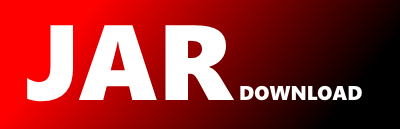
net.sf.staccatocommons.lang.None Maven / Gradle / Ivy
/**
* Copyright (c) 2011, The Staccato-Commons Team
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*/
package net.sf.staccatocommons.lang;
import java.util.Iterator;
import java.util.NoSuchElementException;
import net.sf.staccatocommons.defs.Executable;
import net.sf.staccatocommons.defs.Thunk;
import net.sf.staccatocommons.iterators.thriter.Thriterators;
import net.sf.staccatocommons.restrictions.check.NonNull;
import net.sf.staccatocommons.restrictions.value.Immutable;
import net.sf.staccatocommons.restrictions.value.Value;
/**
* An undefined {@link Option}, that it, and option that does not have a value
*
* @author flbulgarelli
*
* @param
* the type of value - although {@link None} options have no associated
* value at all.
* @see Option
*/
@Value
@Immutable
public final class None extends Option {
private static final long serialVersionUID = 6950027007611799776L;
@SuppressWarnings("unchecked")
private static final None> INSTANCE = new None();
private None() {}
@Override
public T value() {
throw new NoSuchElementException();
}
@Override
public boolean isDefined() {
return false;
}
@Override
public T valueOrElse(T ifUndefined) {
return ifUndefined;
}
@Override
public T valueOrElse(@NonNull Thunk extends T> ifUndefined) {
return ifUndefined.value();
}
@Override
public void ifDefined(Executable super T> block) {
}
// @Override
// public Option bind(Applicable super T, Option> function) {
// return none();
// }
@Override
public T valueOrNull() {
return null;
}
public Iterator iterator() {
return Thriterators.empty();
}
@Override
public int size() {
return 0;
}
@Override
public boolean isEmpty() {
return true;
}
@Override
public boolean contains(Object element) {
return false;
}
public Option skip(T element) {
return this;
}
public int hashCode() {
return 37;
}
public boolean equals(Object obj) {
return obj == this || obj instanceof None;
}
@Override
public String toString() {
return "None";
}
@NonNull
public static None none() {
return (None) INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy