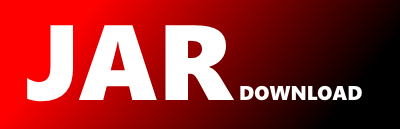
gnu.trove.set.hash.THashSetTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
High performance collections for Java
The newest version!
///////////////////////////////////////////////////////////////////////////////
// Copyright (c) 2001-2006, Eric D. Friedman All Rights Reserved.
// Copyright (c) 2009, Rob Eden All Rights Reserved.
// Copyright (c) 2009, Jeff Randall All Rights Reserved.
//
// This library is free software; you can redistribute it and/or
// modify it under the terms of the GNU Lesser General Public
// License as published by the Free Software Foundation; either
// version 2.1 of the License, or (at your option) any later version.
//
// This library is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
// GNU General Public License for more details.
//
// You should have received a copy of the GNU Lesser General Public
// License along with this program; if not, write to the Free Software
// Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
///////////////////////////////////////////////////////////////////////////////
package gnu.trove.set.hash;
import gnu.trove.impl.PrimeFinder;
import junit.framework.TestCase;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.util.*;
/**
* Created: Sat Nov 3 10:33:15 2001
*
* @author Eric D. Friedman
* @author Rob Eden
* @author Jeff Randall
* @version $Id: THashSetTest.java,v 1.1.2.3 2010/03/02 04:09:50 robeden Exp $
*/
public class THashSetTest extends TestCase {
public THashSetTest( String name ) {
super( name );
}
public void setUp() throws Exception {
super.setUp();
}
public void tearDown() throws Exception {
super.tearDown();
}
public void testConstructors() throws Exception {
Set set = new THashSet();
assertNotNull( set );
String[] strings = {"a", "b", "c", "d"};
set.addAll( Arrays.asList( strings ) );
Set copy = new THashSet( set );
assertTrue( "set not a copy: " + set + ", " + copy, set.equals( copy ) );
Set another = new THashSet( 20 );
another.addAll( Arrays.asList( strings ) );
assertTrue( "set not equal: " + set + ", " + copy, set.equals( another ) );
another = new THashSet( 2, 1.0f );
another.addAll( Arrays.asList( strings ) );
assertTrue( "set not equal: " + set + ", " + copy, set.equals( another ) );
}
// x'd out because we don't usually run tests with > 4gb heaps
public void xxtestLargeCapacity() throws Exception {
THashSet large = new THashSet(Integer.MAX_VALUE);
assertTrue( "capacity was not respected", large.capacity() > 3 );
}
public void xxtestMaxHashSetCapacity() throws Exception {
TIntHashSet full = new TIntHashSet( Integer.MAX_VALUE, 0.5f );
assertEquals( PrimeFinder.nextPrime( Integer.MAX_VALUE ), full.capacity() );
int i;
for (i = 0; full.size() < full.capacity() - 1; i++) {
full.add(i);
}
try {
full.add(++i);
fail( "should have failed to add last key" );
} catch (IllegalStateException ignored) {
}
}
public void testIsEmpty() throws Exception {
Set s = new THashSet();
assertTrue( "new set wasn't empty", s.isEmpty() );
s.add( "One" );
assertTrue( "set with element reports empty", !s.isEmpty() );
s.clear();
assertTrue( "cleared set reports not-empty", s.isEmpty() );
}
public void testContains() throws Exception {
Set s = new THashSet();
String o = "testContains";
s.add( o );
assertTrue( "contains failed", s.contains( o ) );
}
@SuppressWarnings({"ForLoopReplaceableByForEach"})
public void testContainsAll() throws Exception {
Set s = new THashSet();
String[] o = {"Hello World", "Goodbye World", "Hello Goodbye"};
s.addAll( Arrays.asList( o ) );
for ( int i = 0; i < o.length; i++ ) {
assertTrue( o[i], s.contains( o[i] ) );
}
assertTrue( "containsAll failed: " + s,
s.containsAll( Arrays.asList( o ) ) );
String[] more = {"Hello World", "Goodbye World", "Hello Goodbye", "Not There"};
assertFalse( "containsAll failed: " + s,
s.containsAll( Arrays.asList( more ) ) );
}
public void testRetainAll() throws Exception {
Set set = new THashSet();
String[] strings = {"Hello World", "Goodbye World", "Hello Goodbye", "Remove Me"};
set.addAll( Arrays.asList( strings ) );
for ( String string : strings ) {
assertTrue( string, set.contains( string ) );
}
String[] retain = {"Hello World", "Goodbye World", "Hello Goodbye"};
assertTrue( "retainAll failed: " + set,
set.retainAll( Arrays.asList( retain ) ) );
assertTrue( "containsAll failed: " + set,
set.containsAll( Arrays.asList( retain ) ) );
}
public void testRemoveAll() throws Exception {
Set set = new THashSet();
String[] strings = {"Hello World", "Goodbye World", "Hello Goodbye", "Keep Me"};
set.addAll( Arrays.asList( strings ) );
for ( String string : strings ) {
assertTrue( string, set.contains( string ) );
}
String[] remove = {"Hello World", "Goodbye World", "Hello Goodbye"};
assertTrue( "removeAll failed: " + set,
set.removeAll( Arrays.asList( remove ) ) );
assertTrue( "removeAll failed: " + set,
set.containsAll( Arrays.asList( "Keep Me" ) ) );
for ( String element : remove ) {
assertFalse( element + " still in set: " + set, set.contains( element ) );
}
assertEquals( 1, set.size() );
}
public void testAdd() throws Exception {
Set s = new THashSet();
assertTrue( "add failed", s.add( "One" ) );
assertTrue( "duplicated add succeded", !s.add( "One" ) );
}
public void testRemove() throws Exception {
Set s = new THashSet();
s.add( "One" );
s.add( "Two" );
assertTrue( "One was not added", s.contains( "One" ) );
assertTrue( "One was not removed", s.remove( "One" ) );
assertTrue( "One was not removed", !s.contains( "One" ) );
assertTrue( "Two was removed", s.contains( "Two" ) );
assertEquals( 1, s.size() );
}
public void testRemoveObjectNotInSet() throws Exception {
Set set = new THashSet();
set.add( "One" );
set.add( "Two" );
assertTrue( "One was not added", set.contains( "One" ) );
assertTrue( "One was not removed", set.remove( "One" ) );
assertTrue( "One was not removed", !set.contains( "One" ) );
assertTrue( "Two was removed", set.contains( "Two" ) );
assertFalse( "Three was removed (non-existant)", set.remove( "Three" ) );
assertEquals( 1, set.size() );
}
public void testSize() throws Exception {
Set
© 2015 - 2024 Weber Informatics LLC | Privacy Policy