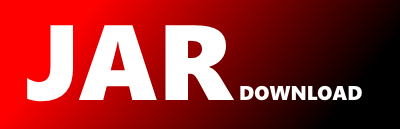
net.sf.staccatocommons.lang.predicate.AbstractPredicate Maven / Gradle / Ivy
/**
* Copyright (c) 2011, The Staccato-Commons Team
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*/
package net.sf.staccatocommons.lang.predicate;
import net.sf.staccatocommons.defs.Applicable;
import net.sf.staccatocommons.defs.Evaluable;
import net.sf.staccatocommons.defs.Executable;
import net.sf.staccatocommons.defs.predicate.Predicate;
import net.sf.staccatocommons.restrictions.check.NonNull;
/**
*
* A {@link AbstractPredicate} is an abstract {@link Evaluable}.
*
*
* Predicates in addition understand the basic boolean logic messages
* {@link #not()}, {@link #and(Evaluable)} and {@link #or(Evaluable)} that
* perform those operations on evaluation result.
*
*
* @author flbulgarelli
*
* @param
* the type of argument to evaluate
*/
public abstract class AbstractPredicate implements Predicate {
@Override
public abstract boolean eval(@NonNull A argument);
public Boolean apply(A arg) {
return eval(arg);
}
/**
* @return a {@link AbstractPredicate} that negates this
* {@link AbstractPredicate}'s result. Non Null.
*/
public Predicate not() {
final class Not extends AbstractPredicate {
public boolean eval(A argument) {
return !AbstractPredicate.this.eval(argument);
}
@Override
public AbstractPredicate not() {
return AbstractPredicate.this;
}
}
return new Not();
}
/**
* Returns a predicate that, performs a short-circuit logical-or between this
* {@link AbstractPredicate}'s {@link #eval(Object)} and other
*
* @param other
* another {@link Evaluable}. Non null.
* @return A new predicate that performs the short circuited or between this
* and other when evaluated. Non Null
*/
public Predicate or(@NonNull final Evaluable super A> other) {
final class Or extends AbstractPredicate {
public boolean eval(A argument) {
return AbstractPredicate.this.eval(argument) || other.eval(argument);
}
}
return new Or();
}
/**
* Returns a predicate that performs a short-circuit logical-and between this
* {@link AbstractPredicate}'s {@link #eval(Object)} and other
*
* @param other
* another {@link Evaluable}. Non null.
* @return A new predicate that performs the short circuited logical-and
* between this and other when evaluated. Non Null
*/
public Predicate and(@NonNull final Evaluable super A> other) {
final class And extends AbstractPredicate {
public boolean eval(A argument) {
return AbstractPredicate.this.eval(argument) && other.eval(argument);
}
}
return new And();
}
public final Predicate andNotNull() {
return Predicates. notNull().and(this);
}
public final Predicate orNull() {
return Predicates. null_().or(this);
}
public Predicate of(@NonNull final Applicable super B, ? extends A> other) {
return new AbstractPredicate() {
public boolean eval(B argument) {
return AbstractPredicate.this.eval(other.apply(argument));
}
};
}
public Predicate withEffectOnFalse(final Executable executable) {
return new AbstractPredicate() {
public boolean eval(A argument) {
boolean result = AbstractPredicate.this.eval(argument);
if (result)
executable.exec(argument);
return result;
}
};
}
public Predicate withEffectOnTrue(final Executable executable) {
return new AbstractPredicate() {
public boolean eval(A argument) {
boolean result = AbstractPredicate.this.eval(argument);
if (!result)
executable.exec(argument);
return result;
}
};
}
// public Predicate withEffect(final Executable executable) {
// return new AbstractPredicate() {
// public boolean eval(A argument) {
// executable.exec(argument);
// return AbstractPredicate.this.eval(argument);
// }
// };
// }
public String toString() {
return "Predicate";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy