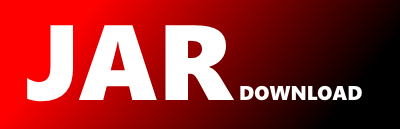
org.netbeans.editor.ext.ScrollJavaDocPane Maven / Gradle / Ivy
/*
* Sun Public License Notice
*
* The contents of this file are subject to the Sun Public License
* Version 1.0 (the "License"). You may not use this file except in
* compliance with the License. A copy of the License is available at
* http://www.sun.com/
*
* The Original Code is NetBeans. The Initial Developer of the Original
* Code is Sun Microsystems, Inc. Portions Copyright 1997-2000 Sun
* Microsystems, Inc. All Rights Reserved.
*/
package org.netbeans.editor.ext;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.awt.Dimension;
import java.awt.Rectangle;
import javax.swing.JScrollPane;
import javax.swing.JComponent;
import javax.swing.JRootPane;
import javax.swing.text.JTextComponent;
import javax.swing.JLayeredPane;
import javax.swing.JList;
import javax.swing.SwingUtilities;
import javax.swing.JPanel;
import org.netbeans.editor.SettingsChangeListener;
import org.netbeans.editor.Utilities;
import org.netbeans.editor.SettingsUtil;
import org.netbeans.editor.ext.ExtSettingsNames;
import org.netbeans.editor.ext.ExtSettingsDefaults;
import org.netbeans.editor.SettingsChangeEvent;
import org.netbeans.editor.Settings;
import javax.swing.JButton;
import javax.swing.border.CompoundBorder;
import javax.swing.border.EtchedBorder;
import javax.swing.border.EmptyBorder;
import java.awt.BorderLayout;
import java.awt.Color;
import javax.swing.ImageIcon;
import java.net.URL;
import javax.swing.Icon;
import java.awt.FlowLayout;
import java.awt.GridBagConstraints;
import java.awt.Insets;
import java.awt.GridLayout;
import java.awt.ComponentOrientation;
import java.awt.GridBagLayout;
import javax.swing.BoxLayout;
import javax.swing.AbstractAction;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.BorderFactory;
import javax.swing.event.HyperlinkListener;
import javax.swing.event.HyperlinkEvent;
import javax.swing.JEditorPane;
import javax.swing.UIManager;
import javax.swing.border.Border;
import javax.swing.border.LineBorder;
import org.netbeans.editor.LocaleSupport;
/**
* JScrollPane implementation of JavaDocPane.
*
* @author Martin Roskanin
* @since 03/2002
*/
public class ScrollJavaDocPane extends JPanel implements JavaDocPane, SettingsChangeListener{
protected ExtEditorUI extEditorUI;
private JComponent view;
private CompletionJavaDoc cjd;
protected JScrollPane scrollPane = new JScrollPane();
Border lineBorder;
/** Creates a new instance of ScrollJavaDocPane */
public ScrollJavaDocPane(ExtEditorUI extEditorUI) {
// new RuntimeException("ScrollJavaDocPane.").printStackTrace();
setLayout(null);
this.extEditorUI = extEditorUI;
// Add the completionJavaDoc view
cjd = extEditorUI.getCompletionJavaDoc();
if (cjd!=null){
JavaDocView javaDocView = cjd.getJavaDocView();
if (javaDocView instanceof JComponent) {
if (javaDocView instanceof JEditorPane){
((JEditorPane)javaDocView).addHyperlinkListener(createHyperlinkAction());
}
view = (JComponent)javaDocView;
scrollPane.setViewportView(view);
}
Settings.addSettingsChangeListener(this);
setMinimumSize(new Dimension(100,100)); //[PENDING] put it into the options
setMaximumSize(getMaxPopupSize());
}else{
setMinimumSize(new Dimension(0,0));
setMaximumSize(new Dimension(0,0));
}
super.setVisible(false);
add(scrollPane);
getAccessibleContext().setAccessibleDescription(LocaleSupport.getString("ACSD_JAVADOC_javaDocPane")); //NOI18N
// !!! virtual method called from contructor!!
installTitleComponent();
setBorder(new LineBorder(javax.swing.UIManager.getColor("controlDkShadow"))); //NOI18N
}
protected HyperlinkAction createHyperlinkAction(){
return new HyperlinkAction();
}
public void setBounds(Rectangle r){
super.setBounds(r);
scrollPane.setBounds(r.x, 0, r.width+1, r.height );
}
public void setVisible(boolean visible){
super.setVisible(visible);
if (cjd!=null && !visible){
cjd.clearHistory();
}
}
protected ImageIcon resolveIcon(String res){
ClassLoader loader = this.getClass().getClassLoader();
URL resource = loader.getResource( res );
if( resource == null ) resource = ClassLoader.getSystemResource( res );
return ( resource != null ) ? new ImageIcon( resource ) : null;
}
protected void installTitleComponent() {
}
private Dimension getMaxPopupSize(){
Class kitClass = Utilities.getKitClass(extEditorUI.getComponent());
if (kitClass != null) {
return (Dimension)SettingsUtil.getValue(kitClass,
ExtSettingsNames.JAVADOC_PREFERRED_SIZE,
ExtSettingsDefaults.defaultJavaDocAutoPopupDelay);
}
return ExtSettingsDefaults.defaultJavaDocPreferredSize;
}
public void settingsChange(SettingsChangeEvent evt) {
if (ExtSettingsNames.JAVADOC_PREFERRED_SIZE.equals(evt.getSettingName())){
setMaximumSize(getMaxPopupSize());
}
}
public JComponent getComponent() {
return this;
}
public void setForwardEnabled(boolean enable) {
}
public void setBackEnabled(boolean enable) {
}
public void setShowWebEnabled(boolean enable) {
}
public JComponent getJavadocDisplayComponent() {
return scrollPane;
}
public class BrowserButton extends JButton {
public BrowserButton() {
setBorderPainted(false);
setFocusPainted(false);
}
public BrowserButton(String text){
super(text);
setBorderPainted(false);
setFocusPainted(false);
}
public BrowserButton(Icon icon){
super(icon);
setBorderPainted(false);
setFocusPainted(false);
}
}
protected class HyperlinkAction implements HyperlinkListener{
public HyperlinkAction(){
}
public void hyperlinkUpdate(HyperlinkEvent e) {
if (e!=null && HyperlinkEvent.EventType.ACTIVATED.equals(e.getEventType())){
if (e.getDescription() != null){
Object obj = cjd.parseLink(e.getDescription(), null);
if (obj!=null){
cjd.setContent(obj, false);
cjd.addToHistory(obj);
} else {
obj = (e.getURL() == null) ? e.getDescription() : (Object)e.getURL();
cjd.setContent(obj, false);
}
}
}
}
}
/*
private class BackAction implements ActionListener{
public void actionPerformed(ActionEvent evt) {
if (cjd!=null){
System.out.println("back");
cjd.backHistory();
}
}
}
private class ForwardAction implements ActionListener {
public void actionPerformed(ActionEvent evt) {
if (cjd!=null){
System.out.println("fwd");
cjd.forwardHistory();
}
}
}
*/
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy