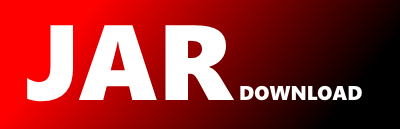
net.sf.staccatocommons.lang.predicate.Predicates Maven / Gradle / Ivy
/**
* Copyright (c) 2011, The Staccato-Commons Team
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*/
package net.sf.staccatocommons.lang.predicate;
import java.util.Arrays;
import java.util.Collection;
import net.sf.staccatocommons.defs.Evaluable;
import net.sf.staccatocommons.defs.NullSafe;
import net.sf.staccatocommons.defs.predicate.Predicate;
import net.sf.staccatocommons.lang.predicate.internal.All;
import net.sf.staccatocommons.lang.predicate.internal.Any;
import net.sf.staccatocommons.lang.predicate.internal.Equals;
import net.sf.staccatocommons.lang.predicate.internal.EvaluablePredicate;
import net.sf.staccatocommons.lang.predicate.internal.False;
import net.sf.staccatocommons.lang.predicate.internal.InPredicate;
import net.sf.staccatocommons.lang.predicate.internal.NullPredicates;
import net.sf.staccatocommons.lang.predicate.internal.Same;
import net.sf.staccatocommons.lang.predicate.internal.True;
import net.sf.staccatocommons.restrictions.Constant;
import net.sf.staccatocommons.restrictions.check.NonNull;
/**
* Factory methods for common predicates
*
* @author flbulgarelli
*/
public class Predicates {
private Predicates() {}
/**
* @param
* @return A {@link AbstractPredicate} that always returns true
*/
@Constant
@NullSafe
public static Predicate true_() {
return True.getInstance();
}
/**
* @param
* @return A {@link AbstractPredicate} that always returns false
*/
@Constant
@NullSafe
public static Predicate false_() {
return False.getInstance();
}
/*
* Object predicates
*/
/**
* Returns a preficate that tests if its argument is not null
*
* @param
* @return A constant {@link AbstractPredicate}
*/
@Constant
@NullSafe
public static Predicate notNull() {
return NullPredicates.notNull();
}
/**
* Returns a predicate that tests if its argument is null
*
* @param
* @return A singleton {@link AbstractPredicate}
*/
@Constant
@NullSafe
public static Predicate null_() {
return NullPredicates.null_();
}
/**
* Returns a predicate that tests if the given value is equal to its argument:
* value.equals(argument)
.
*
* @param
* @param value
* @return Equiv.equal().apply(value)
*/
public static Predicate equal(@NonNull T value) {
return new Equals(value);
}
/**
* Returns a predicate that tests if its argument is the same that the given
* value
*
* @param
* @param value
* @return Equiv.same().apply(value)
*/
@NullSafe
public static Predicate same(T value) {
return new Same(value);
}
/**
* Returns a predicate that tests if its argument is instance of the given
* class
*
* @param
* @param clazz
* @return a new {@link AbstractPredicate}
*/
public static Predicate isInstanceOf(@NonNull final Class extends T> clazz) {
return new AbstractPredicate() {
public boolean eval(T argument) {
return clazz.isAssignableFrom(argument.getClass());
}
};
}
/**
* Returns a predicate that tests if its argument is equal to any of the given
* values
*
* @param
* @param values
* @return a new {@link Predicate}
*/
public static Predicate in(@NonNull T... values) {
return new InPredicate(values);
}
/**
* Returns a predicate that tests if its argument is equal to any of the
* values in the given collection
*
* @param
* @param values
* @return a new {@link AbstractPredicate}
*/
public static Predicate in(@NonNull Collection values) {
return new InPredicate(values);
}
/*
* String predicates
*/
/**
* Returns a predicate that evaluates to true if and only if all the given
* predicates evaluate true
*
* @param
* @param predicates
* @return the all predicate
*/
public static Predicate all(@NonNull Evaluable... predicates) {
return all(Arrays.asList(predicates));
}
/**
* Returns a predicate that evaluates to true if and only if all the given
* predicates evaluate true
*
* @param
* @param predicates
* @return the all predicate
*/
public static Predicate all(@NonNull Iterable> predicates) {
return new All(predicates);
}
/**
* Returns a predicate that evaluates to false if and only if all the given
* predicates evaluate false
*
* @param
* @param predicates
* @return the any predicate
*/
public static Predicate any(@NonNull Evaluable... predicates) {
return any(Arrays.asList(predicates));
}
/**
* Returns a predicate that evaluates to false if and only if all the given
* predicates evaluate false
*
* @param
* @param predicates
* @return the any predicate
*/
public static Predicate any(@NonNull Iterable> predicates) {
return new Any(predicates);
}
/*
* Comparable predicates
*/
/**
* Converts the given {@link Evaluable} into a {@link AbstractPredicate}. If
* it is already a Predicate, returns it.
*
* @param evaluable
* @param
* @return a {@link AbstractPredicate} view of the given evaluable, or the
* evaluable, it is a {@link AbstractPredicate} already
*/
public static Predicate from(@NonNull Evaluable super T> evaluable) {
if (evaluable instanceof AbstractPredicate)
return (Predicate) evaluable;
return new EvaluablePredicate(evaluable);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy