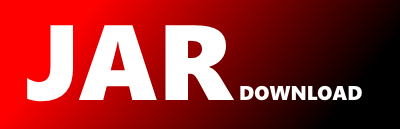
net.sf.staccatocommons.collections.stream.internal.algorithms.MemoizedStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of staccatissimo-collections Show documentation
Show all versions of staccatissimo-collections Show documentation
Collections library of the Staccatissimo project, focused on providing new abstractions
that mix object oriented and functional programming style for dealing with iterable objects.
The newest version!
/**
* Copyright (c) 2010-2012, The StaccatoCommons Team
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*/
package net.sf.staccatocommons.collections.stream.internal.algorithms;
import java.util.NoSuchElementException;
import net.sf.staccatocommons.collections.stream.AbstractStream;
import net.sf.staccatocommons.collections.stream.Stream;
import net.sf.staccatocommons.defs.Thunk;
import net.sf.staccatocommons.iterators.EmptyThriterator;
import net.sf.staccatocommons.iterators.thriter.AdvanceThriterator;
import net.sf.staccatocommons.iterators.thriter.Thriterator;
/**
* @author flbulgarelli
*
*/
public class MemoizedStream extends AbstractStream {
private SingleLinkedDelayedQueue previous = new SingleLinkedDelayedQueue();
private Thriterator remaining;
/**
*
* Creates a new {@link MemoizedStream}
*/
public MemoizedStream(Thriterator source) {
this.remaining = source;
}
@Override
public boolean isEmpty() {
return previous.isEmpty() && !remaining.hasNext();
}
@Override
public Stream memoize() {
return this;
}
@Override
public Thriterator iterator() {
if (remaining.isEmpty()) {
if (previous.isEmpty())
return EmptyThriterator.empty();
return previous.iterator();
}
final Thriterator previousIter = previous.iterator();
return new AdvanceThriterator() {
private Thunk current;
private Thriterator iter = previousIter;
private boolean remainingIterationStarted = false;
public boolean hasNext() {
if (remainingIterationStarted)
return iter.hasNext();
return remaining.hasNext();
}
public void advanceNext() throws NoSuchElementException {
if (!remainingIterationStarted && !iter.hasNext()) {
iter = newRemaningIterator();
remainingIterationStarted = true;
}
iter.advanceNext();
}
public A current() {
return iter.current();
}
@Override
public Thunk delayedCurrent() {
return iter.delayedCurrent();
}
public Thriterator newRemaningIterator() {
return new AdvanceThriterator() {
public boolean hasNext() {
return remaining.hasNext();
}
public void advanceNext() throws NoSuchElementException {
remaining.advanceNext();
current = remaining.delayedCurrent();
previous.add(current);
}
public A current() {
return delayedCurrent().value();
}
@Override
public Thunk delayedCurrent() {
return current;
}
};
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy