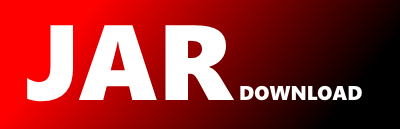
net.sourceforge.squirrel_sql.client.session.properties.EditWhereColsPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of squirrel-sql Show documentation
Show all versions of squirrel-sql Show documentation
This is the jar that contains the main application classes which are very specific to
SQuirreLSQL.
package net.sourceforge.squirrel_sql.client.session.properties;
/*
*
* Adapted from WhereClausePanel.java by Maury Hammel.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.SortedSet;
import java.util.TreeSet;
import javax.swing.JButton;
import javax.swing.JLabel;
import javax.swing.JList;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.ListModel;
import net.sourceforge.squirrel_sql.client.session.ISession;
import net.sourceforge.squirrel_sql.fw.sql.ITableInfo;
import net.sourceforge.squirrel_sql.fw.sql.PrimaryKeyInfo;
import net.sourceforge.squirrel_sql.fw.util.StringManager;
import net.sourceforge.squirrel_sql.fw.util.StringManagerFactory;
import net.sourceforge.squirrel_sql.fw.util.log.ILogger;
import net.sourceforge.squirrel_sql.fw.util.log.LoggerController;
/**
* This panel allows the user to select specific columns from a specific table for use in
* the WHERE clause when editing a cell in a table. This is useful if the table has a large number
* of columns and the WHERE clause generated using all the columns exceeds the DBMS limit.
*/
@SuppressWarnings("serial")
public class EditWhereColsPanel extends JPanel
{
private static final StringManager s_stringMgr =
StringManagerFactory.getStringManager(EditWhereColsPanel.class);
/** Logger for this class. */
private static final ILogger s_log =
LoggerController.createLogger(EditWhereColsPanel.class);
/** The name of the database table the Where clause applies to. */
private String _tableName;
/** The name of the table including the URL **/
private String _unambiguousTableName;
/** The list of all possible columns in the table **/
private SortedSet _columnList;
/** The list of "to use" column names as seen by the user **/
private JList useColsList;
/** The list of "to NOT use" column names as seen by the user **/
private JList notUseColsList;
/** The list of column names to use as calculated when window is created **/
private Object[] initalUseColsArray;
/** The list of column names to NOT use as calculated when window is created **/
private Object[] initalNotUseColsArray;
private ISession _session = null;
private PrimaryKeyInfo[] primaryKeyInfos = null;
EditWhereCols _editWhereCols = new EditWhereCols();
/**
* ?? this should be changed to use the I18N file mechanism.
*/
interface EditWhereColsPanelI18N {
// i18n[editWhereColsPanel.limitColsInCell=Limit Columns in Cell Edit]
String TITLE = s_stringMgr.getString("editWhereColsPanel.limitColsInCell");
// i18n[editWhereColsPanel.limitColsInCellHint=Limit columns used in WHERE clause when editing table]
String HINT = s_stringMgr.getString("editWhereColsPanel.limitColsInCellHint");
// i18n[editWhereColsPanel.usePKLabel=Use PK]
String USE_PK = s_stringMgr.getString("editWhereColsPanel.usePKLabel");
}
/**
* Create a new instance of a WhereClausePanel.
*
* @param columnList A list of column names for the database table.
* @param tableName The name of the database table that the filter
* information will apply to.
* @param unambiguousTableName The name of the table including the URL
* to the specific DBMS
*
* @throws IllegalArgumentException
* The exception thrown if invalid arguments are passed.
*/
public EditWhereColsPanel(ISession session,
ITableInfo ti,
SortedSet columnList,
String unambiguousTableName)
throws IllegalArgumentException
{
super();
_session = session;
_editWhereCols.setApplication(session.getApplication());
getPrimaryKey(ti);
// save the input for use later
_columnList = columnList;
_tableName = ti.getQualifiedName();
_unambiguousTableName = unambiguousTableName;
// look up the table in the EditWhereCols list
HashMap colsTable = EditWhereCols.get(unambiguousTableName);
if (colsTable == null) {
// use all of the columns
initalUseColsArray = _columnList.toArray();
initalNotUseColsArray = new Object[0];
}
else {
// use just the columns listed in the table, and set the not-used cols to the ones
// that are not mentioned in the table
SortedSet
© 2015 - 2025 Weber Informatics LLC | Privacy Policy