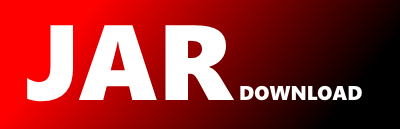
org.fife.ui.autocomplete.CompletionProvider Maven / Gradle / Ivy
/*
* 12/21/2008
*
* CompletionProvider.java - Provides autocompletion values based on the
* text currently in a text component.
* Copyright (C) 2008 Robert Futrell
* robert_futrell at users.sourceforge.net
* http://fifesoft.com/rsyntaxtextarea
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA.
*/
package org.fife.ui.autocomplete;
import java.awt.Point;
import java.util.List;
import javax.swing.ListCellRenderer;
import javax.swing.text.JTextComponent;
/**
* Provides autocompletion values to an {@link AutoCompletion}.
*
* Completion providers can have an optional parent. Parents are searched for
* completions when their children are. This allows for chaining of completion
* providers.
*
* @author Robert Futrell
* @version 1.0
*/
public interface CompletionProvider {
/**
* Clears the values used to identify and insert "parameterized completions"
* (e.g. functions or methods). After this method is called, functions and
* methods will not have their parameters auto-completed.
*
* @see #setParameterizedCompletionParams(char, String, char)
*/
public void clearParameterizedCompletionParams();
/**
* Returns the text just before the current caret position that could be
* the start of something auto-completable.
*
* @param comp The text component.
* @return The text. A return value of null
means nothing
* should be auto-completed; a value of an empty string
* (""
) means auto-completion should still be
* considered (i.e., all possible choices are valid).
*/
public String getAlreadyEnteredText(JTextComponent comp);
/**
* Gets the possible completions for the text component at the current
* caret position.
*
* @param comp The text component.
* @return The list of {@link Completion}s. If no completions are
* available, this may be null
.
*/
public List getCompletions(JTextComponent comp);
/**
* Returns the completions that have been entered at the specified visual
* location. This can be used for tool tips when the user hovers the
* mouse over completed text.
*
* @param comp The text component.
* @param p The position, usually from a MouseEvent.
* @return The completions, or an empty list if there are none.
*/
public List getCompletionsAt(JTextComponent comp, Point p);
/**
* Returns the cell renderer for completions returned from this provider.
*
* @return The cell renderer, or null
if the default should
* be used.
* @see #setListCellRenderer(ListCellRenderer)
*/
public ListCellRenderer getListCellRenderer();
/**
* Returns a list of parameterized completions that have been entered
* at the current caret position of a text component (and thus can have
* their completion choices displayed).
*
* @param tc The text component.
* @return The list of {@link ParameterizedCompletion}s. If no completions
* are available, this may be null
.
*/
public List getParameterizedCompletions(JTextComponent tc);
/**
* Returns the text that marks the end of a list of parameters to a
* function or method.
*
* @return The text for a parameter list end, for example,
* ')
'.
* @see #getParameterListStart()
* @see #getParameterListSeparator()
* @see #setParameterizedCompletionParams(char, String, char)
*/
public char getParameterListEnd();
/**
* Returns the text that separates parameters to a function or method.
*
* @return The text that separates parameters, for example,
* ",
".
* @see #getParameterListStart()
* @see #getParameterListEnd()
* @see #setParameterizedCompletionParams(char, String, char)
*/
public String getParameterListSeparator();
/**
* Returns the text that marks the start of a list of parameters to a
* function or method.
*
* @return The text for a parameter list start, for example,
* "(
".
* @see #getParameterListEnd()
* @see #getParameterListSeparator()
* @see #setParameterizedCompletionParams(char, String, char)
*/
public char getParameterListStart();
/**
* Returns the parent completion provider.
*
* @return The parent completion provider.
* @see #setParent(CompletionProvider)
*/
public CompletionProvider getParent();
/**
* Sets the renderer to use when displaying completion choices.
*
* @param r The renderer to use.
* @see #getListCellRenderer()
*/
public void setListCellRenderer(ListCellRenderer r);
/**
* Sets the values used to identify and insert "parameterized completions"
* (e.g. functions or methods). If this method isn't called, functions
* and methods will not have their parameters auto-completed.
*
* @param listStart The character that marks the beginning of a list of
* parameters, such as '(' in C or Java.
* @param separator Text that should separate parameters in a parameter
* list when one is inserted. For example, ", ".
* @param listEnd The character that marks the end of a list of parameters,
* such as ')' in C or Java.
* @throws IllegalArgumentException If either listStart or
* listEnd is not printable ASCII, or if
* separator is null
or an empty string.
* @see #clearParameterizedCompletionParams()
*/
public void setParameterizedCompletionParams(char listStart,
String separator, char listEnd);
/**
* Sets the parent completion provider.
*
* @param parent The parent provider. null
means there will
* be no parent provider.
* @see #getParent()
*/
public void setParent(CompletionProvider parent);
}