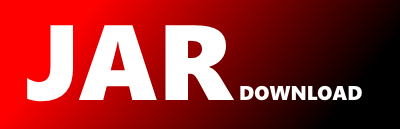
net.sf.staccatocommons.defs.function.Function Maven / Gradle / Ivy
/**
* Copyright (c) 2010-2012, The StaccatoCommons Team
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*/
package net.sf.staccatocommons.defs.function;
import net.sf.staccatocommons.defs.Applicable;
import net.sf.staccatocommons.defs.Applicable2;
import net.sf.staccatocommons.defs.Applicable3;
import net.sf.staccatocommons.defs.Applicative;
import net.sf.staccatocommons.defs.Delayable;
import net.sf.staccatocommons.defs.NullSafe;
import net.sf.staccatocommons.defs.partial.NullSafeAware;
import net.sf.staccatocommons.defs.predicate.Predicate;
import net.sf.staccatocommons.restrictions.check.NonNull;
/**
* {@link Function}s are rich interfaced {@link Applicable}s - one argument
* composable, {@link Delayable} and {@link NullSafeAware} tranformations.
*
* @author flbulgarelli
*
* @param
* function argument type
* @param
* function return type
*/
@Applicative
public interface Function extends Applicable, //
NullSafeAware>, //
Delayable {
/* Composition */
/**
* Composes
* this function with another {@link Applicable}, resulting in a new
* {@link Function} that when applied returns
* this.apply(other.apply(arg)
*
* @param
* @param other
* @return a new function, this
composed with other
*/
Function of(@NonNull final Applicable super C, ? extends A> other);
/**
* Composes
* this function with another {@link Applicable2}, resulting in a new
* {@link Function2} that when applied returns
* this.apply(other.apply(arg0, arg1)
*
* @param
* @param
* @param other
* non null
* @return a new function, this composed with other. Non null.
*/
Function2 of(@NonNull final Applicable2 other);
/**
* Composes
* this function with another {@link Applicable3}, resulting in a new
* {@link Function3} that when applied returns
* this.apply(other.apply(arg0,arg1,arg2)
*
* @param
* @param
* @param
* @param other
* non null
* @return a new function, this composed with other. Non null
*/
Function3 of(@NonNull final Applicable3 other);
/* Then-combination */
/**
* Pipeline combination, equivalent to function composition, like but with
* receptor and argument interchanged.
*
* Functions get combined in the following figure:
*
*
* >----this---+----other---->
*
*
* @param
* @param other
* @return {@code other.of(this)}
*/
Function then(@NonNull Function super B, ? extends C> other);
/**
* Merge combination. Answers a two arg function that combines
* this
function with other
function, using a
* binaryFunction
to merge the results.
*
* The answered {@link Function2} will apply this function to its first
* argument, the other
function to the second argument, and
* return the application of binaryFunction
to both resulting
* values.
*
* Functions get combined in the following figure:
*
*
* >--this-----+
* +---binaryFunction---->
* >--other----+
*
*
* @param
* @param
* @param
* @param binayFunction
* @param other
* @since 1.2
* @return a new {@link Function2} that merges {@code this} and {@code other}
* with {@code binaryFunction}
*/
Function2 then(Function2 binayFunction,
@NonNull Function super A2, ? extends B2> other);
/**
* Predicate composition, like {@link Predicate#of(Applicable)}, but with
* receptor and argument interchanged. Equivalent to {@code other.of(this)}
*
* @param other
* @return a new {@link Predicate}
* @deprecated Use {@link #is(Predicate)} instead
*/
Predicate then(@NonNull Predicate super B> other);
/**
* Predicate composition, like {@link Predicate#of(Applicable)}, but with
* receptor and argument interchanged. Equivalent to {@code other.of(this)}
*
* @param other
* @return a new {@link Predicate}
* @since 2.3
*/
Predicate is(@NonNull Predicate super B> other);
/* Nulls handling */
/**
* Answers a new function that returns null if is argument is null, or the
* result of applying this function, otherwise.
*
* @return a new null-safe {@link Function}
*/
@NullSafe
Function nullSafe();
/* Builtin common compositions */
/**
* Returns a predicate that answers if the result of applying this function is
* equals to the given object.
*
* For example, the following snippet:
*
*
* NumberType<Integer> integerType = ...;
* integerType.add(10).equal(15).apply(5);
*
*
* will be true
, since 5 + 10 = 15
*
* @param other
* @return a new {@link Predicate}
* @deprecated Use {@link #isEqual(B)} instead
*/
Predicate equal(B object);
/**
* Returns a predicate that answers if the result of applying this function is
* equals to the given object.
*
* For example, the following snippet:
*
*
* NumberType<Integer> integerType = ...;
* integerType.add(10).equal(15).apply(5);
*
*
* will be true
, since 5 + 10 = 15
*
* @param other
* @return a new {@link Predicate}
* @since 2.3
*/
Predicate isEqual(B object);
/**
* Returns a predicate that answers if the result of applying this function is
* the same that the given object.
*
* @param other
* @return a new {@link Predicate}
* @deprecated Use {@link #isSame(B)} instead
*/
Predicate same(B object);
/**
* Returns a predicate that answers if the result of applying this function is
* the same that the given object.
*
* @param other
* @return a new {@link Predicate}
* @since 2.3
*/
Predicate isSame(B object);
/**
* Returns a predicate that answers if the result of applying this function is
* null.
*
* @param other
* @return a new {@link Predicate}
* @deprecated Use {@link #isNull()} instead
*/
Predicate null_();
/**
* Returns a predicate that answers if the result of applying this function is
* null.
*
* @param other
* @return a new {@link Predicate}
* @since 2.3
*/
Predicate isNull();
/**
* Returns a predicate that answers if the result of applying this function is
* not null.
*
* @param other
* @return a new {@link Predicate}
* @deprecated Use {@link #isNotNull()} instead
*/
Predicate notNull();
/**
* Returns a predicate that answers if the result of applying this function is
* not null.
*
* @param other
* @return a new {@link Predicate}
* @since 2.3
*/
Predicate isNotNull();
// Function withEffect(Executable effect);
/**
* Answers if this function is the identity function, that is,
* the function that answers the argument it receives
*
* @return if this function is the identity
*/
boolean isIdentity();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy