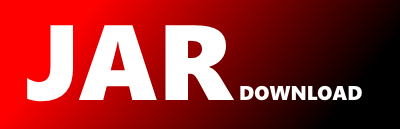
net.sourceforge.squirrel_sql.fw.gui.WindowState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fw Show documentation
Show all versions of fw Show documentation
The framework library contains utility classes that are generic and useful for building applications
that introspect a database via JDBC. These are not intended to be SQuirreLSQL-specific and could be
used by other projects JDBC front-end applications. This project is guaranteed to have no code
dependencies on other SQuirreLSQL projects and could therefore be used when building a different
JDBC front-end application.
package net.sourceforge.squirrel_sql.fw.gui;
/*
* Copyright (C) 2001-20064 Colin Bell
* [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
import java.awt.Frame;
import java.awt.Rectangle;
import java.awt.Window;
import java.io.Serializable;
import javax.swing.JInternalFrame;
import net.sourceforge.squirrel_sql.fw.util.beanwrapper.RectangleWrapper;
import net.sourceforge.squirrel_sql.fw.xml.IXMLAboutToBeWritten;
/**
* This bean will store the state of a window or an internal frame object.
*
* @author Colin Bell
*/
public class WindowState implements IXMLAboutToBeWritten, Serializable
{
private static final long serialVersionUID = 2664203798124718385L;
/**
* Window whose state is being stored. Only one of _window,
* _frame and _internalFrame can be non-null.
*/
private Window _window;
/**
* JInternalFrame whose state is being stored. Only one of _window,
* _frame and _internalFrame can be non-null.
*/
private JInternalFrame _internalFrame;
/**
* Frame whose state is being stored. Only one of _window,
* _frame and _internalFrame can be non-null.
*/
private Frame _frame;
/** Window bounds. */
private RectangleWrapper _bounds = new RectangleWrapper(new Rectangle(600, 400));
/** Was the window visible. */
private boolean _visible = true;
/** Extended state for frame only. */
private int _frameExtendedState = 0;
public interface IPropertyNames
{
String BOUNDS = "bounds";
String FRAME_EXTENDED_STATE = "frameExtendedState";
String VISIBLE = "visible";
}
/**
* Default ctor.
*/
public WindowState()
{
super();
}
/**
* Ctor storing the state of the passed Window
.
*
* @param window Window to store the state of.
*/
public WindowState(Window window)
{
super();
_window = window;
}
/**
* Ctor storing the state of the passed JInternalFrame
.
*
* @param internalFrame JInternalFrame to store the state of.
*/
public WindowState(JInternalFrame internalFrame)
{
super();
_internalFrame = internalFrame;
}
/**
* Ctor storing the state of the passed Frame
.
*
* @param frame frame to store the state of.
*/
public WindowState(Frame frame)
{
super();
_frame = frame;
}
/**
* Set this objects state to that of the passed object. Think of this as
* being like an assignment operator
*
* @param obj Object to copy state from
*
* @throws IllegalArgumentException
* Thrown if null WindowState passed.
*/
public void copyFrom(WindowState obj)
{
if (obj == null)
{
throw new IllegalArgumentException("WindowState == null");
}
setBounds(obj.getBounds());
setVisible(obj.isVisible());
setFrameExtendedState(obj.getFrameExtendedState());
}
/**
* This bean is about to be written out to XML so load its values from its
* window.
*/
public void aboutToBeWritten()
{
refresh();
}
public RectangleWrapper getBounds()
{
refresh();
return _bounds;
}
public void setBounds(RectangleWrapper value)
{
_bounds = value;
_window = null;
_internalFrame = null;
}
public boolean isVisible()
{
refresh();
return _visible;
}
public void setVisible(boolean value)
{
_visible = value;
}
public int getFrameExtendedState()
{
refresh();
return _frameExtendedState;
}
public void setFrameExtendedState(int value)
{
_frameExtendedState = value;
}
private void refresh()
{
Rectangle windRc = null;
if (_window != null)
{
windRc = _window.getBounds();
_visible = _window.isVisible();
}
else if (_internalFrame != null)
{
windRc = _internalFrame.getBounds();
_visible = _internalFrame.isVisible();
}
else if (_frame != null)
{
windRc = _frame.getBounds();
_visible = _frame.isVisible();
_frameExtendedState = _frame.getExtendedState();
}
if (windRc != null)
{
if (_bounds == null)
{
_bounds = new RectangleWrapper();
}
_bounds.setFrom(windRc);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy