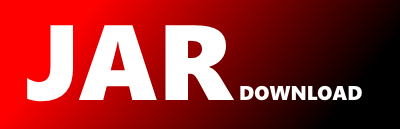
org.netbeans.editor.DelegateAction Maven / Gradle / Ivy
/*
* Sun Public License Notice
*
* The contents of this file are subject to the Sun Public License
* Version 1.0 (the "License"). You may not use this file except in
* compliance with the License. A copy of the License is available at
* http://www.sun.com/
*
* The Original Code is NetBeans. The Initial Developer of the Original
* Code is Sun Microsystems, Inc. Portions Copyright 1997-2004 Sun
* Microsystems, Inc. All Rights Reserved.
*/
package org.netbeans.editor;
import java.beans.PropertyChangeEvent;
import java.beans.PropertyChangeListener;
import javax.swing.AbstractAction;
import javax.swing.Action;
/**
* Action that delegates on delegate action.
*
* @author Miloslav Metelka, Martin Roskanin
*/
public class DelegateAction extends AbstractAction {
protected Action delegate;
private PropertyChangeListener pcl;
public DelegateAction() {
this(null);
}
public DelegateAction(Action delegate) {
this.delegate = delegate;
pcl = new PropertyChangeListener(){
public void propertyChange(PropertyChangeEvent evt){
if(evt!=null){
if ("enabled".equals(evt.getPropertyName())){ //NOI18N
setEnabled(((Boolean)evt.getNewValue()).booleanValue());
}else{
firePropertyChange(evt.getPropertyName(), evt.getOldValue(), evt.getNewValue());
}
}
}
};
}
protected final Action getDelegate() {
return delegate;
}
protected void setDelegate(Action delegate){
if (this.delegate == delegate) return;
if (delegate == this) throw new IllegalStateException("Cannot delegate on the same action"); // NOI18N
if (this.delegate != null){
this.delegate.removePropertyChangeListener(pcl);
}
if (delegate != null) {
delegate.addPropertyChangeListener(pcl);
}
this.delegate = delegate;
setEnabled((delegate != null) ? delegate.isEnabled() : false);
}
public void actionPerformed(java.awt.event.ActionEvent e) {
if (delegate != null){
delegate.actionPerformed(e);
}
}
public Object getValue(String key) {
if (delegate != null){
return delegate.getValue(key);
}else{
return super.getValue(key);
}
}
public void putValue(String key, Object value) {
if (delegate != null){
delegate.putValue(key, value);
}else{
super.putValue(key, value);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy