */ public void clear() { completions.clear(); } /** * Returns a list of Completions in this provider with the * specified input text. * * @param inputText The input text to search for. * @return A list of {@link Completion}s, or null
if there * are no matching Completions. */ public List getCompletionByInputText(String inputText) { // Find any entry that matches this input text (there may be > 1). int end = Collections.binarySearch(completions, inputText, comparator); if (end<0) { return null; } // There might be multiple entries with the same input text. int start = end; while (start>0 && comparator.compare(completions.get(start-1), inputText)==0) { start--; } int count = completions.size(); while (++endtrue str
starts withstart
, * ignoring case. * * @param str The string to check. * @param start The prefix to check for. * @return Whetherstr
starts withstart
, * ignoring case. */ protected boolean startsWithIgnoreCase(String str, String start) { int startLen = start.length(); if (str.length()>=startLen) { for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy