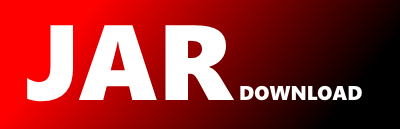
net.sf.staccatocommons.lang.Compare Maven / Gradle / Ivy
/**
* Copyright (c) 2011, The Staccato-Commons Team
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*/
package net.sf.staccatocommons.lang;
import java.util.Comparator;
import net.sf.staccatocommons.defs.Applicable;
import net.sf.staccatocommons.defs.function.Function2;
import net.sf.staccatocommons.defs.predicate.Predicate;
import net.sf.staccatocommons.lang.function.AbstractFunction2;
import net.sf.staccatocommons.lang.internal.NaturalComparator;
import net.sf.staccatocommons.lang.predicate.AbstractPredicate;
import net.sf.staccatocommons.lang.predicate.Predicates;
import net.sf.staccatocommons.lang.predicate.internal.GreaterThan;
import net.sf.staccatocommons.lang.predicate.internal.LessThan;
import net.sf.staccatocommons.restrictions.Constant;
import net.sf.staccatocommons.restrictions.check.NonNull;
import net.sf.staccatocommons.restrictions.processing.IgnoreRestrictions;
/**
* Class methods that implement comparisons for {@link Comparable}s, like
* between(max, min) and in(collection)
*
* @author flbulgarelli
*
*/
public class Compare {
/**
* Tests that given three {@link Comparable}s element
,
* min
and max
, is true that:
*
*
* min <= element <= max
*
*
* @param
* the type of comparable element
* @param element
* non null
* @param min
* non null
* @param max
* non null
* @return if element is between min inclusive, and max, inclusive
*/
@IgnoreRestrictions
public static > boolean between(@NonNull T element, @NonNull T min, @NonNull T max) {
return between(element, min, max, Compare. natural());
}
/**
* Tests that given three {@link Comparable}s element
,
* min
and max
, and a {@link Comparator}, using that
* comparator is true that:
*
*
* min <= element <= max
*
*
* @param
* the type of comparable element
* @param element
* non null
* @param min
* non null
* @param max
* non null
* @param comparator
* non null
* @return if element is between min inclusive, and max, inclusive, using the
* given {@link Comparator} as ordering criteria
*/
public static boolean between(@NonNull T element, @NonNull T min, @NonNull T max,
@NonNull Comparator comparator) {
return comparator.compare(element, max) <= 0 && comparator.compare(element, min) >= 0;
}
/**
* Tests that given three long
s element
,
* min
and max
, is true that:
*
*
* min <= element <= max
*
*
* @param element
* non null
* @param min
* non null
* @param max
* non null
* @return if element is between min inclusive, and max, inclusive
*/
public static boolean between(long element, long min, long max) {
return element <= max && element >= min;
}
/**
* Tests that given three int
s element
,
* min
and max
, is true that:
*
*
* min <= element <= max
*
*
* @param element
* non null
* @param min
* non null
* @param max
* non null
* @return if element is between min inclusive, and max, inclusive
*/
public static boolean between(int element, int min, int max) {
return element <= max && element >= min;
}
/**
* Answers a predicate that evalutes if its argument is between
* min
and max
*
* @param
* @param min
* @param max
* @return a new {@link Predicate}
*/
public static > Predicate between(@NonNull final T min, @NonNull final T max) {
return new AbstractPredicate() {
public boolean eval(T argument) {
return between(argument, min, max);
}
};
}
/**
* Tests if a given array contains an element
*
* @param element
* @param values
* @return if the array contains the given element
*/
public static boolean in(int element, @NonNull int[] values) {
for (int value : values)
if (element == value)
return true;
return false;
}
/**
* Tests if a given array contains an element
*
* @param element
* @param values
* @return if the array contains the given element
*/
public static boolean in(long element, @NonNull long[] values) {
for (long value : values)
if (element == value)
return true;
return false;
}
/**
* Tests if a given array contains an element
*
* @param
*
* @param element
* @param values
* @return if the array contains the given element, using equals comparison
*/
public static boolean in(@NonNull T element, @NonNull T... values) {
for (T value : values)
if (element.equals(value))
return true;
return false;
}
/**
* Returns a predicate that tests if its argument is equal to any of the given
* values
*
* @param
* @param values
* @return a new {@link Predicate}
*/
@IgnoreRestrictions
public static Predicate in_(@NonNull T... values) {
return Predicates.in(values);
}
/**
* @param
* @param c1
* @param c2
* @return c1 if it is lower than or equal to c2, c2 otherwise.
*/
@NonNull
@IgnoreRestrictions
public static > T min(@NonNull T c1, @NonNull T c2) {
return min(c1, c2, Compare. natural());
}
/**
* @param
* @param c1
* @param c2
* @return c1 if it is greater than or equal to c2, c2 otherwise.
*/
@NonNull
@IgnoreRestrictions
public static > T max(@NonNull T c1, @NonNull T c2) {
return max(c1, c2, Compare. natural());
}
/**
* Answers a new {@link AbstractFunction2} that returns the min of its
* arguments using the given comparator.
*
* @param
* @param comparator
* @return a new {@link AbstractFunction2}
*/
@NonNull
public static Function2 min(@NonNull final Comparator super A> comparator) {
return new AbstractFunction2() {
public A apply(A arg0, A arg1) {
return Compare.min(arg0, arg1, comparator);
}
};
}
/**
* Answers a new {@link AbstractFunction2} that returns the max of its
* arguments using the given comparator.
*
* @param
* @param comparator
* @return a new {@link AbstractFunction2}
*/
@NonNull
public static Function2 max(@NonNull final Comparator super A> comparator) {
return new AbstractFunction2() {
public A apply(A arg0, A arg1) {
return Compare.max(arg0, arg1, comparator);
}
};
}
/**
* Answers the min element between c1
and c2
, using
* the given {@link Comparator}
*
* @param
* @param c1
* @param c2
* @param comparator
* @return comparator.compare(c1, c2) >= 0 ? c1 : c2
*/
@NonNull
public static T max(@NonNull T c1, @NonNull T c2, @NonNull Comparator super T> comparator) {
return comparator.compare(c1, c2) >= 0 ? c1 : c2;
}
/**
* Answers the max element between c1
and c2
, using
* the given {@link Comparator}
*
* @param
* @param c1
* @param c2
* @param comparator
* @return comparator.compare(c1, c2) <= 0 ? c1 : c2
*/
@NonNull
public static T min(@NonNull T c1, @NonNull T c2, Comparator super T> comparator) {
return comparator.compare(c1, c2) <= 0 ? c1 : c2;
}
/**
* Answers a new {@link Comparator} that performs the comparison between the
* results of applying the given function
to its arguments.
*
* @param
* @param
* @param function
* @return a new {@link Comparator}
*/
@NonNull
public static > Comparator on(@NonNull final Applicable super A, B> function) {
return new Comparator() {
public int compare(A arg0, A arg1) {
return function.apply(arg0).compareTo(function.apply(arg1));
}
};
}
/**
* Returns a predicate that evaluates if its argument is less than the given
* value.
*
* More formally, this method returns a new predicate that evaluates
* comparable argument with the statement
* argument.compareTo(value) < 0
*
* @param
* @param value
* @return a new predicate
*/
public static > Predicate lessThan(@NonNull T value) {
return new LessThan(value);
}
/**
* Returns a predicate that evaluates if its argument is lower than or equal
* to the given value.
*
* More formally, this method returns a new predicate that evaluates
* comparable argument with the statement
* argument.compareTo(value) <= 0
*
* @param
* @param value
* @return a new predicate
*/
@IgnoreRestrictions
public static > Predicate lessThanOrEqualTo(@NonNull T value) {
return greaterThan(value).not();
}
/**
* Returns a predicate that evaluates if its argument is greater than the
* given value.
*
* More formally, this method returns a new predicate that evaluates
* comparable argument with the statement
* argument.compareTo(value) > 0
*
* @param
* @param value
* @return a new predicate
*/
public static > Predicate greaterThan(@NonNull T value) {
return new GreaterThan(value);
}
/**
* Returns a predicate that evaluates if its argument is greater than or equal
* to the given value.
*
* More formally, this method returns a new predicate that evaluates
* comparable argument with the statement
* argument.compareTo(value) >= 0
*
* @param
* @param value
* @return a new predicate
*/
@IgnoreRestrictions
public static > Predicate greaterThanOrEqualTo(@NonNull T value) {
return lessThan(value).not();
}
/**
* Answers a natural comparator, that is, a comparator that compares elements
* using its natural order, as defined by {@link Comparable}.
*
* @param
* @return a natural {@link Comparator}
*/
@Constant
public static > Comparator natural() {
return NaturalComparator.natural();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy