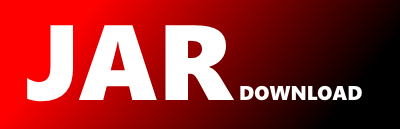
org.netbeans.editor.ext.KeyEventBlocker Maven / Gradle / Ivy
/*
* Sun Public License Notice
*
* The contents of this file are subject to the Sun Public License
* Version 1.0 (the "License"). You may not use this file except in
* compliance with the License. A copy of the License is available at
* http://www.sun.com/
*
* The Original Code is NetBeans. The Initial Developer of the Original
* Code is Sun Microsystems, Inc. Portions Copyright 1997-2003 Sun
* Microsystems, Inc. All Rights Reserved.
*/
package org.netbeans.editor.ext;
import java.awt.Component;
import java.awt.KeyboardFocusManager;
import java.awt.event.KeyListener;
import java.awt.event.KeyEvent;
import java.util.LinkedList;
import javax.swing.text.JTextComponent;
/**
*
* @author Dusan Balek
*/
public class KeyEventBlocker implements KeyListener {
private LinkedList blockedEvents = new LinkedList();
private JTextComponent component;
private boolean discardKeyTyped = true;
public KeyEventBlocker(JTextComponent component, boolean discardFirstKeyTypedEvent) {
this.component = component;
this.discardKeyTyped = discardFirstKeyTypedEvent;
this.component.addKeyListener(this);
}
/** Has to be called from AWT event thread to be properly synchronized */
public void stopBlocking() {
this.component.removeKeyListener(this);
KeyboardFocusManager kfm = KeyboardFocusManager.getCurrentKeyboardFocusManager();
while(!blockedEvents.isEmpty()) {
KeyEvent e = (KeyEvent)blockedEvents.removeFirst();
e = new KeyEvent((Component)e.getSource(), e.getID(), e.getWhen(), e.getModifiers(), e.getKeyCode(), e.getKeyChar(), e.getKeyLocation());
kfm.dispatchEvent(e);
}
}
public void keyPressed(KeyEvent e) {
e.consume();
blockedEvents.add(e);
}
public void keyReleased(KeyEvent e) {
e.consume();
blockedEvents.add(e);
}
public void keyTyped(KeyEvent e) {
e.consume();
if (discardKeyTyped) {
discardKeyTyped = false;
} else {
blockedEvents.add(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy