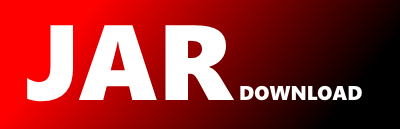
org.netbeans.editor.FoldingToolTip Maven / Gradle / Ivy
/*
* Sun Public License Notice
*
* The contents of this file are subject to the Sun Public License
* Version 1.0 (the "License"). You may not use this file except in
* compliance with the License. A copy of the License is available at
* http://www.sun.com/
*
* The Original Code is NetBeans. The Initial Developer of the Original
* Code is Sun Microsystems, Inc. Portions Copyright 1997-2003 Sun
* Microsystems, Inc. All Rights Reserved.
*/
package org.netbeans.editor;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Rectangle;
import java.awt.Shape;
import java.util.Map;
import javax.swing.JPanel;
import javax.swing.border.LineBorder;
import javax.swing.text.AbstractDocument;
import javax.swing.text.JTextComponent;
import javax.swing.text.View;
import org.netbeans.editor.view.spi.LockView;
/**
* Component for displaying folded part of code in tooltip
*
* @author Martin Roskanin
*/
public class FoldingToolTip extends JPanel {
View view;
EditorUI editorUI;
public static final int BORDER_WIDTH = 2;
/** Creates a new instance of FoldingToolTip */
public FoldingToolTip(View view, EditorUI editorUI) {
this.view = view;
this.editorUI = editorUI;
Coloring defColoring = editorUI.getColoring(SettingsNames.DEFAULT_COLORING);
Color foreColor = (defColoring != null) ? defColoring.getForeColor() : null;
setBorder(new LineBorder((foreColor == null) ? Color.BLACK : foreColor));
setOpaque(true);
}
public void setSize(Dimension d){
setSize(d.width, d.height);
}
/** Setting size of popup panel. The height and size is computed to fit the best place on the screen */
public void setSize(int width, int height){
int viewHeight = (int) view.getPreferredSpan(View.Y_AXIS);
int viewWidth = (int) view.getPreferredSpan(View.X_AXIS);
if (height<30) {
putClientProperty(PopupManager.Placement.class, null);
}else{
height = Math.min(height, viewHeight);
}
height += 2*BORDER_WIDTH;
width = Math.min(width, viewWidth);
super.setSize(width,height);
}
private void updateRenderingHints(Graphics g){
JTextComponent comp = editorUI.getComponent();
if (comp == null) return;
Object value = Settings.getValue(Utilities.getKitClass(comp), SettingsNames.RENDERING_HINTS);
Map renderingHints = (value instanceof Map) ? (java.util.Map)value : null;
// Possibly apply the rendering hints
if (renderingHints != null) {
((java.awt.Graphics2D)g).setRenderingHints(renderingHints);
}
}
protected void paintComponent(Graphics g) {
updateRenderingHints(g);
Rectangle shape = new Rectangle(getSize());
Rectangle clip = g.getClipBounds();
Coloring defaultColoring = editorUI.getColoring(SettingsNames.DEFAULT_COLORING);
if (defaultColoring!=null && defaultColoring.getBackColor()!=null){
g.setColor(defaultColoring.getBackColor());
}else{
g.setColor(SettingsDefaults.defaultBackColor);
}
g.fillRect(clip.x, clip.y, clip.width, clip.height);
g.translate(BORDER_WIDTH, BORDER_WIDTH);
JTextComponent component = editorUI.getComponent();
if (component == null) return;
int sideBarWidth = editorUI.getSideBarWidth();
GlyphGutter gg = editorUI.getGlyphGutter();
if (gg!=null){
View docView = null;
if (view.getViewCount() == 1){//lockview
docView = view.getView(0);
}
int y = 0;
if (docView!=null){
AbstractDocument doc = (AbstractDocument)docView.getDocument();
doc.readLock();
try {
LockView lockView = LockView.get(docView);
if (lockView != null) {
lockView.lock();
try {
for (int i = 0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy