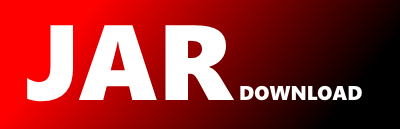
net.sf.staccatocommons.lang.CollectionBuilder Maven / Gradle / Ivy
/*
Copyright (c) 2011, The Staccato-Commons Team
This program is free software; you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as published by
the Free Software Foundation; version 3 of the License.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
*/
package net.sf.staccatocommons.lang;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.LinkedList;
import java.util.List;
import java.util.Set;
import java.util.SortedSet;
import java.util.TreeSet;
import net.sf.staccatocommons.defs.Applicable;
import net.sf.staccatocommons.defs.Evaluable;
import net.sf.staccatocommons.lang.builder.Builder;
import net.sf.staccatocommons.lang.builder.BuilderAlreadyUsedException;
import net.sf.staccatocommons.lang.function.AbstractFunction;
import net.sf.staccatocommons.lang.function.Functions;
import net.sf.staccatocommons.restrictions.Constant;
import net.sf.staccatocommons.restrictions.check.NonNull;
import net.sf.staccatocommons.restrictions.value.Unmodifiable;
/**
* a {@link Builder} for {@link Collection}s. With the exception of
* {@link #from(Collection, Applicable)}, all its factory methods grant to
* return CollectionBuilder that build {@link Unmodifiable} collections
*
* @author flbulgarelli
*/
public class CollectionBuilder> implements Builder {
private B list;
private Applicable wrapperFunction;
/**
* Creates a new {@link CollectionBuilder}
*
* @param list
* the list to be configured by this builder
* @param wrapperFunction
*/
public CollectionBuilder(@NonNull B list, Applicable wrapperFunction) {
this.list = list;
this.wrapperFunction = wrapperFunction;
}
/**
* Adds an element to the list
*
* @param element
* @return this builder
*/
@NonNull
public CollectionBuilder with(A element) {
list.add(element);
return this;
}
/**
* Adds an element to the list if it satisfies the given condition
*
* @param element
* @param evaluable
* @return this
*/
@NonNull
public CollectionBuilder withIf(A element, @NonNull Evaluable super A> evaluable) {
if (evaluable.eval(element))
with(element);
return this;
}
/**
* Sets the wrapper function, that is, the {@link Applicable} to apply to the
* resulting collection before returning it through {@link #build()}.
*
* By default, collections are wrapped with unmodifiable wrappers, but this
* behavior can be changed through this method.
*
* @param wrapperFunction
* @return this
*/
@NonNull
public CollectionBuilder withWrapper(@NonNull Applicable wrapperFunction) {
this.wrapperFunction = wrapperFunction;
return this;
}
/**
* Disables wrapping, which means that a built collection will be returned
* just as it was created.
*
* As a consequence, the collection returned by {@link #build()} will be
* modifiable.
*
* @return this
*/
@NonNull
public CollectionBuilder unwrap() {
return withWrapper(Functions. identity());
}
@NonNull
public B build() throws BuilderAlreadyUsedException {
if (list == null)
throw new BuilderAlreadyUsedException();
B list = this.list;
this.list = null;
return wrapperFunction.apply(list);
}
/**
* Answers a new {@link CollectionBuilder} that configures a
* {@link Collection}
*
* @param
* @return a new {@link CollectionBuilder} that builds a {@link Collection}
*/
@NonNull
public static CollectionBuilder> from(@NonNull Collection collection) {
return from(collection, CollectionBuilder. toUnmodifiableCollection());
}
/**
* Answers a new {@link CollectionBuilder} that configures a {@link SortedSet}
* , with the given element already added
*
* @param
* @return a new {@link CollectionBuilder} that builds a {@link SortedSet}
*/
@NonNull
public static CollectionBuilder> sortedSetWith(A element) {
return from(new TreeSet(), CollectionBuilder. toUnmodifiableSortedSet()).with(element);
}
/**
* Answers a new {@link CollectionBuilder} that configures a {@link Set}, with
* the given element already added
*
* @param
* @return a new {@link CollectionBuilder} that builds a set
*/
@NonNull
public static CollectionBuilder> setWith(A element) {
return from(new HashSet(), CollectionBuilder. toUnmodifiableSet()).with(element);
}
/**
* Answers a new {@link CollectionBuilder} that configures a {@link List},
* with the given element already added. The list built this builder grants to
* be {@link Unmodifiable}
*
* @param
* @return a new {@link CollectionBuilder} that builds lists
*/
@NonNull
public static CollectionBuilder> listWith(A element) {
return from(new LinkedList(), CollectionBuilder. toUnmodifiableList()).with(element);
}
@NonNull
private static > CollectionBuilder from(@NonNull B collection,
@NonNull Applicable immutator) {
return new CollectionBuilder(collection, immutator);
}
/**
* Answers a constant function that returns an {@link Unmodifiable} view of
* its {@link SortedSet} argument
*
* @param
* @return a function
*/
@Constant
public static Applicable, Set> toUnmodifiableSet() {
return new AbstractFunction, Set>() {
public Set apply(Set arg) {
return Collections.unmodifiableSet(arg);
}
};
}
/**
* Answers a constant function that returns an {@link Unmodifiable} view of
* its {@link SortedSet} argument
*
* @param
* @return a function
*/
@Constant
public static Applicable, SortedSet> toUnmodifiableSortedSet() {
return new AbstractFunction, SortedSet>() {
public SortedSet apply(SortedSet arg) {
return Collections.unmodifiableSortedSet(arg);
}
};
}
/**
* Answers a constant function that returns an {@link Unmodifiable} view of
* its list argument
*
* @param
* @return a function
*/
@Constant
public static Applicable, List> toUnmodifiableList() {
return new AbstractFunction, List>() {
public List apply(List arg) {
return Collections.unmodifiableList(arg);
}
};
}
/**
* Answers a constant function that returns an {@link Unmodifiable} view of
* its collection argument
*
* @param
* @return a function
*/
@Constant
public static Applicable, Collection> toUnmodifiableCollection() {
return new AbstractFunction, Collection>() {
public Collection apply(Collection arg) {
return Collections.unmodifiableCollection(arg);
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy