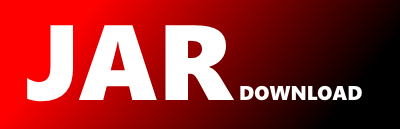
net.sf.staccatocommons.lang.thunk.Thunks Maven / Gradle / Ivy
/**
* Copyright (c) 2011, The Staccato-Commons Team
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*/
package net.sf.staccatocommons.lang.thunk;
import java.util.Date;
import java.util.concurrent.Callable;
import net.sf.staccatocommons.check.Ensure;
import net.sf.staccatocommons.defs.Thunk;
import net.sf.staccatocommons.defs.function.Function;
import net.sf.staccatocommons.iterators.thriter.internal.ConstantThunk;
import net.sf.staccatocommons.lang.SoftException;
import net.sf.staccatocommons.lang.function.AbstractFunction;
import net.sf.staccatocommons.lang.thunk.internal.DateThunk;
import net.sf.staccatocommons.lang.thunk.internal.FailThunk;
import net.sf.staccatocommons.lang.thunk.internal.NullThunk;
import net.sf.staccatocommons.lang.thunk.internal.UndefinedThunk;
import net.sf.staccatocommons.restrictions.Constant;
import net.sf.staccatocommons.restrictions.check.NonNull;
/**
* Class factory methods for some common {@link Thunk}s
*
* @author flbulgarelli
*
*/
public class Thunks {
private Thunks() {}
/**
* Returns a thunk that returns always the given value
*
* @param
* @param value
* the value the constant thunk will return as when invoking
* {@link Thunk#value()}
* @return a new thunk
*/
public static Thunk constant(A value) {
return new ConstantThunk(value);
}
/**
* Returns a constant {@link Thunk} that always provides null
*
* @param
* @return a constant thunk of nulls
*/
@Constant
public static Thunk null_() {
return NullThunk.null_();
}
/**
* Returns a {@link Thunk} that provides the current date
*
* @return a constant thunk that provides new Date()
*/
@Constant
public static Thunk currentDate() {
return DateThunk.INSTANCE;
}
/**
* Returns a thunk whose value is retrieved sending {@link Callable#call()} to
* the given {@link Callable}
*
* @param
* @param callable
* @return a new {@link Thunk} that wraps the given callable
*/
public static Thunk from(@NonNull final Callable callable) {
return new Thunk() {
public A value() {
return SoftException.callOrSoften(callable);
}
};
}
/**
* Returns a thunk that when evaluated throws a {@link RuntimeException}. This
* Thunk is said to have an undefined element.
*
* @param
* @return a {@link Constant} undefined thunk
*/
@Constant
public static Thunk undefined() {
return UndefinedThunk.undefined();
}
/**
* Answers a {@link Thunk} that, when evaluated, throws an exception with the given
* formatted message as {@link Ensure#fail(String, Object...)}
*
* @param message the message
* @param args the message arguments
* @return a new {@link Thunk} that fails with the given message
*/
public static Thunk fail(@NonNull String message, Object... args) {
return new FailThunk(message, args);
}
/**
* Returns a cell that provides not actual value, but a side effect instead,
* by sending {@link Runnable#run()} to the given runnable
*
* @param runnable
* @return a new {@link Thunk} that wraps the given {@link Runnable}
*/
public static Thunk from(@NonNull final Runnable runnable) {
return new Thunk() {
public Void value() {
runnable.run();
return null;
}
};
}
/**
* Returns a {@link Function} that evaluates its Thunk argument
*
* @param
* @return a constant {@link Function}
*/
@Constant
public static Function, A> value() {
return new AbstractFunction, A>() {
public A apply(Thunk arg) {
return arg.value();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy