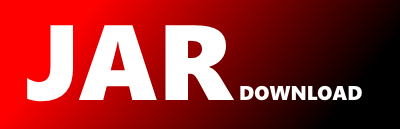
net.sf.staccatocommons.lang.Option Maven / Gradle / Ivy
/**
* Copyright (c) 2011, The Staccato-Commons Team
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*/
package net.sf.staccatocommons.lang;
import java.io.Serializable;
import java.util.Map;
import java.util.NoSuchElementException;
import net.sf.staccatocommons.defs.Applicable;
import net.sf.staccatocommons.defs.Evaluable;
import net.sf.staccatocommons.defs.Executable;
import net.sf.staccatocommons.defs.ProtoMonad;
import net.sf.staccatocommons.defs.Thunk;
import net.sf.staccatocommons.defs.partial.ContainsAware;
import net.sf.staccatocommons.defs.partial.SizeAware;
import net.sf.staccatocommons.restrictions.Conditionally;
import net.sf.staccatocommons.restrictions.Constant;
import net.sf.staccatocommons.restrictions.check.NonNull;
import net.sf.staccatocommons.restrictions.value.Immutable;
import net.sf.staccatocommons.restrictions.value.Value;
/**
*
* {@link Option} represent optional values, that can either be instances of
* {@link Some} or {@link None}.
*
* There are three possible scenarios where {@link Option} type should be used
*
* - When a method may fail without throwing an exception and returns an
* unusable value. Traditionally, this problem is solved using null as centinel
* value. For example, {@link Map#get(Object)}, returns null if there is no
* object mapped for the given key. This kind of code can be tricky, as clients
* may ignore this and continue using the returned value without checking it.
* What is more, such client code can even consider null values valid and
* usable, and then a return value of null has an ambiguous meaning. Using
* {@link Option}s removes ambiguity.
* - When there are uninitialized attributes, but null is already a valid
* initialized value, and should not be treated the same way. Traditionally,
* this is solved with extra boolean variables, but this is error prone. Again,
* use {@link Option} instead, which traits nulls and not-set values not the
* same way
* - When properties may be unset as a valid state, and null is not a valid
* value for the property if set. Client code may forget to check this
* condition, and causing {@link NullPointerException}.
*
*
*
* Option subclasses redefine {@link #equals(Object)} and {@link #hashCode()},
* so that two instances are equal as long they values are equal, or as long
* they are undefined.
*
*
*
* @author flbulgarelli
*
* @param
* the type of optional value
*
*/
@Value
@Conditionally({ Immutable.class, Serializable.class })
public abstract class Option implements Thunk, Iterable, SizeAware, ContainsAware,
ProtoMonad
© 2015 - 2025 Weber Informatics LLC | Privacy Policy