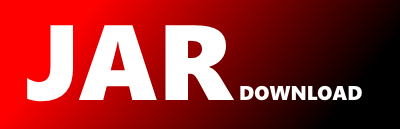
gnu.trove.map.TObjectByteMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
High performance collections for Java
The newest version!
///////////////////////////////////////////////////////////////////////////////
// Copyright (c) 2001, Eric D. Friedman All Rights Reserved.
// Copyright (c) 2009, Rob Eden All Rights Reserved.
// Copyright (c) 2009, Jeff Randall All Rights Reserved.
//
// This library is free software; you can redistribute it and/or
// modify it under the terms of the GNU Lesser General Public
// License as published by the Free Software Foundation; either
// version 2.1 of the License, or (at your option) any later version.
//
// This library is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU Lesser General Public
// License along with this program; if not, write to the Free Software
// Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
///////////////////////////////////////////////////////////////////////////////
package gnu.trove.map;
import gnu.trove.TByteCollection;
import gnu.trove.function.TByteFunction;
import gnu.trove.procedure.TObjectProcedure;
import gnu.trove.procedure.TByteProcedure;
import gnu.trove.procedure.TObjectByteProcedure;
import gnu.trove.iterator.TObjectByteIterator;
import java.util.Map;
import java.util.Set;
//////////////////////////////////////////////////
// THIS IS A GENERATED CLASS. DO NOT HAND EDIT! //
//////////////////////////////////////////////////
/**
* Interface for a primitive map of Object keys and byte values.
*/
public interface TObjectByteMap {
// Query Operations
/**
* Returns the value that will be returned from {@link #get} or {@link #put} if no
* entry exists for a given key. The default value is generally zero, but can be
* configured during construction of the collection.
*
* @return the value that represents a null value in this collection.
*/
byte getNoEntryValue();
/**
* Returns the number of key-value mappings in this map. If the
* map contains more than Integer.MAX_VALUE elements, returns
* Integer.MAX_VALUE.
*
* @return the number of key-value mappings in this map
*/
int size();
/**
* Returns true if this map contains no key-value mappings.
*
* @return true if this map contains no key-value mappings
*/
boolean isEmpty();
/**
* Checks for the present of key in the keys of the map.
*/
boolean containsKey( Object key );
/**
* Checks for the presence of val in the values of the map.
*/
boolean containsValue( byte value );
/**
* Retrieves the value for key.
*
* @return the previous value associated with key, or the "no entry" value
* if none was found (see {@link #getNoEntryValue}).
*/
byte get( Object key );
// Modification Operations
/**
* Inserts a key/value pair into the map.
*
* @return the previous value associated with key, or the "no entry" value
* if none was found (see {@link #getNoEntryValue}).
*/
byte put( K key, byte value);
/**
* Inserts a key/value pair into the map if the specified key is not already
* associated with a value.
*
* @param key key with which the specified value is to be associated
* @param value an byte value to be associated with the specified key
*
* @return the previous value associated with key, or the "no entry" value
* if none was found (see {@link #getNoEntryValue}).
*/
byte putIfAbsent( K key, byte value );
/**
* Deletes a key/value pair from the map.
*
* @param key an #k#
value
*
* @return the previous value associated with key, or the "no entry" value
* if none was found (see {@link #getNoEntryValue}).
*/
byte remove( Object key );
// Bulk Operations
/**
* Copies all of the mappings from the specified map to this map
* (optional operation). The effect of this call is equivalent to that
* of calling {@link #put(Object,byte) put(k, v)} on this map once
* for each mapping from key k to value v in the
* specified map. The behavior of this operation is undefined if the
* specified map is modified while the operation is in progress.
*
* @param m mappings to be stored in this map
* @throws UnsupportedOperationException if the putAll operation
* is not supported by this map
* @throws ClassCastException if the class of a key or value in the
* specified map prevents it from being stored in this map
* @throws NullPointerException if the specified map is null, or if
* this map does not permit null keys or values, and the
* specified map contains null keys or values
* @throws IllegalArgumentException if some property of a key or value in
* the specified map prevents it from being stored in this map
*/
void putAll( Map extends K, ? extends Byte> m);
/**
* Put all the entries from the given map into this map.
*
* @param map The map from which entries will be obtained to put into this map.
*/
void putAll( TObjectByteMap extends K> map );
/**
* Removes all of the mappings from this map (optional operation).
* The map will be empty after this call returns.
*
* @throws UnsupportedOperationException if the clear operation
* is not supported by this map
*/
void clear();
// Views
/**
* Returns a {@link Set} view of the keys contained in this map.
* The set is backed by the map, so changes to the map are
* reflected in the set, and vice-versa. If the map is modified
* while an iteration over the set is in progress (except through
* the iterator's own remove operation), the results of
* the iteration are undefined. The set supports element removal,
* which removes the corresponding mapping from the map, via the
* Iterator.remove, Set.remove,
* removeAll, retainAll, and clear
* operations. It does not support the add or addAll
* operations.
*
* @return a set view of the keys contained in this map
*/
Set keySet();
/**
* Returns a copy of the keys of the map as an array.
* Changes to the array of keys will not be reflected in the map
* nor vice-versa.
*
* @return a copy of the keys of the map as an array.
*/
Object[] keys();
/**
* Returns a copy of the keys of the map as an array.
* Changes to the array of keys will not be reflected in the map
* nor vice-versa.
*
* @param array the array into which the elements of the list are to be stored,
* if it is big enough; otherwise, a new array of the same type is
* allocated for this purpose.
* @return the keys of the map as an array.
*/
K[] keys( K[] array );
/**
* Returns a {@link TByteCollection} view of the values contained in this map.
* The collection is backed by the map, so changes to the map are
* reflected in the collection, and vice-versa. If the map is
* modified while an iteration over the collection is in progress
* (except through the iterator's own remove operation),
* the results of the iteration are undefined. The collection
* supports element removal, which removes the corresponding
* mapping from the map, via the TByteIterator.remove,
* TByteCollection.remove, removeAll,
* retainAll and clear operations. It does not
* support the add or addAll operations.
*
* @return a collection view of the values contained in this map
*/
TByteCollection valueCollection();
/**
* Returns the values of the map as an array of byte values.
* Changes to the array of values will not be reflected in the map
* nor vice-versa.
*
* @return the values of the map as an array of byte values.
*/
byte[] values();
/**
* Returns the values of the map using an existing array.
* Changes to the array of values will not be reflected in the map
* nor vice-versa.
*
* @param array the array into which the elements of the list are to be stored,
* if it is big enough; otherwise, a new array of the same type is
* allocated for this purpose.
* @return the values of the map as an array of byte values.
*/
byte[] values( byte[] array );
/**
* Returns a TObjectByteIterator with access to this map's keys and values.
*
* @return a TObjectByteIterator with access to this map's keys and values.
*/
public TObjectByteIterator iterator();
/**
* Increments the primitive value mapped to key by 1
*
* @param key the key of the value to increment
* @return true if a mapping was found and modified.
*/
public boolean increment( K key );
/**
* Adds an amount to the primitive value mapped to key. If the key does not exist,
* the map is not modified (key-value is not inserted).
*
* @param key the key of the value to increment
* @param amount the amount to add to the value; may be positive, zero, or negative
* @return true if a mapping was found and modified
* @see #adjustOrPutValue(Object, byte, byte)
*/
public boolean adjustValue( K key, byte amount );
/**
* Adds an amount to the primitive value mapped to the key if the key is present in
* the map. Otherwise, the put_amount is put in the map.
*
* @param key the key of the value to increment
* @param adjust_amount the amount to add to the value; may be positive, zero,
* or negative
* @param put_amount the value put into the map if the key is not present
*
* @return the value present in the map after the adjustment or put operation
*/
public byte adjustOrPutValue( K key, byte adjust_amount, byte put_amount );
/**
* Executes procedure for each key in the map.
*
* @param procedure a TObjectProcedure
value
* @return false if the loop over the keys terminated because
* the procedure returned false for some key.
*/
public boolean forEachKey( TObjectProcedure super K> procedure );
/**
* Executes procedure for each value in the map.
*
* @param procedure a TByteProcedure
value
* @return false if the loop over the values terminated because
* the procedure returned false for some value.
*/
public boolean forEachValue( TByteProcedure procedure );
/**
* Executes procedure for each key/value entry in the
* map.
*
* @param procedure a TOObjectByteProcedure
value
* @return false if the loop over the entries terminated because
* the procedure returned false for some entry.
*/
public boolean forEachEntry( TObjectByteProcedure super K> procedure );
/**
* Transform the values in this map using function.
*
* @param function a TByteFunction
value
*/
public void transformValues( TByteFunction function );
/**
* Retains only those entries in the map for which the procedure
* returns a true value.
*
* @param procedure determines which entries to keep
* @return true if the map was modified.
*/
public boolean retainEntries( TObjectByteProcedure super K> procedure );
// Comparison and hashing
/**
* Compares the specified object with this map for equality. Returns
* true if the given object is also a map and the two maps
* represent the same mappings. More formally, two maps m1 and
* m2 represent the same mappings if
* m1.entrySet().equals(m2.entrySet()). This ensures that the
* equals method works properly across different implementations
* of the Map interface.
*
* @param o object to be compared for equality with this map
* @return true if the specified object is equal to this map
*/
boolean equals( Object o );
/**
* Returns the hash code value for this map. The hash code of a map is
* defined to be the sum of the hash codes of each entry in the map's
* entrySet() view. This ensures that m1.equals(m2)
* implies that m1.hashCode()==m2.hashCode() for any two maps
* m1 and m2, as required by the general contract of
* {@link Object#hashCode}.
*
* @return the hash code value for this map
* @see Object#equals(Object)
* @see #equals(Object)
*/
int hashCode();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy