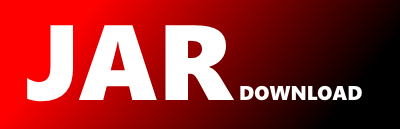
net.sf.staccatocommons.io.internal.Handle Maven / Gradle / Ivy
/**
* Copyright (c) 2010-2012, The StaccatoCommons Team
*
* This program is free software; you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as published by
* the Free Software Foundation; version 3 of the License.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Lesser General Public License for more details.
*/
package net.sf.staccatocommons.io.internal;
import java.util.concurrent.Callable;
import net.sf.staccatocommons.lang.SoftException;
/**
* Utility class for handling exception in {@link Callable}s
*
* @author flbulgarelli
*/
public class Handle {
/**
* Creates a new {@link Handle}
*/
private Handle() {}
/**
* Sends {@link Callable#call()} to the given callable
, throwing
* any exception of type exceptionClass
- including subtypes - ,
* and softening any exception of any other type.
*
*
* @param
* @param exceptionClass
* the type of exception that will be thrown without being soften.
* This class should correspond to a checked
* exception type. Otherwise, this method would not provide any
* benefit over sending call()
directly.
* @throws RuntimeException
* if any exception of type different from
* exceptionClass
is thrown while evaluating
* {@link Callable#call()}.
* @return the result of evaluating {@link Callable#call()}
*/
public static R throwing(Callable thunk, Class exceptionClass)
throws ExceptionType {
try {
return thunk.call();
} catch (Exception e) {
if (shouldCatch(exceptionClass, e)) {
throw (ExceptionType) e;
}
throw SoftException.soften(e);
}
}
/**
* Sends {@link Callable#call()} to the given callable
, throwing
* any exception of type exceptionClass1
or
* exceptionClass2
- including subtypes - , and softening any
* exception of any other type.
*
* @param
* @param
* @param exceptionClass1
* one of the two types of exceptions that will be thrown without
* being soften. This class should correspond to a
* checked exception type. Otherwise, this method would not provide
* any benefit over sending call()
directly.
* @param exceptionClass2
* the second type of exception that will be thrown without being
* soften. Same restrictions of exceptionClass1
apply
* @throws RuntimeException
* if any exception of type different from
* exceptionClass1
or exceptionClass2
is
* thrown while evaluating {@link Callable#call()}.
* @return the result of evaluating {@link Callable#call()}
*/
public static R throwing(Callable thunk,
Class exceptionClass1, Class exceptionClass2) throws ExceptionType1, ExceptionType2 {
try {
return thunk.call();
} catch (Exception e) {
if (shouldCatch(exceptionClass1, e)) {
throw (ExceptionType1) e;
}
if (shouldCatch(exceptionClass2, e)) {
throw (ExceptionType2) e;
}
throw SoftException.soften(e);
}
}
private static boolean shouldCatch(Class exceptionClass, Exception e) {
return exceptionClass.isAssignableFrom(e.getClass());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy