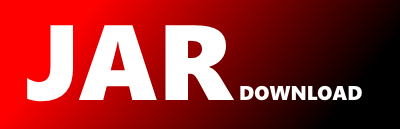
com.ibm.staf.STAFUtil Maven / Gradle / Ivy
/*****************************************************************************/
/* Software Testing Automation Framework (STAF) */
/* (C) Copyright IBM Corp. 2001, 2005 */
/* */
/* This software is licensed under the Eclipse Public License (EPL) V1.0. */
/*****************************************************************************/
package com.ibm.staf;
import com.ibm.staf.service.*;
// STAFUtil - This class provides STAF utility functions
public class STAFUtil
{
public static String privacyDelimiter1 = "!!@";
public static String privacyDelimiter2 = "@!!";
public static String escapedPrivacyDelimiter1 = "^!!@";
public static String escapedPrivacyDelimiter2 = "^@!!";
public static String privacyDelimiterEscape = "^";
// Multiplier to get the value in milliseconds for seconds, minutes, etc.
public static int sMILLISECOND = 1;
public static int sSECOND_IN_MS = 1000;
public static int sMINUTE_IN_MS = 60000;
public static int sHOUR_IN_MS = 3600000;
public static int sDAY_IN_MS = 24 * 3600000;
public static int sWEEK_IN_MS = 7 * 24 * 3600000;
// Made the maximum 4294967294 because we use 4294967295 to indicate
// an indefinite wait on 32-bit machine (in C++). For example:
// STAF_EVENT_SEM_INDEFINITE_WAIT = (unsigned int)-1
// and because the duration value is often passed on to a C++ function
// such as STAFThreadManager::sleepCurrentThread(unsigned int milliseconds)
public static long sMAX_MILLISECONDS = 4294967294L;
public static int sMAX_SECONDS = 4294967;
public static int sMAX_MINUTES = 71582;
public static int sMAX_HOURS = 1193;
public static int sMAX_DAYS = 49;
public static int sMAX_WEEKS = 7;
// Multiplier to get the value in bytes for kilobytes and megabytes
public static int sBYTES = 1;
public static int sBYTES_IN_KILOBYTES = 1024;
public static int sBYTES_IN_MEGABYTES = 1048576;
// Made the maximum 4294967294 because that's the maximum size of
// UINT_MAX on 32-bit machines in C++ and because the size value is often
// passed on to a C++ function
public static long sMAX_BYTES = 4294967294L;
public static int sMAX_KILOBYTES = 4194303;
public static int sMAX_MEGABYTES = 4095;
/***********************************************************************/
/* Description: */
/* This method returns length delimited representation of the string */
/* (aka the colon length colon format). */
/* */
/* Input: A string. For example: "Hi there" */
/* */
/* Returns: */
/* String in the colon lengthh colon format. */
/* For example: ":8:Hi there" */
/***********************************************************************/
public static String wrapData(String data)
{
return ":" + data.length() + ":" + data;
}
/***********************************************************************/
/* Description: */
/* This method returns the string without the colon length colon */
/* prefix. */
/* */
/* Input: String which is in colon length colon format */
/* For example: ":8:Hi there" */
/* */
/* Returns: */
/* String without the colon length colon. For example: "Hi there" */
/***********************************************************************/
public static String unwrapData(String data)
{
if (data != null)
{
int colon1Pos = data.indexOf(":");
if (colon1Pos == 0)
{
int colon2Pos = data.indexOf(":", 1);
if (colon2Pos > -1)
{
try
{
// Verify that an integer was specified between the two
// colons to make sure the value has a colonLengthColon
// format, and just doesn't happen to contain two colons
int length = (new Integer(
data.substring(1, colon2Pos))).intValue();
String newValue = data.substring(colon2Pos + 1);
if (length == newValue.length())
return newValue;
}
catch (NumberFormatException e)
{
// Not a CLC format
}
}
}
}
return data;
}
/***********************************************************************/
/* Description: */
/* This method returns the endpoint without the port (strips @nnnn */
/* from the end of the endpoint */
/* */
/* Input: Endpoint ://[@] */
/* For example: tcp://client1.company.com@6500 */
/* */
/* Returns: */
/* Endpoint without the port. For example: tcp://client1.company.com */
/***********************************************************************/
public static String stripPortFromEndpoint(String endpoint)
{
// Strip the port from the endpoint, if present.
String endpointNoPort = endpoint;
int portIndex = endpoint.lastIndexOf("@");
if (portIndex != -1)
{
// If the characters following the "@" are numeric, then assume
// it's a valid port and strip the @ and the port number from
// the endpoint.
try
{
int port = new Integer(endpoint.substring(portIndex + 1)).
intValue();
endpointNoPort = endpoint.substring(0, portIndex);
}
catch (NumberFormatException e)
{
// Do nothing - Not valid port so don't remove from endpoint
}
}
return endpointNoPort;
}
/***********************************************************************/
/* Description: */
/* This method validates that the requesting machine has the */
/* required trust to submit a service request. */
/* */
/* Input: Required trust level */
/* Service name */
/* Request string (submitted to the service) */
/* Name of the service machine (it's machine identifier) which */
/* is used in the error message if has insufficient trust */
/* Request information */
/* */
/* Returns: */
/* STAFResult.rc = the return code (STAFResult.Ok if successful) */
/* STAFResult.result = blank if successful */
/* a detailed error message if not successful */
/* */
/* Note: Each time a new service interface level is added, must add */
/* another validateTrust method overloaded to support the new */
/* service interface level. */
/***********************************************************************/
public static STAFResult validateTrust(
int requiredTrustLevel, String service, String request,
String localMachine, STAFServiceInterfaceLevel30.RequestInfo info)
{
if (info.trustLevel < requiredTrustLevel)
{
// Strip the port from the machine's endpoint, if present.
String endpoint = stripPortFromEndpoint(info.endpoint);
return new STAFResult(
STAFResult.AccessDenied,
"Trust level " + requiredTrustLevel + " required for the " +
service + " service's " + request + " request\n" +
"Requester has trust level " + info.trustLevel +
" on machine " + localMachine + "\nRequesting machine: " +
endpoint + " (" + info.physicalInterfaceID + ")" +
"\nRequesting user : " + info.user);
}
return new STAFResult(STAFResult.Ok);
}
/***********************************************************************/
/* Description: */
/* This method verifies that the timeout duration is valid and */
/* returns the timeout duration converted to milliseconds. */
/* */
/* Input: String which contains a timeout duration in the format */
/* [s|m|h|d|w] */
/* For example: "100", "1s", "5m", "1h", "1d", "1w" */
/* */
/* Returns: */
/* STAFResult.rc = the return code (STAFResult.Ok if successful) */
/* STAFResult.result = the converted duration in milliseconds */
/* if successful */
/* an error message if not successful */
/***********************************************************************/
public static STAFResult convertDurationString(String durationString)
{
int rc = STAFResult.Ok;
String durationStr = durationString;
long duration = 0;
// Asume duration is specified in milliseconds if numeric
int multiplier = 1;
if ((durationStr == null) || (durationStr.length() == 0))
{
rc = STAFResult.InvalidValue;
}
else
{
// Check if the duration string is not all digits
try
{
duration = (new Long(durationStr)).longValue();
}
catch (NumberFormatException e)
{
// Get duration type (last character of duration string)
String durationType = durationStr.substring(
durationStr.length() - 1).toLowerCase();
if (durationType.equals("s"))
multiplier = sSECOND_IN_MS;
else if (durationType.equals("m"))
multiplier = sMINUTE_IN_MS;
else if (durationType.equals("h"))
multiplier = sHOUR_IN_MS;
else if (durationType.equals("d"))
multiplier = sDAY_IN_MS;
else if (durationType.equals("w"))
multiplier = sWEEK_IN_MS;
else
rc = STAFResult.InvalidValue;
if (rc == STAFResult.Ok)
{
// Assign numeric duration value (all characters
// except the last one)
durationStr = durationString.substring(
0, durationString.length() - 1);
try
{
duration = (new Long(durationStr)).longValue();
}
catch (NumberFormatException e2)
{
rc = STAFResult.InvalidValue;
}
}
}
}
if (rc != STAFResult.Ok)
{
return new STAFResult(
rc,
"This value may be expressed in milliseconds, seconds, " +
"minutes, hours, days, or weeks. Its format is " +
"[s|m|h|d|w] where is an integer >= 0 and " +
"indicates milliseconds unless one of the following " +
"case-insensitive suffixes is specified: s (for seconds), " +
"m (for minutes), h (for hours), d (for days), or " +
"w (for weeks). The calculated value cannot exceed " +
"4294967294 milliseconds.\n\nExamples: \n" +
" 100 specifies 100 milliseconds, \n" +
" 10s specifies 10 seconds, \n" +
" 5m specifies 5 minutes, \n" +
" 2h specifies 2 hours, \n" +
" 1d specifies 1 day, \n" +
" 1w specifies 1 week.");
}
// Because the maximum duration in milliseconds cannot exceed
// 4294967294:
// - Duration specified in seconds cannot exceed 4294967 seconds
// - Duration specified in minutes cannot exceed 71582 minutes
// - Duration specified in hours cannot exceed 1193 hours
// - Duration specified in days cannot exceed 49 days
// - Duration specified in weeks cannot exceed 7 weeks
// Note: The maximum is 4294967294 because we use 4294967295 to
// indicate an indefinite wait on 32-bit machine (in C++).
// For example: STAF_EVENT_SEM_INDEFINITE_WAIT = (unsigned int)-1
// Alsso, because the duration value is often passed on to a C++
// function such as:
// STAFThreadManager::sleepCurrentThread(unsigned int milliseconds)
if (((multiplier == sMILLISECOND) && (duration > sMAX_MILLISECONDS)) ||
((multiplier == sSECOND_IN_MS) && (duration > sMAX_SECONDS)) ||
((multiplier == sMINUTE_IN_MS) && (duration > sMAX_MINUTES)) ||
((multiplier == sHOUR_IN_MS) && (duration > sMAX_HOURS)) ||
((multiplier == sDAY_IN_MS) && (duration > sMAX_DAYS)) ||
((multiplier == sWEEK_IN_MS) && (duration > sMAX_WEEKS)))
{
rc = STAFResult.InvalidValue;
}
if (rc == STAFResult.Ok)
{
duration *= multiplier;
return new STAFResult(
STAFResult.Ok, (new Long(duration)).toString());
}
String errorMsg = "";
if (multiplier == sMILLISECOND)
errorMsg = "Cannot exceed " + sMAX_MILLISECONDS + " milliseconds.";
else if (multiplier == sSECOND_IN_MS)
errorMsg = "Cannot exceed " + sMAX_SECONDS + " seconds.";
else if (multiplier == sMINUTE_IN_MS)
errorMsg = "Cannot exceed " + sMAX_MINUTES + " minutes.";
else if (multiplier == sHOUR_IN_MS)
errorMsg = "Cannot exceed " + sMAX_HOURS + " hours.";
else if (multiplier == sDAY_IN_MS)
errorMsg = "Cannot exceed " + sMAX_DAYS + " days.";
else if (multiplier == sWEEK_IN_MS)
errorMsg = "Cannot exceed " + sMAX_WEEKS + " weeks.";
return new STAFResult(rc, errorMsg);
}
/***********************************************************************/
/* Description: */
/* This method verifies that the size is valid and returns the size */
/* of a file converted to bytes. */
/* */
/* Input: String which contains a size in the format [k|m] */
/* For example: "100000", "500k", "5m" */
/* */
/* Returns: */
/* STAFResult.rc = the return code (STAFResult.Ok if successful) */
/* STAFResult.result = the converted size in bytes if successful */
/* an error message if not successful */
/***********************************************************************/
public static STAFResult convertSizeString(String sizeString)
{
int rc = STAFResult.Ok;
String sizeStr = sizeString;
long size = 0;
// Asume size is specified in bytes if numeric
int multiplier = sBYTES;
if ((sizeStr == null) || (sizeStr.length() == 0))
{
rc = STAFResult.InvalidValue;
}
else
{
// Check if the size string is not all digits
try
{
size = (new Long(sizeStr)).longValue();
}
catch (NumberFormatException e)
{
// Get size type (last character of size string)
String sizeType = sizeStr.substring(
sizeStr.length() - 1).toLowerCase();
if (sizeType.equals("k"))
multiplier = sBYTES_IN_KILOBYTES;
else if (sizeType.equals("m"))
multiplier = sBYTES_IN_MEGABYTES;
else
rc = STAFResult.InvalidValue;
if (rc == STAFResult.Ok)
{
// Assign numeric size value (all characters
// except the last one)
sizeStr = sizeString.substring(
0, sizeString.length() - 1);
try
{
size = (new Long(sizeStr)).longValue();
}
catch (NumberFormatException e2)
{
rc = STAFResult.InvalidValue;
}
}
}
}
if (rc != STAFResult.Ok)
{
return new STAFResult(
rc,
"This value may be expressed in bytes, kilobytes, or " +
"megabytes. Its format is " +
"[k|m] where is an integer >= 0 and " +
"indicates bytes unless one of the following " +
"case-insensitive suffixes is specified: " +
"k (for kilobytes) or m (for megabytes). " +
"The calculated value cannot exceed 4294967294 bytes.\n\n" +
"Examples: \n" +
" 100000 specifies 100,000 bytes, \n" +
" 500k specifies 500 kilobytes (or 512,000 bytes), \n" +
" 5m specifies 5 megabytes (or 5,242,880 bytes), \n" +
" 0 specifies no maximum size limit");
}
// Because the maximum size in bytes cannot exceed 4294967294:
// - Size specified in kilobytes cannot exceed 4294967 seconds
// - Size specified in megabytes cannot exceed 71582 minutes
if (((multiplier == sBYTES) && (size > sMAX_BYTES)) ||
((multiplier == sBYTES_IN_KILOBYTES) && (size > sMAX_KILOBYTES)) ||
((multiplier == sBYTES_IN_MEGABYTES) && (size > sMAX_MEGABYTES)))
{
rc = STAFResult.InvalidValue;
}
if (rc == STAFResult.Ok)
{
size *= multiplier;
return new STAFResult(
STAFResult.Ok, (new Long(size)).toString());
}
String errorMsg = "";
if (multiplier == sBYTES)
errorMsg = "Cannot exceed " + sMAX_BYTES + " bytes.";
else if (multiplier == sBYTES_IN_KILOBYTES)
errorMsg = "Cannot exceed " + sMAX_KILOBYTES + " kilobytes.";
else if (multiplier == sBYTES_IN_MEGABYTES)
errorMsg = "Cannot exceed " + sMAX_MEGABYTES + " megabytes.";
return new STAFResult(rc, errorMsg);
}
/***********************************************************************/
/* Description: */
/* This method resolves any STAF variables that are contained within */
/* the string passed in by submitting a */
/* RESOLVE REQUEST STRING */
/* request to the VAR service on the local system. */
/* The variables will be resolved using the originating handle's pool*/
/* associated with the specified request number, the local system's */
/* shared pool, and the local system's system pool. */
/* */
/* Input: String that may contain STAF variables to be resolved */
/* STAF handle */
/* Request number */
/* */
/* Returns: */
/* STAFResult.rc = the return code (STAFResult.Ok if successful) */
/* STAFResult.result = the resolved value if successful */
/* an error message if not successful */
/***********************************************************************/
public static STAFResult resolveRequestVar(
String value, STAFHandle handle, int requestNumber)
{
if (value.indexOf("{") != -1)
{
// The string may contains STAF variables
STAFResult resolvedResult = handle.submit2(
"local", "VAR", "RESOLVE REQUEST " + requestNumber +
" STRING " + STAFUtil.wrapData(value));
return resolvedResult;
}
return new STAFResult(STAFResult.Ok, value);
}
/***********************************************************************/
/* Description: */
/* This method resolves any STAF variables that are contained within */
/* the string passed in by submitting a */
/* RESOLVE REQUEST STRING */
/* request to the VAR service on the local system. */
/* The variables will be resolved using the originating handle's pool*/
/* associated with the specified request number, the local system's */
/* shared pool, and the local system's system pool. */
/* Then it checks if the resolved value is an integer and returns an */
/* error if it's not an integer. */
/* */
/* Input: Option name whose value is being resolved */
/* Value that may contain STAF variables to be resolved */
/* STAF handle */
/* Request number */
/* */
/* Returns: */
/* STAFResult.rc = the return code (STAFResult.Ok if successful) */
/* STAFResult.result = the resolved option value if successful */
/* an error message if not successful */
/***********************************************************************/
public static STAFResult resolveRequestVarAndCheckInt(
String option, String value, STAFHandle handle, int requestNumber)
{
STAFResult resolvedValue = resolveRequestVar(
value, handle, requestNumber);
if (resolvedValue.rc != STAFResult.Ok) return resolvedValue;
try
{
Integer.parseInt(resolvedValue.result);
}
catch (NumberFormatException e)
{
return new STAFResult(
STAFResult.InvalidValue, option +
" value must be an Integer. " +
option + "=" + resolvedValue.result);
}
return resolvedValue;
}
/***********************************************************************/
/* Description: */
/* This method resolves any STAF variables that are contained within */
/* the string passed in by submitting a */
/* RESOLVE REQUEST STRING */
/* request to the VAR service on the local system. */
/* The variables will be resolved using the originating handle's pool*/
/* associated with the specified request number, the local system's */
/* shared pool, and the local system's system pool. */
/* Then it checks if the resolved value is a valid timeout duration. */
/* If valid, it converts the duration into milliseconds. */
/* If not a valid timeout duration, returns an error. */
/* */
/* Input: Option name whose value is being resolved */
/* Value that may contain STAF variables to be resolved */
/* STAF handle */
/* Request number */
/* */
/* Returns: */
/* STAFResult.rc = the return code (STAFResult.Ok if successful) */
/* STAFResult.result = the resolved and converted timeout duration */
/* in milliseconds if successful, */
/* or an error message if not successful */
/***********************************************************************/
public static STAFResult resolveRequestVarAndConvertDuration(
String option, String value, STAFHandle handle, int requestNumber)
{
STAFResult resolvedValue = resolveRequestVar(
value, handle, requestNumber);
if (resolvedValue.rc != STAFResult.Ok) return resolvedValue;
STAFResult result = convertDurationString(resolvedValue.result);
if (result.rc != STAFResult.Ok)
{
result.result = "Invalid value for the " + option + " option: " +
resolvedValue.result + " \n\n" + result.result;
}
return result;
}
/***********************************************************************/
/* Description: */
/* This method resolves any STAF variables that are contained within */
/* the string passed in by submitting a */
/* RESOLVE REQUEST STRING */
/* request to the VAR service on the local system. */
/* The variables will be resolved using the originating handle's pool*/
/* associated with the specified request number, the local system's */
/* shared pool, and the local system's system pool. */
/* Then it checks if the resolved value is a valid size. */
/* If valid, it converts the size into bytes. */
/* If not a valid size, returns an error. */
/* */
/* Input: Option name whose value is being resolved */
/* Value that may contain STAF variables to be resolved */
/* STAF handle */
/* Request number */
/* */
/* Returns: */
/* STAFResult.rc = the return code (STAFResult.Ok if successful) */
/* STAFResult.result = the resolved and converted size in bytes */
/* if successful, */
/* or an error message if not successful */
/***********************************************************************/
public static STAFResult resolveRequestVarAndConvertSize(
String option, String value, STAFHandle handle, int requestNumber)
{
STAFResult resolvedValue = resolveRequestVar(
value, handle, requestNumber);
if (resolvedValue.rc != STAFResult.Ok) return resolvedValue;
STAFResult result = convertSizeString(resolvedValue.result);
if (result.rc != STAFResult.Ok)
{
result.result = "Invalid value for the " + option + " option: " +
resolvedValue.result + " \n\n" + result.result;
}
return result;
}
/***********************************************************************/
/* Description: */
/* This method resolves any STAF variables that are contained within */
/* the string passed in by submitting a */
/* RESOLVE STRING */
/* request to the VAR service on the local system. */
/* */
/* Input: String that may contain STAF variables to be resolved */
/* STAF handle */
/* */
/* Returns: */
/* STAFResult.rc = the return code (STAFResult.Ok if successful) */
/* STAFResult.result = the resolved string if successful */
/* an error message if not successful */
/***********************************************************************/
public static STAFResult resolveInitVar(String value, STAFHandle handle)
{
if (value.indexOf("{") != -1)
{
// The string may contains STAF variables
STAFResult resolvedResult = handle.submit2(
"local", "VAR", "RESOLVE STRING " + STAFUtil.wrapData(value));
return resolvedResult;
}
return new STAFResult(STAFResult.Ok, value);
}
/***********************************************************************/
/* Description: */
/* This method resolves any STAF variables that are contained within */
/* the string passed in by submitting a */
/* RESOLVE STRING */
/* request to the VAR service on the local system. */
/* Then it checks if the resolved value is an integer and returns an */
/* error if it's not an integer. */
/* */
/* Input: Option name whose value is being resolved */
/* Value for the option that may contain STAF variables */
/* STAF handle */
/* */
/* Returns: */
/* STAFResult.rc = the return code (STAFResult.Ok if successful) */
/* STAFResult.result = the resolved option value if successful */
/* an error message if not successful */
/***********************************************************************/
public static STAFResult resolveInitVarAndCheckInt(
String option, String value, STAFHandle handle)
{
STAFResult resolvedValue = resolveInitVar(value, handle);
if (resolvedValue.rc != STAFResult.Ok) return resolvedValue;
try
{
Integer.parseInt(resolvedValue.result);
}
catch (NumberFormatException e)
{
return new STAFResult(
STAFResult.InvalidValue, option + " value must be numeric. " +
option + "=" + resolvedValue.result);
}
return resolvedValue;
}
/***********************************************************************/
/* Description: */
/* This method gets the version of STAF (or of a STAF service) */
/* running on a specified machine by submitting a VERSION request to */
/* either the MISC service (to get the version of STAF) or to another*/
/* STAF service on the specified machine. Then it checks if the */
/* version meets the minimum required version specified by the */
/* minRequiredVersion argument. If the version is at or above the */
/* required version, STAFResult.Ok is returned in the rc and the */
/* version running on the specified machine is returned in the */
/* result. If the version is lower than the mininum required */
/* version, STAFResult.InvalidSTAFVersion is returned in the rc with */
/* an error message returned in the result. If another error occurs */
/* (e.g. RC 16 if the machine is not currently running STAF, etc.), */
/* that error will be returned. */
/* */
/* Input: 1) machine: endpoint of the machine whose STAF or STAF */
/* service version is to be compared */
/* 2) handle: STAF handle to use to submit the request */
/* 3) minRequiredVersion: The minimum required version. */
/* The version must have the following format unless it's */
/* blank or "", which equates to "no version" and is */
/* internally represented as 0.0.0.0 */
/* */
/* a[.b[.c[.d]]] [text] */
/* */
/* where: */
/* - a, b, c, and d (if specified) are numeric */
/* - text is separated by one or more spaces from the */
/* version numbers */
/* */
/* Versions are compared as follows: */
/* a) The numeric versions (a[.b[.c[.d]]]) are numerically */
/* compared. */
/* b) If the numeric versions are "equal", then the [text] */
/* values are compared using a case-insensitive string */
/* compare. Except, note that no text is considered */
/* GREATER than any text (e.g."3.1.0" > "3.1.0 Beta 1"). */
/* */
/* Examples: */
/* "3" = "3.0" = "3.0.0" = "3.0.0.0" */
/* "3.0.0" < "3.1.0" */
/* "3.0.2" < "3.0.3" */
/* "3.0.0" < "3.1" */
/* "3.0.9" < "3.0.10" */
/* "3.1.0 Beta 1" < "3.1.0" */
/* "3.1.0 Alpha 1" < "3.1.0 Beta 1" */
/* */
/* 4) service: Name of the service for which you want the */
/* version of. Optional. Defaults to "MISC" which means */
/* that you want to compare the version of STAF running on */
/* the machine. Or, you can specify the name of a STAF */
/* service (such as STAX, Event, Cron, etc.) that implements*/
/* a VERSION request and follows the STAF version format */
/* requirements. */
/* */
/* Returns: */
/* STAFResult.rc = the return code (STAFResult.Ok if successful) */
/* STAFResult.result = the resolved option value if successful */
/* an error message if not successful */
/***********************************************************************/
public static STAFResult compareSTAFVersion(
String machine, STAFHandle handle, String minRequiredVersion)
{
// The default is to check the version of STAF running (which is
// done by submitting a VERSION request to the MISC service)
return compareSTAFVersion(
machine, handle, minRequiredVersion, "MISC");
}
public static STAFResult compareSTAFVersion(
String machine, STAFHandle handle, String minRequiredVersion,
String service)
{
STAFResult res = handle.submit2(
machine, service, "VERSION");
if (res.rc != STAFResult.Ok)
{
return new STAFResult(
res.rc,
"Request VERSION submitted to the " + service +
" service on machine " + machine + " failed." +
" Additional info: " + res.result);
}
else
{
STAFVersion version;
STAFVersion minReqVersion;
try
{
version = new STAFVersion(res.result);
}
catch (NumberFormatException e)
{
// Should never happen
return new STAFResult(
STAFResult.InvalidValue,
"Invalid value specified for the version: " +
res.result + ", Exception info: " + e.toString());
}
try
{
minReqVersion = new STAFVersion(minRequiredVersion);
}
catch (NumberFormatException e)
{
return new STAFResult(
STAFResult.InvalidValue,
"Invalid value specified for the minimum required " +
"version: " + minRequiredVersion +
", Exception info: " + e.toString());
}
if (version.compareTo(minReqVersion) < 0)
{
String servMsg = service + " service";
if (service.equalsIgnoreCase("MISC"))
servMsg = "STAF";
return new STAFResult(
STAFResult.InvalidSTAFVersion,
"Machine " + machine + " is running " + servMsg +
" Version " + version + ". Version " +
minRequiredVersion + " or later is required.");
}
else
{
return new STAFResult(STAFResult.Ok, version.toString());
}
}
}
/***********************************************************************/
/* Description: */
/* This method returns the data with a privacy delimiters */
/* */
/* Input: A string. For example: "secret" */
/* */
/* Returns: */
/* String with privacy delimiters added. */
/* For example: "!!@secret@!!" */
/***********************************************************************/
public static String addPrivacyDelimiters(String data)
{
return STAFUtilAddPrivacyDelimiters(data);
}
/***********************************************************************/
/* Description: */
/* This method removes any privacy delimiters from the data */
/* */
/* Input: A string. For example: "!!@secret@!!" */
/* */
/* An integer containing the number of levels of privacy data */
/* to remove (optional). The default is 0 which indicates to */
/* remove all levels of privacy data. */
/* Returns: */
/* String with privacy delimiters removed. */
/* For example: "secret" */
/***********************************************************************/
public static String removePrivacyDelimiters(String data)
{
return STAFUtil.removePrivacyDelimiters(data, 0);
}
public static String removePrivacyDelimiters(String data, int numLevels)
{
return STAFUtilRemovePrivacyDelimiters(data, numLevels);
}
/***********************************************************************/
/* Description: */
/* This method masks any private data indicated by the privacy */
/* delimiters by replacing the private data with asterisks. */
/* */
/* Input: A string. For example: "!!@secret@!!" */
/* */
/* Returns: */
/* String with privacy prefixes removed. */
/* For example: "************" */
/***********************************************************************/
public static String maskPrivateData(String data)
{
return STAFUtilMaskPrivateData(data);
}
/***********************************************************************/
/* Description: */
/* This method escapes all privacy delimiters found in the data and */
/* returns the updated data. */
/* */
/* Input: A string. For example: "!!@secret@!!" */
/* */
/* Returns: */
/* A string with all privacy delimiters escaped. */
/* For example: "^!!@secret^@!!" */
/***********************************************************************/
public static String escapePrivacyDelimiters(String data)
{
return STAFUtilEscapePrivacyDelimiters(data);
}
/************************/
/* All the native stuff */
/************************/
private static native void initialize();
private static native String STAFUtilAddPrivacyDelimiters(String data);
private static native String STAFUtilRemovePrivacyDelimiters(
String data, int numLevels);
private static native String STAFUtilMaskPrivateData(String data);
private static native String STAFUtilEscapePrivacyDelimiters(String data);
// Static initializer - called first time class is loaded.
static
{
if ((System.getProperty("os.name").toLowerCase().indexOf("aix") == 0) ||
(System.getProperty("os.name").toLowerCase().indexOf("linux") == 0) ||
((System.getProperty("os.name").toLowerCase().indexOf("sunos") == 0)
&& (System.getProperty("os.arch").equals("sparc"))))
{
System.loadLibrary("STAF");
}
System.loadLibrary("JSTAF");
initialize();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy