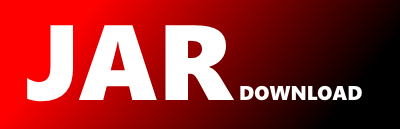
net.sourceforge.squirrel_sql.fw.xml.XMLObjectCache Maven / Gradle / Ivy
Show all versions of fw Show documentation
package net.sourceforge.squirrel_sql.fw.xml;
/*
* Copyright (C) 2001-2003 Colin Bell
* [email protected]
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.Reader;
import java.util.Iterator;
import net.sourceforge.squirrel_sql.fw.id.IHasIdentifier;
import net.sourceforge.squirrel_sql.fw.id.IIdentifier;
import net.sourceforge.squirrel_sql.fw.util.DuplicateObjectException;
import net.sourceforge.squirrel_sql.fw.util.IObjectCache;
import net.sourceforge.squirrel_sql.fw.util.IObjectCacheChangeListener;
import net.sourceforge.squirrel_sql.fw.util.ObjectCache;
import net.sourceforge.squirrel_sql.fw.util.StringManager;
import net.sourceforge.squirrel_sql.fw.util.StringManagerFactory;
/**
* This class is a cache of objects that can be read from/written to an XML
* document. All objects stored must implement IHasIdentifier
.
*
* It is implemented using ObjectCache
.
*
* @author Colin Bell
*/
public class XMLObjectCache implements IObjectCache
{
/** Internationalized strings for this class. */
private static final StringManager s_stringMgr =
StringManagerFactory.getStringManager(XMLObjectCache.class);
/** Cache of stored objects. */
private ObjectCache _cache = new ObjectCache();
/**
* Default ctor.
*/
public XMLObjectCache()
{
super();
}
/**
* Retrieve a stored object.
*
* @param objClass The class of the object to be retrieved.
* @param id The IIdentifier
that identifies
* the object to be retrieved.
*
* @return The IHasIdentifier
retrieved or null
* if no object exists for id
.
*/
public IHasIdentifier get(Class objClass, IIdentifier id)
{
return _cache.get(objClass, id);
}
/**
* Store an object.
*
* @param obj Object to be stored.
*
* @exception DuplicateObjectException
* Thrown if an object of the same class as obj
* and with the same identifier is already in the cache.
*/
public void add(E obj) throws DuplicateObjectException
{
_cache.add(obj);
}
/**
* Remove an object.
*
* @param objClass Class of object to be removed.
* @param id Identifier for object to be removed.
*/
public void remove(Class objClass, IIdentifier id)
{
_cache.remove(objClass, id);
}
/**
* Return an array of Class
[] getAllClasses()
{
return _cache.getAllClasses();
}
/**
* Return an Iterator
of all objects stored for the
* passed class.
*
* @param objClass Class to return objects for.
*
* @return Iterator
over all objects.
*/
public Iterator getAllForClass(Class objClass)
{
return _cache.getAllForClass(objClass);
}
/**
* Adds a listener for changes to the cache entry for the passed class.
*
* @param lis an IObjectCacheChangeListener that will be notified
* when objects are added and removed from this cache
* entry.
* @param objClass The class of objects whose cache we want to listen
* to.
*/
public void addChangesListener(IObjectCacheChangeListener lis, Class objClass)
{
_cache.addChangesListener(lis, objClass);
}
/**
* Removes a listener for changes to the cache entry for the passed class.
*
* @param lis an IObjectCacheChangeListener that will be notified
* when objects are added and removed from this cache
* entry.
* @param objClass The class of objects whose cache we want to listen
* to.
*/
public void removeChangesListener(IObjectCacheChangeListener lis,
Class objClass)
{
_cache.removeChangesListener(lis, objClass);
}
/**
* Load from an XML document.
*
* @param xmlFileName Name of XML file to load from.
*
* @exception FileNotFoundException
* Thrown if file not found.
*
* @exception XMLException
* Thrown if an XML error occurs.
*
* @exception DuplicateObjectException
* Thrown if two objects of the same class
* and with the same identifier are added to the cache.
*/
public void load(String xmlFileName)
throws FileNotFoundException, XMLException, DuplicateObjectException
{
load(xmlFileName, null);
}
/**
* Load from an XML document but don't ignore duplicate objects.
*
* @param xmlFileName Name of XML file to load from.
* @param cl Class loader to use for object creation.
*
* @exception FileNotFoundException
* Thrown if file not found.
*
* @exception XMLException
* Thrown if an XML error occurs.
*
* @exception DuplicateObjectException
* Thrown if two objects of the same class
* and with the same identifier are added to the cache.
*/
public void load(String xmlFileName, ClassLoader cl)
throws FileNotFoundException, XMLException, DuplicateObjectException
{
XMLBeanReader rdr = new XMLBeanReader();
rdr.load(xmlFileName, cl);
for (Iterator