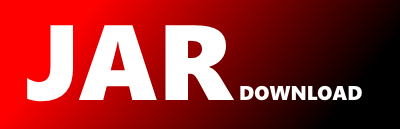
net.sf.staccatocommons.lang.function.AbstractFunction Maven / Gradle / Ivy
/*
Copyright (c) 2011, The Staccato-Commons Team
This program is free software; you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as published by
the Free Software Foundation; version 3 of the License.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
*/
package net.sf.staccatocommons.lang.function;
import net.sf.staccatocommons.defs.Applicable;
import net.sf.staccatocommons.defs.Applicable2;
import net.sf.staccatocommons.defs.Applicable3;
import net.sf.staccatocommons.defs.function.Function;
import net.sf.staccatocommons.defs.function.Function2;
import net.sf.staccatocommons.defs.function.Function3;
import net.sf.staccatocommons.defs.predicate.Predicate;
import net.sf.staccatocommons.lang.predicate.AbstractPredicate;
import net.sf.staccatocommons.restrictions.check.NonNull;
/**
*
* @author flbulgarelli
*
* @param
* @param
*/
public abstract class AbstractFunction extends AbstractDelayable implements Function {
@NonNull
public Function of(@NonNull final Applicable super C, ? extends A> other) {
return new AbstractFunction() {
public B apply(C arg) {
return AbstractFunction.this.apply(other.apply(arg));
}
};
}
@NonNull
public Function2 of(@NonNull final Applicable2 other) {
return new AbstractFunction2() {
public B apply(Tp1 arg0, Tp2 arg1) {
return AbstractFunction.this.apply(other.apply(arg0, arg1));
}
};
}
@NonNull
public Function3 of(@NonNull final Applicable3 other) {
return new AbstractFunction3() {
public B apply(Tp1 arg0, Tp2 arg1, Tp3 arg2) {
return AbstractFunction.this.apply(other.apply(arg0, arg1, arg2));
}
};
}
@NonNull
public Function nullSafe() {
return new AbstractFunction() {
public B apply(A arg) {
if (arg == null)
return null;
return AbstractFunction.this.apply(arg);
}
};
}
public Function then(@NonNull Function super B, ? extends C> other) {
return (Function) other.of(this);
}
public Predicate then(@NonNull Predicate super B> other) {
return other.of(this);
}
/** equivalent to then(Predicates.equal(object)) */
public Predicate equal(final B object) {
return new AbstractPredicate() {
public boolean eval(A argument) {
return AbstractFunction.this.apply(argument).equals(object);
}
};
}
/** equivalent to then(Predicates.same(object)) */
public Predicate same(final B object) {
return new AbstractPredicate() {
public boolean eval(A argument) {
return AbstractFunction.this.apply(argument) == object;
}
};
}
/** equivalent to then(Predicates.notNull()); */
public Predicate notNull() {
return new AbstractPredicate() {
public boolean eval(A argument) {
return AbstractFunction.this.apply(argument) != null;
}
};
}
/** then(Predicates.null_()) */
public Predicate null_() {
return new AbstractPredicate() {
public boolean eval(A argument) {
return AbstractFunction.this.apply(argument) == null;
}
};
}
public Function2 then(final Function2 combinator,
final Function super A2, ? extends B2> other) {
return new AbstractFunction2() {
public C apply(A arg0, A2 arg1) {
return combinator.apply(AbstractFunction.this.apply(arg0), other.apply(arg1));
}
};
}
public String toString() {
return "Function";
}
@Override
public boolean isIdentity() {
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy