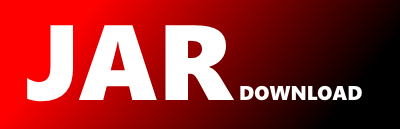
net.sf.staccatocommons.collections.Maps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of staccatissimo-collections Show documentation
Show all versions of staccatissimo-collections Show documentation
Collections library of the Staccatissimo project, focused on providing new abstractions
that mix object oriented and functional programming style for dealing with iterable objects.
The newest version!
/**
* Copyright (c) 2010-2012, The StaccatoCommons Team
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*/
package net.sf.staccatocommons.collections;
import static net.sf.staccatocommons.collections.iterable.Iterables.*;
import java.util.Arrays;
import java.util.Collections;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.NoSuchElementException;
import net.sf.staccatocommons.defs.Applicable;
import net.sf.staccatocommons.defs.tuple.Tuple2;
import net.sf.staccatocommons.lang.MapBuilder;
import net.sf.staccatocommons.lang.None;
import net.sf.staccatocommons.lang.Option;
import net.sf.staccatocommons.restrictions.check.NonNull;
import net.sf.staccatocommons.restrictions.value.Unmodifiable;
/**
* Class methods for dealing with maps
*
* @author flbulgarelli
*/
public class Maps {
/**
* Answers the optional value for the given key
*
* @param
* @param map
* @param key
* the key to lookup
* @return Some(value) if the key exists, None, otherwise
*/
@NonNull
public static Option get(@NonNull Map, V> map, Object key) {
V value = map.get(key);
if (value != null)
return Option.some(value);
if (map.containsKey(key))
return Option.someNull();
return Option.none();
}
/**
* Answers the value for the given key. Throws a
* {@link NoSuchElementException} if there is no mapping for it
*
* @param
* @param map
* @param key
* the key to lookup
* @return Some(value) if the key exists, None, otherwise
*/
@NonNull
public static V getExistent(@NonNull Map, V> map, Object key) {
V value = map.get(key);
if (value != null)
return value;
if (map.containsKey(key))
return null;
throw new NoSuchElementException("No entry found for key " + key);
}
/**
* Answers Some(key) from the given {@link Map}, or {@link None}, if it is
* empty. Notice that any does not mean random, it may return
* always the same key, as this method just guarantees that if Some(key) is
* returned, map.containsKey(key) will return true
*
* @param
* @param map
* @return Some(key) if map is not empty, None otherwise
*/
@NonNull
public static Option anyKey(@NonNull Map map) {
if (map.isEmpty())
return Option.none();
return Option.some(any(map.keySet()));
}
/**
* Answers a key from the given {@link Map}, or null, if it is empty. Notice
* that any does not mean random, it may return always the
* same key, as this method just guarantees that if a non null key is
* returned, map.containsKey(key)
will return true
*
* @param
* @param map
* @return a key if map is not empty, null otherwise
*/
public static K anyKeyOrNull(@NonNull Map map) {
if (map.isEmpty())
return null;
return any(map.keySet());
}
/**
* Answers a Some(value)
from the given {@link Map}, or
* {@link None}, if it is empty. Notice that any does not mean
* random, it may return always the same value, this method just
* guarantees that if a Some(value)
is returned,
* map.containsValue(value)
will return true
*
* @param
* @param map
* @return Some(value) if map is not empty, None otherwise
*/
@NonNull
public static Option anyValue(@NonNull Map, V> map) {
if (map.isEmpty())
return Option.none();
return Option.some(any(map.values()));
}
/**
* Answers a value from the given {@link Map}, or null
, if it is
* empty. Notice that any does not mean random, it may return
* always the same value, as this method just guarantees that if a
* value
is returned, map.containsValue(value)
will
* return true
*
* @param
* @param map
* @return a value
if map is not empty, null
* otherwise
*/
public static V anyValueOrNull(@NonNull Map, V> map) {
if (map.isEmpty())
return null;
return any(map.values());
}
/**
* Answers if the given map is null or empty
*
* @param map
* @return map == null || map.isEmpty()
*/
public static boolean isNullOrEmpty(Map, ?> map) {
return map == null || map.isEmpty();
}
public static Map mapKeys(Map map, Applicable function) {
Map result = new LinkedHashMap();
for (Entry e : map.entrySet())
result.put(e.getKey(), function.apply(e.getValue()));
return Collections.unmodifiableMap(result);
}
public static Map delayedMapKeys(final Map map,
final Applicable super V1, ? extends V2> function) {
return Collections.unmodifiableMap(new LinkedHashMap() {
public V2 get(Object key) {
return function.apply(map.get(key));
}
});
}
/**
* Answers a new {@link Unmodifiable} map with the given entries
*
* @param
* @param
* @param entries
* the new map entries
* @return a new unmodifiable map
*/
public static Map from(Iterable> entries) {
MapBuilder> b = MapBuilder.from(new LinkedHashMap());
for (Tuple2 p : entries)
b.with(p);
return b.build();
}
/**
* Answers a new {@link Unmodifiable} map with the given entries
*
* @param
* @param
* @param entries
* the new map entries
* @return a new unmodifiable map
*/
public static Map from(Tuple2... entries) {
return from(Arrays.asList(entries));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy